mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-09-16 11:34:47 +02:00
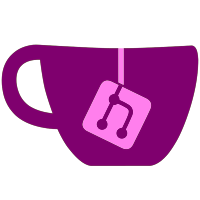
properties can't be set until Validate-time (eg, Method.IsGetter), but it's annoying for every potential user of those properties to have to make sure it has Validated the generatable first. So now we add an explicit Validate() step after everything is loaded but before anything is Generated, so that at Generation time, everything can be assumed to have been Validated. * generator/IGeneratable.cs: add "bool Validate()" * generator/CodeGenerator.cs (Main): after loading all of the generatables, DeAlias the SymbolTable, Validate() all the generatables, and discard any invalid ones. * generator/*.cs: Implement Validate() trivially in generatables that didn't implement it before. Move Validate() calls from Generate() to Validate(). Remove non-hierarchical Validate() calls. * generator/SymbolTable.cs: GPtrArray is IntPtr, not IntPtr[] svn path=/trunk/gtk-sharp/; revision=48046
213 lines
4.9 KiB
C#
213 lines
4.9 KiB
C#
// GtkSharp.Generation.StructBase.cs - The Structure/Boxed Base Class.
|
|
//
|
|
// Author: Mike Kestner <mkestner@speakeasy.net>
|
|
//
|
|
// Copyright (c) 2001-2003 Mike Kestner
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of version 2 of the GNU General Public
|
|
// License as published by the Free Software Foundation.
|
|
//
|
|
// This program is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU General Public
|
|
// License along with this program; if not, write to the
|
|
// Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
// Boston, MA 02111-1307, USA.
|
|
|
|
|
|
namespace GtkSharp.Generation {
|
|
|
|
using System;
|
|
using System.Collections;
|
|
using System.IO;
|
|
using System.Text.RegularExpressions;
|
|
using System.Xml;
|
|
|
|
public abstract class StructBase : ClassBase {
|
|
|
|
new ArrayList fields = new ArrayList ();
|
|
|
|
protected StructBase (XmlElement ns, XmlElement elem) : base (ns, elem)
|
|
{
|
|
foreach (XmlNode node in elem.ChildNodes) {
|
|
|
|
if (!(node is XmlElement)) continue;
|
|
XmlElement member = (XmlElement) node;
|
|
|
|
switch (node.Name) {
|
|
case "field":
|
|
fields.Add (new StructField (member, this));
|
|
break;
|
|
|
|
case "callback":
|
|
Statistics.IgnoreCount++;
|
|
break;
|
|
|
|
default:
|
|
if (!IsNodeNameHandled (node.Name))
|
|
Console.WriteLine ("Unexpected node " + node.Name + " in " + CName);
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
|
|
public override string MarshalType {
|
|
get
|
|
{
|
|
return "ref " + QualifiedName;
|
|
}
|
|
}
|
|
|
|
public override string MarshalReturnType {
|
|
get
|
|
{
|
|
return "IntPtr";
|
|
}
|
|
}
|
|
|
|
public override string ToNativeReturnType {
|
|
get
|
|
{
|
|
return QualifiedName;
|
|
}
|
|
}
|
|
|
|
public override string CallByName (string var_name)
|
|
{
|
|
return "ref " + var_name;
|
|
}
|
|
|
|
public override string CallByName ()
|
|
{
|
|
return "ref this";
|
|
}
|
|
|
|
public override string AssignToName {
