mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-29 14:12:07 +02:00
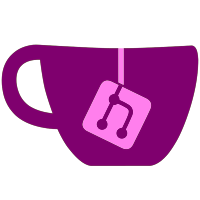
* generator/CodeGenerator.cs: add a --glue-includes flag * generator/GenerationInfo.cs: Accept glue_includes value from Main and output it to the glue_filename. * generator/FieldBase.cs (Ignored): handle more ignorable cases. (CheckGlue): New method to figure out what kind of glue we'll need for a field. (GenerateImports): generate appropriate imports per CheckGlue. (GenerateGlue): Generate C glue for accessing a struct field; either a fully-C-based accessor, or a method to just return the field's offset in the struct. (Generate): Use the generated glue to read the field. * generator/PropertyBase.cs (CType): if the field is a single bit, set its type to gboolean. * generator/ObjectGen.cs (Generate): * generator/OpaqueGen.cs (Generate): Call GenFields. * generator/StructField.cs: Use FieldBase's glue-generation code to handle bitfields. [#54489] * generator/ObjectField.cs: Generates accessors for public fields of objects and opaque structs. [#69514] * generator/ClassBase.cs (ClassBase): Parse <fields> nodes and create ObjectField objects. (GenFields): Output field properties (IgnoreMethod): Ignore Get/Set methods that duplicate fields * generator/Makefile.am (sources): update * {gdk,gnome,gtk,pango}/*.metadata: Mark some additional fields as public. Rename/retype some fields for consistency with earlier hand-coded bindings. * {gdk,gnome,gtk,pango}/*.custom: Remove custom methods that can now be autogenerated. * {gdk,gnome,gtk,pango}/glue/*.c: Remove glue methods that can now be autogenerated * {gdk,glade,gnome,gtk,pango,vte}/Makefile.am * {gdk,glade,gnome,gtk,pango,vte}/glue/Makefile.am * {gdk,gnome,gtk,pango}/glue/makefile.win32: Update svn path=/trunk/gtk-sharp/; revision=44563
217 lines
8.9 KiB
Plaintext
217 lines
8.9 KiB
Plaintext
//
|
|
// Gnome.CanvasItem.custom - Gnome CanvasItem class customizations
|
|
//
|
|
// Author: Rachel Hestilow <hestilow@ximian.com>
|
|
//
|
|
// Copyright (C) 2002 Rachel Hestilow
|
|
//
|
|
// This code is inserted after the automatically generated code.
|
|
//
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of version 2 of the Lesser GNU General
|
|
// Public License as published by the Free Software Foundation.
|
|
//
|
|
// This program is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public
|
|
// License along with this program; if not, write to the
|
|
// Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
// Boston, MA 02111-1307, USA.
|
|
|
|
[DllImport("gnomecanvas-2")]
|
|
static extern System.IntPtr gnome_canvas_item_new (IntPtr group, GLib.GType type, IntPtr null_terminator);
|
|
|
|
[DllImport("libgobject-2.0-0.dll")]
|
|
static extern void g_object_ref (IntPtr raw);
|
|
|
|
public CanvasItem (Gnome.CanvasGroup group, GLib.GType type)
|
|
: base (gnome_canvas_item_new (group.Handle, type, IntPtr.Zero))
|
|
{
|
|
g_object_ref (Handle);
|
|
}
|
|
|
|
[DllImport("gnomecanvas-2")]
|
|
static extern void gnome_canvas_item_i2c_affine(IntPtr raw, double[] affine);
|
|
|
|
public void I2cAffine(out double[] affine) {
|
|
affine = new double [6];
|
|
gnome_canvas_item_i2c_affine(Handle, affine);
|
|
}
|
|
|
|
[DllImport("gnomecanvas-2")]
|
|
static extern void gnome_canvas_item_i2w_affine(IntPtr raw, double[] affine);
|
|
|
|
public void I2wAffine(out double[] affine) {
|
|
affine = new double [6];
|
|
gnome_canvas_item_i2w_affine(Handle, affine);
|
|
}
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_base_realize (IntPtr handle);
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_override_realize (GLib.GType gtype, RealizeDelegate cb);
|
|
|
|
[GLib.CDeclCallback]
|
|
delegate void RealizeDelegate (IntPtr item);
|
|
|
|
static RealizeDelegate RealizeCallback;
|
|
|
|
static void Realize_cb (IntPtr item)
|
|
{
|
|
CanvasItem obj = GLib.Object.GetObject (item, false) as CanvasItem;
|
|
obj.Realize ();
|
|
}
|
|
|
|
static void OverrideRealize (GLib.GType gtype)
|
|
{
|
|
if (RealizeCallback == null)
|
|
RealizeCallback = new RealizeDelegate (Realize_cb);
|
|
gnomesharp_canvas_item_override_realize (gtype, RealizeCallback);
|
|
}
|
|
|
|
[GLib.DefaultSignalHandler (Type=typeof(Gnome.CanvasItem), ConnectionMethod="OverrideRealize")]
|
|
protected virtual void Realize ()
|
|
{
|
|
gnomesharp_canvas_item_base_realize (Handle);
|
|
}
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern double gnomesharp_canvas_item_base_point (IntPtr handle, double x, double y, int cx, int cy, out IntPtr actual_item_handle);
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_override_point (GLib.GType gtype, PointDelegate cb);
|
|
|
|
[GLib.CDeclCallback]
|
|
delegate double PointDelegate (IntPtr item, double x, double y, int cx, int cy, out IntPtr actual_item_handle);
