mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-29 14:12:07 +02:00
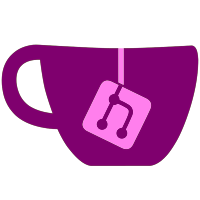
* */*.cs : s/glue-2.0/glue-2 so that dllimport works on win32. * */*.custom : s/glue-2.0/glue-2 * */glue/makefile.win32 : s/glue-2.0/glue-2 * */glue/Makefile.am : s/glue-2.0/glue-2 svn path=/trunk/gtk-sharp/; revision=37324
96 lines
2.8 KiB
Plaintext
96 lines
2.8 KiB
Plaintext
//
|
|
// Gnome.Program.custom - Gnome Program class customizations
|
|
//
|
|
// Author: Rachel Hestilow <hestilow@ximian.com>
|
|
//
|
|
// Copyright (C) 2002 Rachel Hestilow
|
|
//
|
|
// This code is inserted after the automatically generated code.
|
|
//
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of version 2 of the Lesser GNU General
|
|
// Public License as published by the Free Software Foundation.
|
|
//
|
|
// This program is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public
|
|
// License along with this program; if not, write to the
|
|
// Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
// Boston, MA 02111-1307, USA.
|
|
|
|
|
|
[DllImport("libgobject-2.0-0.dll")]
|
|
static extern void g_type_init ();
|
|
|
|
[StructLayout(LayoutKind.Sequential)]
|
|
struct PropertyArg {
|
|
public string name;
|
|
public GLib.Value value;
|
|
}
|
|
|
|
[DllImport("gnomesharpglue-2")]
|
|
static extern System.IntPtr
|
|
gtksharp_gnome_program_init (string app_id, string app_version, ref ModuleInfo module, int argc, string[] argv, int nargs, PropertyArg[] args);
|
|
|
|
public Program (string app_id, string app_version, ModuleInfo module, string[] argv, params object[] props) : base (IntPtr.Zero)
|
|
{
|
|
if (app_id.IndexOf (" ") > 0)
|
|
throw new ArgumentException ("app_id argument can't contain spaces.");
|
|
|
|
int nargs = props.Length / 2;
|
|
PropertyArg[] args = new PropertyArg[nargs];
|
|
GLib.Value[] vals = new GLib.Value[nargs];
|
|
string[] new_argv = new string[argv.Length + 1];
|
|
|
|
g_type_init ();
|
|
|
|
for (int i = 0; i < nargs; i++)
|
|
{
|
|
args[i].name = (string) props[i * 2];
|
|
GLib.Value value;
|
|
// FIXME: handle more types
|
|
object prop = props[i * 2 + 1];
|
|
Type type = prop.GetType ();
|
|
if (type == "hello".GetType ())
|
|
value = new GLib.Value ((string) prop);
|
|
else if (type == true.GetType ())
|
|
value = new GLib.Value ((bool) prop);
|
|
else
|
|
value = GLib.Value.Empty;
|
|
vals[i] = value;
|
|
args[i].value = value;
|
|
}
|
|
|
|
/* FIXME: Is there a way to access this in .NET? */
|
|
new_argv[0] = app_id;
|
|
Array.Copy (argv, 0, new_argv, 1, argv.Length);
|
|
Raw = gtksharp_gnome_program_init (app_id, app_version, ref module, new_argv.Length, new_argv, nargs, args);
|
|
|
|
//
|
|
// Dynamically reset the signal handlers, because Gnome overwrites them
|
|
//
|
|
Type mono_runtime_type = Type.GetType ("Mono.Runtime");
|
|
if (mono_runtime_type != null){
|
|
object [] iargs = new object [0];
|
|
System.Reflection.MethodInfo mi = mono_runtime_type.GetMethod ("InstallSignalHandlers", System.Reflection.BindingFlags.NonPublic | System.Reflection.BindingFlags.Static);
|
|
|
|
mi.Invoke (null, iargs);
|
|
}
|
|
}
|
|
|
|
public void Run ()
|
|
{
|
|
Gtk.Application.Run ();
|
|
}
|
|
|
|
public void Quit ()
|
|
{
|
|
Gtk.Application.Quit ();
|
|
}
|
|
|
|
|