|
|
get { return "raw"; }
|
|
}
|
|
|
|
public override string FromNative(string var)
|
|
{
|
|
return var;
|
|
}
|
|
|
|
public override string FromNativeReturn(string var)
|
|
{
|
|
return QualifiedName + ".New (" + var + ")";
|
|
}
|
|
|
|
public override string ToNativeReturn(string var)
|
|
{
|
|
// FIXME
|
|
return var;
|
|
}
|
|
|
|
private bool DisableNew {
|
|
get {
|
|
return Elem.HasAttribute ("disable_new");
|
|
}
|
|
}
|
|
|
|
protected new void GenFields (GenerationInfo gen_info)
|
|
{
|
|
int bitfields = 0;
|
|
bool need_field = true;
|
|
|
|
foreach (StructField field in fields) {
|
|
if (field.IsBitfield) {
|
|
if (need_field) {
|
|
StreamWriter sw = gen_info.Writer;
|
|
|
|
sw.WriteLine ("\t\tprivate uint _bitfield{0};\n", bitfields++);
|
|
need_field = false;
|
|
}
|
|
} else
|
|
need_field = true;
|
|
field.Generate (gen_info, "\t\t");
|
|
}
|
|
}
|
|
|
|
public override bool Validate ()
|
|
{
|
|
foreach (StructField field in fields) {
|
|
if (!field.Validate ()) {
|
|
Console.WriteLine ("in Struct " + QualifiedName);
|
|
if (!field.IsPointer)
|
|
return false;
|
|
}
|
|
}
|
|
|
|
return base.Validate ();
|
|
}
|
|
|
|
public override void Generate (GenerationInfo gen_info)
|
|
{
|
|
bool need_close = false;
|
|
if (gen_info.Writer == null) {
|
|
gen_info.Writer = gen_info.OpenStream (Name);
|
|
need_close = true;
|
|
}
|
|
|
|
StreamWriter sw = gen_info.Writer;
|
|
|
|
sw.WriteLine ("namespace " + NS + " {");
|
|
sw.WriteLine ();
|
|
sw.WriteLine ("\tusing System;");
|
|
sw.WriteLine ("\tusing System.Collections;");
|
|
sw.WriteLine ("\tusing System.Runtime.InteropServices;");
|
|
sw.WriteLine ();
|
|
|
|
sw.WriteLine ("#region Autogenerated code");
|
|
sw.WriteLine ("\t[StructLayout(LayoutKind.Sequential)]");
|
|
sw.WriteLine ("\tpublic struct " + Name + " {");
|
|
sw.WriteLine ();
|
|
|
|
GenFields (gen_info);
|
|
sw.WriteLine ();
|
|
GenCtors (gen_info);
|
|
GenMethods (gen_info, null, null);
|
|
|
|
if (!need_close)
|
|
return;
|
|
|
|
sw.WriteLine ("#endregion");
|
|
AppendCustom(sw, gen_info.CustomDir);
|
|
|
|
sw.WriteLine ("\t}");
|
|
sw.WriteLine ("}");
|
|
sw.Close ();
|
|
gen_info.Writer = null;
|
|
}
|
|
|
|
protected override void GenCtors (GenerationInfo gen_info)
|
|
{
|
|
StreamWriter sw = gen_info.Writer;
|
|
|
|
sw.WriteLine ("\t\tpublic static {0} Zero = new {0} ();", QualifiedName);
|
|
sw.WriteLine();
|
|
if (!DisableNew) {
|
|
sw.WriteLine ("\t\tpublic static " + QualifiedName + " New(IntPtr raw) {");
|
|
sw.WriteLine ("\t\t\tif (raw == IntPtr.Zero) {");
|
|
sw.WriteLine ("\t\t\t\treturn {0}.Zero;", QualifiedName);
|
|
sw.WriteLine ("\t\t\t}");
|
|
sw.WriteLine ("\t\t\t{0} self = new {0}();", QualifiedName);
|
|
sw.WriteLine ("\t\t\tself = ({0}) Marshal.PtrToStructure (raw, self.GetType ());", QualifiedName);
|
|
sw.WriteLine ("\t\t\treturn self;");
|
|
sw.WriteLine ("\t\t}");
|
|
sw.WriteLine ();
|
|
}
|
|
|
|
foreach (Ctor ctor in Ctors)
|
|
ctor.IsStatic = true;
|
|
|
|
base.GenCtors (gen_info);
|
|
}
|
|
|
|
}
|
|
}
|
|
|