mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-24 19:52:10 +02:00
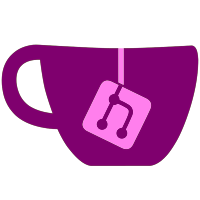
Potentially all these IDisposable classes could be used after being disposed, which would result in native crashes. We now do an explicit check and throw an exception in managed land when the object has been disposed. This is particularly useful because: a/ the native crashes are quite obscure, there no indication that you're using a disposed object b/ Gtk# is passing Context instances to user methods, and disposes them when the method returns. So if the user code keeps a reference to the Context, there a good chance it will try to use it after it's disposed. Other changes in this patch include: * Renaming a parameter to be more consistent with the other subsequent ctor called. * Replacing implementation of some [Obsolete()] methods with the call to the methods they were replaced with, to avoid redundancy and the need for more CheckDisposed() calls than necessary. * Throw ArgumentException when receiving an IntPtr.Zero as a handle, as a way to protect ourselves from wrapping invalid native pointers, and throwing ObjectDisposedExceptions because the object was invalid in the first place. Signed-off-by: Bertrand Lorentz <bertrand.lorentz@gmail.com>
52 lines
1.6 KiB
C#
52 lines
1.6 KiB
C#
//
|
|
// Mono.Cairo.PdfSurface.cs
|
|
//
|
|
// Authors:
|
|
// John Luke
|
|
//
|
|
// (C) John Luke, 2006.
|
|
//
|
|
// Permission is hereby granted, free of charge, to any person obtaining
|
|
// a copy of this software and associated documentation files (the
|
|
// "Software"), to deal in the Software without restriction, including
|
|
// without limitation the rights to use, copy, modify, merge, publish,
|
|
// distribute, sublicense, and/or sell copies of the Software, and to
|
|
// permit persons to whom the Software is furnished to do so, subject to
|
|
// the following conditions:
|
|
//
|
|
// The above copyright notice and this permission notice shall be
|
|
// included in all copies or substantial portions of the Software.
|
|
//
|
|
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
|
// EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
|
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
|
// NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
|
// LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
|
// OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
|
// WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
|
//
|
|
|
|
using System;
|
|
|
|
namespace Cairo {
|
|
|
|
public class PdfSurface : Surface
|
|
{
|
|
internal PdfSurface (IntPtr handle, bool owns) : base (handle, owns)
|
|
{
|
|
}
|
|
|
|
public PdfSurface (string filename, double width, double height)
|
|
: base (NativeMethods.cairo_pdf_surface_create (filename, width, height), true)
|
|
{
|
|
}
|
|
|
|
public void SetSize (double width, double height)
|
|
{
|
|
CheckDisposed ();
|
|
NativeMethods.cairo_pdf_surface_set_size (Handle, width, height);
|
|
}
|
|
}
|
|
}
|
|
|