mirror of
https://github.com/twitter/the-algorithm.git
synced 2025-07-06 03:05:48 +02:00
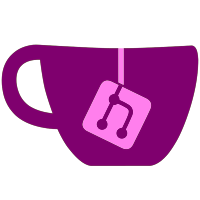
Please note we have force-pushed a new initial commit in order to remove some publicly-available Twitter user information. Note that this process may be required in the future.
46 lines
926 B
Java
46 lines
926 B
Java
package com.twitter.ann.hnsw;
|
|
|
|
import org.apache.commons.lang.builder.EqualsBuilder;
|
|
import org.apache.commons.lang.builder.HashCodeBuilder;
|
|
|
|
public class HnswNode<T> {
|
|
public final int level;
|
|
public final T item;
|
|
|
|
public HnswNode(int level, T item) {
|
|
this.level = level;
|
|
this.item = item;
|
|
}
|
|
|
|
/**
|
|
* Create a hnsw node.
|
|
*/
|
|
public static <T> HnswNode<T> from(int level, T item) {
|
|
return new HnswNode<>(level, item);
|
|
}
|
|
|
|
@Override
|
|
public boolean equals(Object o) {
|
|
if (o == this) {
|
|
return true;
|
|
}
|
|
if (!(o instanceof HnswNode)) {
|
|
return false;
|
|
}
|
|
|
|
HnswNode<?> that = (HnswNode<?>) o;
|
|
return new EqualsBuilder()
|
|
.append(this.item, that.item)
|
|
.append(this.level, that.level)
|
|
.isEquals();
|
|
}
|
|
|
|
@Override
|
|
public int hashCode() {
|
|
return new HashCodeBuilder()
|
|
.append(item)
|
|
.append(level)
|
|
.toHashCode();
|
|
}
|
|
}
|