mirror of
https://github.com/Sude-/lgogdownloader.git
synced 2024-11-20 11:49:17 +01:00
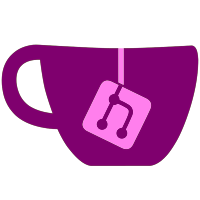
Helps with large libraries when running the downloader multiple times. Getting game details for many games takes a long time. Caching the game details makes the process much faster for subsequent runs. Game details are cached to "$XDG_CACHE_HOME/lgogdownloader/gamedetails.json" --update-cache creates and updates the cache. --use-cache enables loading game details from cache. --cache-valid specifies how long cached game details are considered valid
50 lines
1.0 KiB
C++
50 lines
1.0 KiB
C++
#include "gamefile.h"
|
|
|
|
gameFile::gameFile(const int& t_updated, const std::string& t_id, const std::string& t_name, const std::string& t_path, const std::string& t_size, const unsigned int& t_language, const unsigned int& t_platform, const int& t_silent)
|
|
{
|
|
this->updated = t_updated;
|
|
this->id = t_id;
|
|
this->name = t_name;
|
|
this->path = t_path;
|
|
this->size = t_size;
|
|
this->platform = t_platform;
|
|
this->language = t_language;
|
|
this->silent = t_silent;
|
|
}
|
|
|
|
gameFile::gameFile()
|
|
{
|
|
//ctor
|
|
}
|
|
|
|
gameFile::~gameFile()
|
|
{
|
|
//dtor
|
|
}
|
|
|
|
void gameFile::setFilepath(const std::string& path)
|
|
{
|
|
this->filepath = path;
|
|
}
|
|
|
|
std::string gameFile::getFilepath()
|
|
{
|
|
return this->filepath;
|
|
}
|
|
|
|
Json::Value gameFile::getAsJson()
|
|
{
|
|
Json::Value json;
|
|
|
|
json["updated"] = this->updated;
|
|
json["id"] = this->id;
|
|
json["name"] = this->name;
|
|
json["path"] = this->path;
|
|
json["size"] = this->size;
|
|
json["platform"] = this->platform;
|
|
json["language"] = this->language;
|
|
json["silent"] = this->silent;
|
|
|
|
return json;
|
|
}
|