mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-11 14:41:43 +02:00
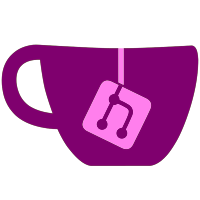
* generator/ClassBase.cs: New base class for classes and interfaces. * generator/InterfaceGen.cs: Inherit from ClassBase, generate declarations. * generator/ObjectGen.cs: Move half of this into ClassBase. * generator/Method.cs: Turn all applicable Get/Set functions into .NET accessors. Remove redundant == overload and move into Equals, as it was confusing "!= null". * generator/Parameters.cs: Alter signature creation to accept "is_set" option, add support for variable arguments. Add properties "Count", "IsVarArgs", "VAType". * generator/Ctor.cs: Fixup for changes in Parameters (indenting, signature creation). * generator/Signal.cs: Support generating declarations. * generator/SymbolTable: Change GetObjectGen to GetClassGen. * glib/IWrapper.cs: Move "Handle" declaration to here, so both classes and interfaces can benefit from it. * glib/Object.cs: Inherit from IWrapper.cs * parser/Metadata.pm: Support attribute changes on constructors, methods, signals, and paramater lists. * parser/gapi2xml.pl: Parse init funcs for interfaces. Ignore "_" functions here. * parser/gapi_pp.pl: Remove boxed_type_register check, as it will be caught in the init funcs. * parser/Atk.metadata: Added. * parser/Gtk.metadata: Add all needed signal/method collision renames. Rename GtkEditable.Editable accessors to IsEditable, as .NET does not like accessors with the same name as their declaring type. Tag TreeStore constructor as varargs. * samples/ButtonApp.cs: s/EmitAdd/Add. * samples/Menu.cs: s/EmitAdd/Add, s/Activate/Activated. svn path=/trunk/gtk-sharp/; revision=5394
88 lines
2.2 KiB
Perl
Executable File
88 lines
2.2 KiB
Perl
Executable File
#!/usr/bin/perl
|
|
#
|
|
# gapi_pp.pl : A source preprocessor for the extraction of API info from a
|
|
# C library source directory.
|
|
#
|
|
# Author: Mike Kestner <mkestner@speakeasy.net>
|
|
#
|
|
# <c> 2001 Mike Kestner
|
|
|
|
$eatit_regex = "^#if.*(__cplusplus|DEBUG|DISABLE_(DEPRECATED|COMPAT)|ENABLE_BROKEN|COMPILATION)";
|
|
$ignoreit_regex = '^\s+\*|#\s*include|#\s*else|#\s*endif|#\s*undef|G_(BEGIN|END)_DECLS|extern|GDKVAR|GTKVAR|GTKMAIN_C_VAR|GTKTYPEUTILS_VAR|VARIABLE|GTKTYPEBUILTIN';
|
|
|
|
foreach $dir (@ARGV) {
|
|
@hdrs = (@hdrs, `ls $dir/*.h`);
|
|
}
|
|
|
|
foreach $fname (@hdrs) {
|
|
|
|
if ($fname =~ /test|private|internals|gtktextlayout|gtkmarshalers/) {
|
|
@privhdrs = (@privhdrs, $fname);
|
|
next;
|
|
}
|
|
|
|
open(INFILE, $fname) || die "Could open $fname\n";
|
|
|
|
while ($line = <INFILE>) {
|
|
|
|
next if ($line =~ /$ignoreit_regex/);
|
|
next if ($line !~ /\S/);
|
|
|
|
if ($line =~ /#\s*define\s+\w+\s*\D+/) {
|
|
$def = $line;
|
|
while ($line =~ /\\\n/) {$def .= ($line = <INFILE>);}
|
|
if ($def =~ /_CHECK_\w*CAST|INSTANCE_GET_INTERFACE/) {
|
|
$def =~ s/\\\n//g;
|
|
print $def;
|
|
}
|
|
} elsif ($line =~ /^\s*\/\*/) {
|
|
while ($line !~ /\*\//) {$line = <INFILE>;}
|
|
} elsif ($line =~ /^#ifndef\s+\w+_H_*\b/) {
|
|
while ($line !~ /#define/) {$line = <INFILE>;}
|
|
} elsif ($line =~ /$eatit_regex/) {
|
|
while ($line !~ /#else|#endif/) {$line = <INFILE>;}
|
|
} elsif ($line =~ /^#\s*ifn?\s*\!?def/) {
|
|
#warn "Ignored #if:\n$line";
|
|
} elsif ($line =~ /typedef\s+struct\s+\w*\s*\{/) {
|
|
while ($line !~ /^}\s*\w+;/) {$line = <INFILE>;}
|
|
} elsif ($line =~ /^enum\s+\{/) {
|
|
while ($line !~ /^};/) {$line = <INFILE>;}
|
|
} else {
|
|
print $line;
|
|
}
|
|
}
|
|
}
|
|
|
|
foreach $fname (`ls $ARGV[0]/*.c`, @privhdrs) {
|
|
|
|
open(INFILE, $fname) || die "Could open $fname\n";
|
|
|
|
if ($fname =~ /builtins_ids/) {
|
|
while ($line = <INFILE>) {
|
|
next if ($line !~ /\{/);
|
|
|
|
chomp($line);
|
|
$builtin = "BUILTIN" . $line;
|
|
$builtin .= <INFILE>;
|
|
print $builtin;
|
|
}
|
|
next;
|
|
}
|
|
|
|
while ($line = <INFILE>) {
|
|
#next if ($line !~ /^(struct|\w+_class_init)|g_boxed_type_register_static/);
|
|
next if ($line !~ /^(struct|\w+_class_init|\w+_base_init|\w+_get_type)/);
|
|
|
|
if ($line =~ /^struct/) {
|
|
# need some of these to parse out parent types
|
|
print "private";
|
|
}
|
|
|
|
do {
|
|
print $line;
|
|
} until (($line = <INFILE>) =~ /^}/);
|
|
print $line;
|
|
}
|
|
}
|
|
|