mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-11 22:51:42 +02:00
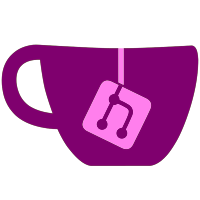
* glib/Object.cs, glib/SList.cs, glib/Value.cs, gtk/Application.cs: Move documentation to right before their actual methods, rather than the DllImported ones. * generator/Method.cs: Generate documentation before the actual method and not the DllImport. svn path=/trunk/gtk-sharp/; revision=5423
231 lines
4.3 KiB
C#
231 lines
4.3 KiB
C#
// Object.cs - GObject class wrapper implementation
|
|
//
|
|
// Authors: Bob Smith <bob@thestuff.net>
|
|
// Mike Kestner <mkestner@speakeasy.net>
|
|
//
|
|
// (c) 2001 Bob Smith and Mike Kestner
|
|
|
|
namespace GLib {
|
|
|
|
using System;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Runtime.InteropServices;
|
|
|
|
/// <summary>
|
|
/// Object Class
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Wrapper class for GObject.
|
|
/// </remarks>
|
|
|
|
public class Object : IWrapper {
|
|
|
|
// Private class and instance members
|
|
IntPtr _obj;
|
|
EventHandlerList _events;
|
|
Hashtable Data;
|
|
static Hashtable Objects = new Hashtable();
|
|
|
|
/// <summary>
|
|
/// GetObject Shared Method
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Used to obtain a CLI typed object associated with a
|
|
/// given raw object pointer. This method is primarily
|
|
/// used to wrap object references that are returned
|
|
/// by either the signal system or raw class methods that
|
|
/// return GObject references.
|
|
/// </remarks>
|
|
///
|
|
/// <returns>
|
|
/// The wrapper instance.
|
|
/// </returns>
|
|
|
|
public static Object GetObject(IntPtr o)
|
|
{
|
|
Object obj = (Object)Objects[(int)o];
|
|
if (obj != null) return obj;
|
|
return null; //FIXME: Call TypeParser here eventually.
|
|
}
|
|
|
|
/// <summary>
|
|
/// Object Constructor
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Dummy constructor needed for derived classes.
|
|
/// </remarks>
|
|
|
|
public Object () {}
|
|
|
|
/// <summary>
|
|
/// Object Constructor
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Creates an object from a raw object reference.
|
|
/// </remarks>
|
|
|
|
public Object (IntPtr raw)
|
|
{
|
|
Raw = raw;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Raw Property
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// The raw GObject reference associated with this wrapper.
|
|
/// Only subclasses of Object can access this read/write
|
|
/// property. For public read-only access, use the
|
|
/// Handle property.
|
|
/// </remarks>
|
|
|
|
protected IntPtr Raw {
|
|
get {
|
|
return _obj;
|
|
}
|
|
set {
|
|
Objects [value] = this;
|
|
_obj = value;
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Handle Property
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// The raw GObject reference associated with this object.
|
|
/// Subclasses can use Raw property for read/write
|
|
/// access.
|
|
/// </remarks>
|
|
|
|
public IntPtr Handle {
|
|
get {
|
|
return _obj;
|
|
}
|
|
set {
|
|
_obj = value;
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// EventList Property
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// A list object containing all the events for this
|
|
/// object indexed by the Gtk+ signal name.
|
|
/// </remarks>
|
|
|
|
protected EventHandlerList EventList {
|
|
get {
|
|
if (_events == null)
|
|
_events = new EventHandlerList ();
|
|
return _events;
|
|
}
|
|
}
|
|
|
|
/// <summary>
|
|
/// Equals Method
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Checks equivalence of two Objects.
|
|
/// </remarks>
|
|
|
|
public override bool Equals (object o)
|
|
{
|
|
if (!(o is Object))
|
|
return false;
|
|
|
|
return (Handle == ((Object) o).Handle);
|
|
}
|
|
|
|
/// <summary>
|
|
/// GetHashCode Method
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Calculates a hashing value.
|
|
/// </remarks>
|
|
|
|
public override int GetHashCode ()
|
|
{
|
|
return Handle.GetHashCode ();
|
|
}
|
|
|
|
/// <summary>
|
|
/// GetData Method
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Accesses arbitrary data storage on the Object.
|
|
/// </remarks>
|
|
|
|
public object GetData (string key)
|
|
{
|
|
if (Data == null)
|
|
return String.Empty;
|
|
|
|
return Data [key];
|
|
}
|
|
|
|
/// <summary>
|
|
/// SetData Method
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Stores arbitrary data on the Object.
|
|
/// </remarks>
|
|
|
|
public void SetData (string key, object val)
|
|
{
|
|
if (Data == null)
|
|
Data = new Hashtable ();
|
|
|
|
Data [key] = val;
|
|
}
|
|
|
|
[DllImport("gobject-2.0")]
|
|
static extern void g_object_get_property (
|
|
IntPtr obj, string name, IntPtr val);
|
|
|
|
/// <summary>
|
|
/// GetProperty Method
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Accesses a Value Property.
|
|
/// </remarks>
|
|
|
|
public void GetProperty (String name, GLib.Value val)
|
|
{
|
|
g_object_get_property (Raw, name, val.Handle);
|
|
}
|
|
|
|
[DllImport("gobject-2.0")]
|
|
static extern void g_object_set_property (
|
|
IntPtr obj, string name, IntPtr val);
|
|
|
|
/// <summary>
|
|
/// SetProperty Method
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Accesses a Value Property.
|
|
/// </remarks>
|
|
|
|
public void SetProperty (String name, GLib.Value val)
|
|
{
|
|
g_object_set_property (Raw, name, val.Handle);
|
|
}
|
|
|
|
}
|
|
}
|