mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-11 14:41:43 +02:00
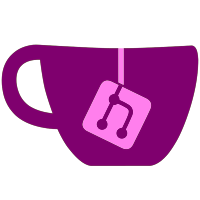
* gdk/Pixbuf.custom: Changed Pixbuf.Pixels to return an IntPtr instead of a byte * -- anyone who needs a byte * can do the cast in an unsafe context already. svn path=/trunk/gtk-sharp/; revision=27617
170 lines
5.9 KiB
Plaintext
170 lines
5.9 KiB
Plaintext
// Pixbuf.custom - Gdk Pixbuf class customizations
|
|
//
|
|
// Authors:
|
|
// Vladimir Vukicevic <vladimir@pobox.com>
|
|
// Miguel de Icaza <miguel@ximian.com>
|
|
// Mike Kestner <mkestner@ximian.com>
|
|
// Duncan Mak <duncan@ximian.com>
|
|
// Gonzalo Paniagua Javier <gonzalo@ximian.com>
|
|
// Martin Willemoes Hansen <mwh@sysrq.dk>
|
|
//
|
|
// (C) 2002 Vladimir Vukicevic
|
|
// (C) 2003 Ximian, Inc. (Miguel de Icaza)
|
|
// (C) 2003 Ximian, Inc. (Duncan Mak)
|
|
// (C) 2003 Ximian, Inc. (Gonzalo Paniagua Javier)
|
|
// (C) 2003 Martin Willemoes Hansen
|
|
// (C) 2004 Novell, Inc.
|
|
//
|
|
// This code is inserted after the automatically generated code.
|
|
|
|
[DllImport("libgobject-2.0-0.dll")]
|
|
static extern void g_object_ref (IntPtr handle);
|
|
|
|
private void LoadFromStream (System.IO.Stream input)
|
|
{
|
|
PixbufLoader loader = new PixbufLoader ();
|
|
byte [] buffer = new byte [8192];
|
|
int n;
|
|
|
|
while ((n = input.Read (buffer, 0, 8192)) != 0)
|
|
loader.Write (buffer, (uint) n);
|
|
|
|
loader.Close ();
|
|
Raw = loader.PixbufHandle;
|
|
if (Raw == IntPtr.Zero)
|
|
throw new ArgumentException ("Unable to load pixbuf from stream");
|
|
g_object_ref (Raw);
|
|
}
|
|
|
|
public Pixbuf (System.IO.Stream stream) : base (IntPtr.Zero)
|
|
{
|
|
LoadFromStream (stream);
|
|
}
|
|
|
|
public Pixbuf (System.Reflection.Assembly assembly, string resource) : base (IntPtr.Zero)
|
|
{
|
|
if (assembly == null)
|
|
assembly = System.Reflection.Assembly.GetCallingAssembly ();
|
|
|
|
if (resource == null)
|
|
throw new ArgumentNullException ("resource");
|
|
|
|
System.IO.Stream s = assembly.GetManifestResourceStream (resource);
|
|
if (s == null)
|
|
throw new ArgumentException ("resource must be a valid resource name of 'assembly'.");
|
|
|
|
LoadFromStream (s);
|
|
}
|
|
|
|
static public Pixbuf LoadFromResource (string resource)
|
|
{
|
|
if (resource == null)
|
|
throw new ArgumentNullException ("resource");
|
|
|
|
return new Pixbuf (System.Reflection.Assembly.GetCallingAssembly (), resource);
|
|
}
|
|
|
|
|
|
[DllImport("libgdk_pixbuf-2.0-0.dll")]
|
|
static extern IntPtr gdk_pixbuf_scale_simple(IntPtr raw, int dest_width, int dest_height, int interp_type);
|
|
|
|
public Gdk.Pixbuf ScaleSimple(int dest_width, int dest_height, Gdk.InterpType interp_type) {
|
|
IntPtr raw_ret = gdk_pixbuf_scale_simple(Handle, dest_width, dest_height, (int) interp_type);
|
|
Gdk.Pixbuf ret = (Gdk.Pixbuf) GLib.Object.GetObject(raw_ret, true);
|
|
return ret;
|
|
}
|
|
|
|
[DllImport("libgdk_pixbuf-2.0-0.dll")]
|
|
static extern IntPtr gdk_pixbuf_composite_color_simple(IntPtr raw, int dest_width, int dest_height, int interp_type, int overall_alpha, int check_size, uint color1, uint color2);
|
|
|
|
public Gdk.Pixbuf CompositeColorSimple(int dest_width, int dest_height, Gdk.InterpType interp_type, int overall_alpha, int check_size, uint color1, uint color2) {
|
|
