mirror of
https://github.com/Ryujinx/ChocolArm64.git
synced 2024-06-02 17:28:52 +02:00
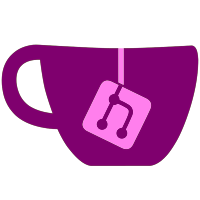
* Change naming convention for Ryujinx project * Change naming convention for ChocolArm64 project * Fix NaN * Remove unneeded this. from Ryujinx project * Adjust naming from new PRs * Name changes based on feedback * How did this get removed? * Rebasing fix * Change FP enum case * Remove prefix from ChocolArm64 classes - Part 1 * Remove prefix from ChocolArm64 classes - Part 2 * Fix alignment from last commit's renaming * Rename namespaces * Rename stragglers * Fix alignment * Rename OpCode class * Missed a few * Adjust alignment
70 lines
1.8 KiB
C#
70 lines
1.8 KiB
C#
using ChocolArm64.Decoders;
|
|
using ChocolArm64.Decoders32;
|
|
using ChocolArm64.Memory;
|
|
using ChocolArm64.State;
|
|
|
|
using static ChocolArm64.Instructions32.A32InstInterpretHelper;
|
|
|
|
namespace ChocolArm64.Instructions32
|
|
{
|
|
static partial class A32InstInterpret
|
|
{
|
|
public static void B(CpuThreadState state, MemoryManager memory, OpCode64 opCode)
|
|
{
|
|
A32OpCodeBImmAl op = (A32OpCodeBImmAl)opCode;
|
|
|
|
if (IsConditionTrue(state, op.Cond))
|
|
{
|
|
BranchWritePc(state, GetPc(state) + (uint)op.Imm);
|
|
}
|
|
}
|
|
|
|
public static void Bl(CpuThreadState state, MemoryManager memory, OpCode64 opCode)
|
|
{
|
|
Blx(state, memory, opCode, false);
|
|
}
|
|
|
|
public static void Blx(CpuThreadState state, MemoryManager memory, OpCode64 opCode)
|
|
{
|
|
Blx(state, memory, opCode, true);
|
|
}
|
|
|
|
public static void Blx(CpuThreadState state, MemoryManager memory, OpCode64 opCode, bool x)
|
|
{
|
|
A32OpCodeBImmAl op = (A32OpCodeBImmAl)opCode;
|
|
|
|
if (IsConditionTrue(state, op.Cond))
|
|
{
|
|
uint pc = GetPc(state);
|
|
|
|
if (state.Thumb)
|
|
{
|
|
state.R14 = pc | 1;
|
|
}
|
|
else
|
|
{
|
|
state.R14 = pc - 4U;
|
|
}
|
|
|
|
if (x)
|
|
{
|
|
state.Thumb = !state.Thumb;
|
|
}
|
|
|
|
if (!state.Thumb)
|
|
{
|
|
pc &= ~3U;
|
|
}
|
|
|
|
BranchWritePc(state, pc + (uint)op.Imm);
|
|
}
|
|
}
|
|
|
|
private static void BranchWritePc(CpuThreadState state, uint pc)
|
|
{
|
|
state.R15 = state.Thumb
|
|
? pc & ~1U
|
|
: pc & ~3U;
|
|
}
|
|
}
|
|
} |