mirror of
https://github.com/Sude-/lgogdownloader.git
synced 2024-11-20 11:49:17 +01:00
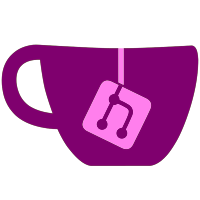
Game details are now acquired using Galaxy API. Allow using most features without valid downloader API login by changing Downloader::isLoggedIn to return false only if website is not logged in. --download-file still uses old API and will not work without valid API login. Downloader::downloadFileWithId prints error message and exits if user doesn't have valid API login. Game details cache version is incremented because of changes to gameFile class. Show product id for DLCs when using --list-details option. Rewrote Downloader::repair to remove duplicated code. Fixed serials containing <br> tags.
50 lines
1.3 KiB
C++
50 lines
1.3 KiB
C++
/* This program is free software. It comes without any warranty, to
|
|
* the extent permitted by applicable law. You can redistribute it
|
|
* and/or modify it under the terms of the Do What The Fuck You Want
|
|
* To Public License, Version 2, as published by Sam Hocevar. See
|
|
* http://www.wtfpl.net/ for more details. */
|
|
|
|
#ifndef GAMEFILE_H
|
|
#define GAMEFILE_H
|
|
|
|
#include "globalconstants.h"
|
|
#include "globals.h"
|
|
|
|
#include <iostream>
|
|
#include <vector>
|
|
#include <json/json.h>
|
|
|
|
// Game file types
|
|
const unsigned int GFTYPE_INSTALLER = 1 << 0;
|
|
const unsigned int GFTYPE_EXTRA = 1 << 1;
|
|
const unsigned int GFTYPE_PATCH = 1 << 2;
|
|
const unsigned int GFTYPE_LANGPACK = 1 << 3;
|
|
const unsigned int GFTYPE_DLC = 1 << 4;
|
|
|
|
class gameFile
|
|
{
|
|
public:
|
|
gameFile();
|
|
int updated;
|
|
std::string gamename;
|
|
std::string id;
|
|
std::string name;
|
|
std::string path;
|
|
std::string size;
|
|
std::string galaxy_downlink_json_url;
|
|
unsigned int platform;
|
|
unsigned int language;
|
|
unsigned int type;
|
|
int score;
|
|
int silent;
|
|
void setFilepath(const std::string& path);
|
|
std::string getFilepath();
|
|
Json::Value getAsJson();
|
|
virtual ~gameFile();
|
|
protected:
|
|
private:
|
|
std::string filepath;
|
|
};
|
|
|
|
#endif // GAMEFILE_H
|