IntPtr raw_ret = gdk_pixbuf_composite_color_simple(Handle, dest_width, dest_height, (int) interp_type, overall_alpha, check_size, color1, color2);
|
|
Gdk.Pixbuf ret = (Gdk.Pixbuf) GLib.Object.GetObject(raw_ret, true);
|
|
return ret;
|
|
}
|
|
|
|
[DllImport("libgdk_pixbuf-2.0-0.dll")]
|
|
static extern IntPtr gdk_pixbuf_add_alpha(IntPtr raw, bool substitute_color, byte r, byte g, byte b);
|
|
|
|
public Gdk.Pixbuf AddAlpha(bool substitute_color, byte r, byte g, byte b) {
|
|
IntPtr raw_ret = gdk_pixbuf_add_alpha(Handle, substitute_color, r, g, b);
|
|
Gdk.Pixbuf ret = (Gdk.Pixbuf) GLib.Object.GetObject(raw_ret, true);
|
|
return ret;
|
|
}
|
|
|
|
// overload to default the colorspace
|
|
public Pixbuf(byte [] data, bool has_alpha, int bits_per_sample, int width, int height, int rowstride, Gdk.PixbufDestroyNotify destroy_fn) : base (IntPtr.Zero)
|
|
{
|
|
GdkSharp.PixbufDestroyNotifyWrapper destroy_fn_wrapper = null;
|
|
destroy_fn_wrapper = new GdkSharp.PixbufDestroyNotifyWrapper (destroy_fn, this);
|
|
Raw = gdk_pixbuf_new_from_data(data, (int) Gdk.Colorspace.Rgb, has_alpha, bits_per_sample, width, height, rowstride, destroy_fn_wrapper.NativeDelegate, IntPtr.Zero);
|
|
}
|
|
|
|
public unsafe Pixbuf(byte[] data, bool copy_pixels) : base (IntPtr.Zero)
|
|
{
|
|
IntPtr error = IntPtr.Zero;
|
|
Raw = gdk_pixbuf_new_from_inline(data.Length, data, copy_pixels, out error);
|
|
if (error != IntPtr.Zero) throw new GLib.GException (error);
|
|
}
|
|
|
|
[DllImport("libgdk_pixbuf-2.0-0.dll")]
|
|
static extern unsafe IntPtr gdk_pixbuf_new_from_inline(int len, IntPtr data, bool copy_pixels, out IntPtr error);
|
|
|
|
public unsafe Pixbuf(int data_length, void *data, bool copy_pixels) : base (IntPtr.Zero)
|
|
{
|
|
IntPtr error = IntPtr.Zero;
|
|
Raw = gdk_pixbuf_new_from_inline(data_length, (IntPtr) data, copy_pixels, out error);
|
|
if (error != IntPtr.Zero) throw new GLib.GException (error);
|
|
}
|
|
|
|
//
|
|
// ICloneable interface
|
|
//
|
|
|
|
public object Clone ()
|
|
{
|
|
return Copy ();
|
|
}
|
|
|
|
|
|
//
|
|
// These are factory versions of a couple of existing methods, but simplify
|
|
// the process by reducing the number of steps required. Here a single
|
|
// operation will create the Pixbuf instead of two
|
|
//
|
|
|
|
public Gdk.Pixbuf CreateFromDrawable (Gdk.Drawable src, Gdk.Colormap cmap, int src_x, int src_y, int dest_x, int dest_y, int width, int height) {
|
|
IntPtr raw_ret = gdk_pixbuf_get_from_drawable((IntPtr) 0, src.Handle, cmap.Handle, src_x, src_y, dest_x, dest_y, width, height);
|
|
return new Pixbuf (raw_ret);
|
|
}
|
|
|
|
//
|
|
// the 'Pixels' property
|
|
//
|
|
[DllImport("libgdk_pixbuf-2.0-0.dll")]
|
|
static extern IntPtr gdk_pixbuf_get_pixels(IntPtr raw);
|
|
|
|
public unsafe IntPtr Pixels {
|
|
get {
|
|
IntPtr ret = gdk_pixbuf_get_pixels (Handle);
|
|
return ret;
|
|
}
|
|
}
|
|
|
|
[DllImport("libgdk_pixbuf-2.0-0.dll")]
|
|
static extern IntPtr gdk_pixbuf_get_formats();
|
|
|
|
public static PixbufFormat[] Formats {
|
|
get {
|
|
IntPtr list_ptr = gdk_pixbuf_get_formats ();
|
|
if (list_ptr == IntPtr.Zero)
|
|
return new PixbufFormat [0];
|
|
GLib.SList list = new GLib.SList (list_ptr, typeof (PixbufFormat));
|
|
PixbufFormat[] result = new PixbufFormat [list.Count];
|
|
for (int i = 0; i < list.Count; i++)
|
|
result [i] = (PixbufFormat) list [i];
|
|
return result;
|
|
}
|
|
}
|
|
|