|
|
|
|
static PointDelegate PointCallback;
|
|
|
|
static double Point_cb (IntPtr item, double x, double y, int cx, int cy, out IntPtr actual_item_handle)
|
|
{
|
|
CanvasItem obj = GLib.Object.GetObject (item, false) as CanvasItem;
|
|
CanvasItem actual_item;
|
|
double result = obj.Point (x, y, cx, cy, out actual_item);
|
|
actual_item_handle = actual_item != null ? actual_item.Handle : IntPtr.Zero;
|
|
return result;
|
|
}
|
|
|
|
static void OverridePoint (GLib.GType gtype)
|
|
{
|
|
if (PointCallback == null)
|
|
PointCallback = new PointDelegate (Point_cb);
|
|
gnomesharp_canvas_item_override_point (gtype, PointCallback);
|
|
}
|
|
|
|
[GLib.DefaultSignalHandler (Type=typeof(Gnome.CanvasItem), ConnectionMethod="OverridePoint")]
|
|
protected virtual double Point (double x, double y, int cx, int cy, out CanvasItem actual_item)
|
|
{
|
|
IntPtr actual_item_handle;
|
|
double result = gnomesharp_canvas_item_base_point (Handle, x, y, cx, cy, out actual_item_handle);
|
|
actual_item = GLib.Object.GetObject (actual_item_handle, false) as CanvasItem;
|
|
return result;
|
|
}
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_base_draw (IntPtr handle, IntPtr drawable, int x, int y, int width, int height);
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_override_draw (GLib.GType gtype, DrawDelegate cb);
|
|
|
|
[GLib.CDeclCallback]
|
|
delegate void DrawDelegate (IntPtr handle, IntPtr drawable, int x, int y, int width, int height);
|
|
|
|
static DrawDelegate DrawCallback;
|
|
|
|
static void Draw_cb (IntPtr handle, IntPtr drawable_handle, int x, int y, int width, int height)
|
|
{
|
|
CanvasItem obj = GLib.Object.GetObject (handle, false) as CanvasItem;
|
|
Gdk.Drawable drawable = GLib.Object.GetObject (drawable_handle, false) as Gdk.Drawable;
|
|
obj.Draw (drawable, x, y, width, height);
|
|
}
|
|
|
|
static void OverrideDraw (GLib.GType gtype)
|
|
{
|
|
if (DrawCallback == null)
|
|
DrawCallback = new DrawDelegate (Draw_cb);
|
|
gnomesharp_canvas_item_override_draw (gtype, DrawCallback);
|
|
}
|
|
|
|
[GLib.DefaultSignalHandler (Type=typeof(Gnome.CanvasItem), ConnectionMethod="OverrideDraw")]
|
|
protected virtual void Draw (Gdk.Drawable drawable, int x, int y, int width, int height)
|
|
{
|
|
gnomesharp_canvas_item_base_draw (Handle, drawable.Handle, x, y, width, height);
|
|
}
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_base_render (IntPtr handle, ref CanvasBuf buf);
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_override_render (GLib.GType gtype, RenderDelegate cb);
|
|
|
|
[GLib.CDeclCallback]
|
|
delegate void RenderDelegate (IntPtr handle, ref CanvasBuf buf);
|
|
|
|
static RenderDelegate RenderCallback;
|
|
|
|
static void Render_cb (IntPtr handle, ref CanvasBuf buf)
|
|
{
|
|
CanvasItem obj = GLib.Object.GetObject (handle, false) as CanvasItem;
|
|
obj.Render (ref buf);
|
|
}
|
|
|
|
[GLib.DefaultSignalHandler (Type=typeof(Gnome.CanvasItem), ConnectionMethod="OverrideRender")]
|
|
static void OverrideRender (GLib.GType gtype)
|
|
{
|
|
if (RenderCallback == null)
|
|
RenderCallback = new RenderDelegate (Render_cb);
|
|
gnomesharp_canvas_item_override_render (gtype, RenderCallback);
|
|
}
|
|
|
|
protected virtual void Render (ref CanvasBuf buf)
|
|
{
|
|
gnomesharp_canvas_item_base_render (Handle, ref buf);
|
|
}
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_base_update (IntPtr handle, double[] affine, ref Art.SVP clip_path, int flags);
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern void gnomesharp_canvas_item_override_update (GLib.GType gtype, UpdateDelegate cb);
|
|
|
|
[GLib.CDeclCallback]
|
|
delegate void UpdateDelegate (IntPtr item, IntPtr affine_ptr, IntPtr clip_path, int flags);
|
|
|
|
static UpdateDelegate UpdateCallback;
|
|
|
|
static void Update_cb (IntPtr item, IntPtr affine_ptr, IntPtr clip_path_handle, int flags)
|
|
{
|
|
CanvasItem obj = GLib.Object.GetObject (item, false) as CanvasItem;
|
|
double[] affine = new double [6];
|
|
Marshal.Copy (affine_ptr, affine, 0, 6);
|
|
Art.SVP clip_path;
|
|
if (clip_path_handle == IntPtr.Zero)
|
|
clip_path = Art.SVP.Zero;
|
|
else
|
|
clip_path = (Art.SVP) Marshal.PtrToStructure (clip_path_handle, typeof(Art.SVP));
|
|
obj.Update (affine, ref clip_path, flags);
|
|
}
|
|
|
|
static void OverrideUpdate (GLib.GType gtype)
|
|
{
|
|
if (UpdateCallback == null)
|
|
UpdateCallback = new UpdateDelegate (Update_cb);
|
|
gnomesharp_canvas_item_override_update (gtype, UpdateCallback);
|
|
}
|
|
|
|
[GLib.DefaultSignalHandler (Type=typeof(Gnome.CanvasItem), ConnectionMethod="OverrideUpdate")]
|
|
protected virtual void Update (double[] affine, ref Art.SVP clip_path, int flags)
|
|
{
|
|
gnomesharp_canvas_item_base_update (Handle, affine, ref clip_path, flags);
|
|
}
|
|
|