mirror of
https://github.com/Lime3DS/Lime3DS.git
synced 2024-11-14 05:55:13 +01:00
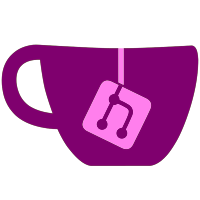
* Change authentication system to JWT * Address review comments * Get rid of global variable, fix some documentations, fix a bug when verificating * Refactor PostJson to avoid code duplication * Rename jwt_token, add functionality to request a new JWT when getting a 401 * Take bools by value instead of const reference * Send request again when JWT is invalid and use forward declarations * Omit brackets
34 lines
943 B
C++
34 lines
943 B
C++
// Copyright 2017 Citra Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#include "web_service/json.h"
|
|
#include "web_service/verify_login.h"
|
|
#include "web_service/web_backend.h"
|
|
|
|
namespace WebService {
|
|
|
|
std::future<bool> VerifyLogin(std::string& username, std::string& token,
|
|
const std::string& endpoint_url, std::function<void()> func) {
|
|
auto get_func = [func, username](const std::string& reply) -> bool {
|
|
func();
|
|
|
|
if (reply.empty()) {
|
|
return false;
|
|
}
|
|
|
|
nlohmann::json json = nlohmann::json::parse(reply);
|
|
const auto iter = json.find("username");
|
|
|
|
if (iter == json.end()) {
|
|
return username.empty();
|
|
}
|
|
|
|
return username == *iter;
|
|
};
|
|
UpdateCoreJWT(true, username, token);
|
|
return GetJson<bool>(get_func, endpoint_url, false);
|
|
}
|
|
|
|
} // namespace WebService
|