mirror of
https://github.com/Sude-/lgogdownloader.git
synced 2024-11-20 11:49:17 +01:00
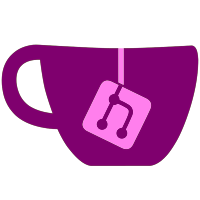
New option "--threads" can be used to set how many parallel downloads to run. The default is 4 threads. Changes to behavior: - Serials and changelogs are saved and covers downloaded for all games that match the "--game" filter before any other files are downloaded. - Automatic XML creation is run after all files are downloaded. Previously xml data was automatically created right after download finished. - The "--limit-rate" option sets rate limit for thread not global rate limit.
85 lines
2.1 KiB
C++
85 lines
2.1 KiB
C++
/* This program is free software. It comes without any warranty, to
|
|
* the extent permitted by applicable law. You can redistribute it
|
|
* and/or modify it under the terms of the Do What The Fuck You Want
|
|
* To Public License, Version 2, as published by Sam Hocevar. See
|
|
* http://www.wtfpl.net/ for more details. */
|
|
|
|
#ifndef THREADSAFEQUEUE_H
|
|
#define THREADSAFEQUEUE_H
|
|
|
|
#include <queue>
|
|
#include <mutex>
|
|
#include <condition_variable>
|
|
|
|
template<typename T>
|
|
class ThreadSafeQueue
|
|
{
|
|
public:
|
|
void push(const T& item)
|
|
{
|
|
std::unique_lock<std::mutex> lock(m);
|
|
q.push(item);
|
|
lock.unlock();
|
|
cvar.notify_one();
|
|
}
|
|
|
|
bool empty() const
|
|
{
|
|
std::unique_lock<std::mutex> lock(m);
|
|
return q.empty();
|
|
}
|
|
|
|
typename std::queue<T>::size_type size() const
|
|
{
|
|
std::unique_lock<std::mutex> lock(m);
|
|
return q.size();
|
|
}
|
|
|
|
bool try_pop(T& item)
|
|
{
|
|
std::unique_lock<std::mutex> lock(m);
|
|
if(q.empty())
|
|
return false;
|
|
|
|
item = q.front();
|
|
q.pop();
|
|
return true;
|
|
}
|
|
|
|
void wait_and_pop(T& item)
|
|
{
|
|
std::unique_lock<std::mutex> lock(m);
|
|
while(q.empty())
|
|
cvar.wait(lock);
|
|
|
|
item = q.front();
|
|
q.pop();
|
|
}
|
|
|
|
ThreadSafeQueue() = default;
|
|
|
|
ThreadSafeQueue(const ThreadSafeQueue& other)
|
|
{
|
|
std::lock_guard<std::mutex> guard(other.m);
|
|
q = other.q;
|
|
}
|
|
|
|
ThreadSafeQueue& operator= (ThreadSafeQueue& other)
|
|
{
|
|
if(&other == this)
|
|
return *this;
|
|
|
|
std::unique_lock<std::mutex> lock1(m, std::defer_lock);
|
|
std::unique_lock<std::mutex> lock2(other.m, std::defer_lock);
|
|
std::lock(lock1, lock2);
|
|
q = other.q;
|
|
return *this;
|
|
}
|
|
private:
|
|
std::queue<T> q;
|
|
mutable std::mutex m;
|
|
std::condition_variable cvar;
|
|
};
|
|
|
|
#endif // THREADSAFEQUEUE_H
|