mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-09-08 16:03:21 +02:00
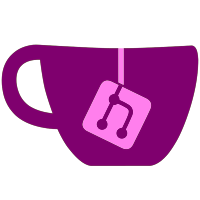
GInterfaceInfo.Data was automatically set to be a GCHandle on the interface adapter. But the generated GInterfaceInitHandlers were not using it, just free'ing it. But for the GInterface property support, the Data field is now used to pass the class pointer, so casting it to a GCHandle to free it would cause an exception. We now don't assume anything about GInterfaceInfo.Data.
62 lines
1.6 KiB
C#
62 lines
1.6 KiB
C#
// GInterfaceAdapter.cs
|
|
//
|
|
// Author: Mike Kestner <mkestner@novell.com>
|
|
//
|
|
// Copyright (c) 2007 Novell, Inc.
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of version 2 of the Lesser GNU General
|
|
// Public License as published by the Free Software Foundation.
|
|
//
|
|
// This program is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public
|
|
// License along with this program; if not, write to the
|
|
// Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
// Boston, MA 02111-1307, USA.
|
|
|
|
|
|
namespace GLib {
|
|
|
|
using System;
|
|
using System.Runtime.InteropServices;
|
|
|
|
[UnmanagedFunctionPointer (CallingConvention.Cdecl)]
|
|
public delegate void GInterfaceInitHandler (IntPtr iface_ptr, IntPtr data);
|
|
|
|
[UnmanagedFunctionPointer (CallingConvention.Cdecl)]
|
|
internal delegate void GInterfaceFinalizeHandler (IntPtr iface_ptr, IntPtr data);
|
|
|
|
internal struct GInterfaceInfo {
|
|
internal GInterfaceInitHandler InitHandler;
|
|
internal GInterfaceFinalizeHandler FinalizeHandler;
|
|
internal IntPtr Data;
|
|
}
|
|
|
|
public abstract class GInterfaceAdapter {
|
|
|
|
GInterfaceInfo info;
|
|
|
|
protected GInterfaceAdapter ()
|
|
{
|
|
}
|
|
|
|
protected GInterfaceInitHandler InitHandler {
|
|
set {
|
|
info.InitHandler = value;
|
|
}
|
|
}
|
|
|
|
public abstract GType GType { get; }
|
|
|
|
public abstract IntPtr Handle { get; }
|
|
|
|
internal GInterfaceInfo Info {
|
|
get { return info; }
|
|
}
|
|
}
|
|
}
|