mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-09-16 11:34:47 +02:00
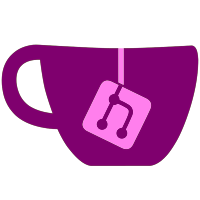
* generator/CodeGenerator.cs (Main): use new ObjectGen.GenerateMappers. * generator/GenerationInfo.cs (Ctor): new (dir, assembly) ctor * generator/ObjectGen.cs : move hash management to Generate from Ctor, index it on dir, and make it hold new DirectoryInfo refs. Refactor GenerateMapper. The object mappers are now assembly based instead of namespace based. svn path=/trunk/gtk-sharp/; revision=18681
83 lines
1.7 KiB
C#
83 lines
1.7 KiB
C#
// GtkSharp.Generation.GenerationInfo.cs - Generation information class.
|
|
//
|
|
// Author: Mike Kestner <mkestner@ximian.com>
|
|
//
|
|
// (c) 2003 Ximian Inc.
|
|
|
|
namespace GtkSharp.Generation {
|
|
|
|
using System;
|
|
using System.Collections;
|
|
using System.IO;
|
|
using System.Xml;
|
|
|
|
public class GenerationInfo {
|
|
|
|
string dir;
|
|
string custom_dir;
|
|
string assembly_name;
|
|
StreamWriter sw;
|
|
|
|
public GenerationInfo (XmlElement ns)
|
|
{
|
|
string ns_name = ns.GetAttribute ("name");
|
|
char sep = Path.DirectorySeparatorChar;
|
|
dir = ".." + sep + ns_name.ToLower () + sep + "generated";
|
|
custom_dir = ".." + sep + ns_name.ToLower ();
|
|
assembly_name = ns_name.ToLower () + "-sharp";
|
|
}
|
|
|
|
public GenerationInfo (string dir, string assembly_name)
|
|
{
|
|
this.dir = dir;
|
|
custom_dir = dir;
|
|
this.assembly_name = assembly_name;
|
|
}
|
|
|
|
public string AssemblyName {
|
|
get {
|
|
return assembly_name;
|
|
}
|
|
}
|
|
|
|
public string CustomDir {
|
|
get {
|
|
return custom_dir;
|
|
}
|
|
}
|
|
|
|
public string Dir {
|
|
get {
|
|
return dir;
|
|
}
|
|
}
|
|
|
|
public StreamWriter Writer {
|
|
get {
|
|
return sw;
|
|
}
|
|
set {
|
|
sw = value;
|
|
}
|
|
}
|
|
|
|
public StreamWriter OpenStream (string name)
|
|
{
|
|
char sep = Path.DirectorySeparatorChar;
|
|
if (!Directory.Exists(dir))
|
|
Directory.CreateDirectory(dir);
|
|
string filename = dir + sep + name + ".cs";
|
|
|
|
FileStream stream = new FileStream (filename, FileMode.Create, FileAccess.Write);
|
|
StreamWriter sw = new StreamWriter (stream);
|
|
|
|
sw.WriteLine ("// This file was generated by the Gtk# code generator.");
|
|
sw.WriteLine ("// Any changes made will be lost if regenerated.");
|
|
sw.WriteLine ();
|
|
|
|
return sw;
|
|
}
|
|
}
|
|
}
|
|
|