mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-11 14:41:43 +02:00
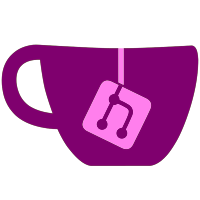
* */*.cs : add lgpl license blurb and clean up (c)'s. * */*.custom : add lgpl license blurb and clean up (c)'s. * */glue/*.c : add lgpl license blurb and clean up (c)'s. file adds without license from now on are punishable by wedgie. svn path=/trunk/gtk-sharp/; revision=30401
138 lines
4.1 KiB
Plaintext
138 lines
4.1 KiB
Plaintext
// Global.custom - customizations to Gdk.Global
|
|
//
|
|
// Authors: Mike Kestner <mkestner@ximian.com>
|
|
// Boyd Timothy <btimothy@novell.com>
|
|
//
|
|
// Copyright (c) 2004 Novell, Inc.
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of version 2 of the Lesser GNU General
|
|
// Public License as published by the Free Software Foundation.
|
|
//
|
|
// This program is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public
|
|
// License along with this program; if not, write to the
|
|
// Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
// Boston, MA 02111-1307, USA.
|
|
|
|
[DllImport("libgdk-win32-2.0-0.dll")]
|
|
static extern IntPtr gdk_devices_list ();
|
|
|
|
public static Device[] DevicesList ()
|
|
{
|
|
IntPtr raw_ret = gdk_devices_list ();
|
|
if (raw_ret == IntPtr.Zero)
|
|
return new Device [0];
|
|
GLib.List list = new GLib.List(raw_ret);
|
|
Device[] result = new Device [list.Count];
|
|
for (int i = 0; i < list.Count; i++)
|
|
result [i] = list [i] as Device;
|
|
return result;
|
|
}
|
|
|
|
[DllImport("libgdk-win32-2.0-0.dll")]
|
|
static extern IntPtr gdk_list_visuals ();
|
|
|
|
public static Visual[] ListVisuals ()
|
|
{
|
|
IntPtr raw_ret = gdk_list_visuals ();
|
|
if (raw_ret == IntPtr.Zero)
|
|
return new Visual [0];
|
|
GLib.List list = new GLib.List(raw_ret);
|
|
Visual[] result = new Visual [list.Count];
|
|
for (int i = 0; i < list.Count; i++)
|
|
result [i] = list [i] as Visual;
|
|
return result;
|
|
}
|
|
|
|
[DllImport ("gdksharpglue")]
|
|
static extern IntPtr gtksharp_get_gdk_net_supported ();
|
|
|
|
public static Gdk.Atom[] SupportedWindowManagerHints {
|
|
get {
|
|
IntPtr raw_ret = gtksharp_get_gdk_net_supported ();
|
|
if (raw_ret == IntPtr.Zero)
|
|
return new Gdk.Atom [0];
|
|
GLib.List list = new GLib.List (raw_ret, typeof (Gdk.Atom));
|
|
Gdk.Atom[] atoms = new Gdk.Atom [list.Count];
|
|
for (int i = 0; i < list.Count; i++)
|
|
atoms [i] = list [i] as Gdk.Atom;
|
|
|
|
return atoms;
|
|
}
|
|
}
|
|
|
|
[DllImport ("gdksharpglue")]
|
|
static extern IntPtr gtksharp_get_gdk_net_client_list (out int count);
|
|
|
|
public static Gdk.Window[] WindowManagerClientWindows {
|
|
get {
|
|
int count;
|
|
IntPtr raw_ret = gtksharp_get_gdk_net_client_list (out count);
|
|
if (raw_ret == IntPtr.Zero)
|
|
return new Gdk.Window [0];
|
|
Gdk.Window [] windows = new Gdk.Window [count];
|
|
int offset = 0;
|
|
for (int i = 0; i < count; i++) {
|
|
int windowID = Marshal.ReadInt32 (raw_ret, offset);
|
|
Console.WriteLine ("WinID: {0}", windowID);
|
|
offset += IntPtr.Size;
|
|
windows [i] = Gdk.Window.ForeignNew ((uint) windowID);
|
|
}
|
|
return windows;
|
|
}
|
|
}
|
|
|
|
[DllImport ("gdksharpglue")]
|
|
static extern int gtksharp_get_gdk_net_number_of_desktops ();
|
|
|
|
public static int NumberOfDesktops {
|
|
get {
|
|
return gtksharp_get_gdk_net_number_of_desktops ();
|
|
}
|
|
}
|
|
|
|
[DllImport ("gdksharpglue")]
|
|
static extern int gtksharp_get_gdk_net_current_desktop ();
|
|
|
|
public static int CurrentDesktop {
|
|
get {
|
|
return gtksharp_get_gdk_net_current_desktop ();
|
|
}
|
|
}
|
|
|
|
[DllImport ("gdksharpglue")]
|
|
static extern uint gtksharp_get_gdk_net_active_window ();
|
|
|
|
public static Gdk.Window ActiveWindow {
|
|
get {
|
|
uint windowID = gtksharp_get_gdk_net_active_window ();
|
|
if (windowID == 0)
|
|
return Gdk.Global.DefaultRootWindow;
|
|
Console.WriteLine ("Active Window ID: {0}", windowID);
|
|
Gdk.Window window = Gdk.Window.ForeignNew (windowID);
|
|
return window;
|
|
}
|
|
}
|
|
|
|
[DllImport ("gdksharpglue")]
|
|
static extern IntPtr gtksharp_get_gdk_net_workarea ();
|
|
|
|
public static Gdk.Rectangle[] DesktopWorkareas {
|
|
get {
|
|
IntPtr raw_ret = gtksharp_get_gdk_net_workarea ();
|
|
if (raw_ret == IntPtr.Zero)
|
|
return new Gdk.Rectangle [0];
|
|
GLib.List list = new GLib.List (raw_ret, typeof (Gdk.Rectangle));
|
|
Gdk.Rectangle[] workareas = new Gdk.Rectangle [list.Count];
|
|
for (int i = 0; i < list.Count; i++)
|
|
workareas [i] = (Gdk.Rectangle) list [i];
|
|
|
|
return workareas;
|
|
}
|
|
}
|