mirror of
https://github.com/sanni/cartreader.git
synced 2024-09-21 07:39:44 +02:00
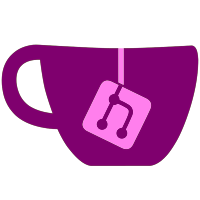
Lots of changes/additions. Added: * Firmware Updater support: Supports the Firmware Updater app (release to follow soon). Enabled by default, can be disabled in the config. * 3.3V Fix (3V3FIX): Enable if you have stability issues when using 3.3V, works best with VSELECT. Disabled by default, can be enabled in the config. * `DynamicClockSerial`: Class that extends and modifies HardwareSerial to be compatible with a dynamically changing clock speed. Used through the `ClockedSerial` object/variable. * `OSCR.cpp` & `OSCR.h`: New files for storing globals. Only contains these new additions for now. More code cleanup to come. Changed: * Moved configuration flags to `Config.h` and documented them better. * Removed `vselect()` function. Now uses `setVoltage()` with the params `VOLTS_SET_3V3` and `VOLTS_SET_5V`. Known Issues: * Rarely the LCD backlight turns white when using 3V3FIX. Resetting fixes it. Doesn't affect functionality/usability; it's just weird.
58 lines
1.8 KiB
C
58 lines
1.8 KiB
C
/********************************************************************
|
|
* Open Source Cartridge Reader *
|
|
********************************************************************/
|
|
#ifndef OSCR_H_
|
|
#define OSCR_H_
|
|
|
|
#include <stdint.h>
|
|
#include <Arduino.h>
|
|
#include <SPI.h>
|
|
#include <Wire.h>
|
|
#include <avr/pgmspace.h>
|
|
#include <avr/wdt.h>
|
|
|
|
#include "Config.h"
|
|
|
|
/*==== SANITY CHECKS ==============================================*/
|
|
#if !(defined(HW1) || defined(HW2) || defined(HW3) || defined(HW4) || defined(HW5) || defined(SERIAL_MONITOR))
|
|
#error !!! PLEASE CHOOSE HARDWARE VERSION !!!
|
|
#endif
|
|
|
|
#if defined(ENABLE_3V3FIX) && !defined(ENABLE_VSELECT)
|
|
#warning Using 3V3FIX is best with VSELECT.
|
|
#endif
|
|
|
|
/*==== CONSTANTS ==================================================*/
|
|
// Updater baud rate
|
|
const uint16_t UPD_BAUD = 9600;
|
|
// Clock speeds
|
|
const unsigned long CS_16MHZ = 16000000UL;
|
|
const unsigned long CS_8MHZ = 8000000UL;
|
|
|
|
// ENUM for VSELECT & 3V3FIX
|
|
enum VOLTS: uint8_t {
|
|
// Paramters to pass to setVoltage() and setClockScale()
|
|
VOLTS_SET_5V = 0, // 5V parameter [ClockScale 0 = 16MHz, Voltage = 5V]
|
|
VOLTS_SET_3V3, // 3.3V parameter [ClockScale 1 = 8MHz, Voltage = 3.3V]
|
|
// Don't use the following as parameters
|
|
// Return values:
|
|
VOLTS_SUCCESS, // Return value for success
|
|
VOLTS_ERROR, // Return value for error
|
|
VOLTS_NOTENABLED, // Return value for not being enabled
|
|
VOLTS_UNKNOWN // Return value for all other states
|
|
};
|
|
|
|
/*==== VARIABLES ==================================================*/
|
|
extern unsigned long clock;
|
|
extern char ver[5];
|
|
extern VOLTS voltage;
|
|
|
|
/*==== FUNCTIONS ==================================================*/
|
|
extern void printVersionToSerial();
|
|
extern void setClockScale(VOLTS __x);
|
|
extern VOLTS setVoltage(VOLTS volts);
|
|
|
|
#include "ClockedSerial.h"
|
|
|
|
#endif /* OSCR_H_ */
|