mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-24 19:52:10 +02:00
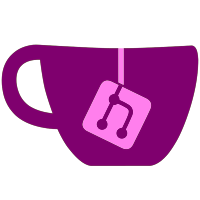
In the same way all GLib.Object-derived classes provided by the generator have a GType static property (i.e. Gtk.Widget), we need the same for the *Adapter classes, to access their GType without having an instance of it. (The first use case would be GStreamerSharp's Gst.Bin.IterateAllByInterface ().) For this, we cannot simply add the property to all adapter classes and be done, because it would clash with a property which is already there, but is non-static, that has the same name and same value (this property is needed for complying GInterfaceAdapter abstract class), so we rename this property to GInterfaceGType because using this name for the static one would not be consistent with the rest of the classes generated which already provide the static one with the name "GType".
62 lines
1.6 KiB
C#
62 lines
1.6 KiB
C#
// GInterfaceAdapter.cs
|
|
//
|
|
// Author: Mike Kestner <mkestner@novell.com>
|
|
//
|
|
// Copyright (c) 2007 Novell, Inc.
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of version 2 of the Lesser GNU General
|
|
// Public License as published by the Free Software Foundation.
|
|
//
|
|
// This program is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public
|
|
// License along with this program; if not, write to the
|
|
// Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
// Boston, MA 02111-1307, USA.
|
|
|
|
|
|
namespace GLib {
|
|
|
|
using System;
|
|
using System.Runtime.InteropServices;
|
|
|
|
[UnmanagedFunctionPointer (CallingConvention.Cdecl)]
|
|
public delegate void GInterfaceInitHandler (IntPtr iface_ptr, IntPtr data);
|
|
|
|
[UnmanagedFunctionPointer (CallingConvention.Cdecl)]
|
|
internal delegate void GInterfaceFinalizeHandler (IntPtr iface_ptr, IntPtr data);
|
|
|
|
internal struct GInterfaceInfo {
|
|
internal GInterfaceInitHandler InitHandler;
|
|
internal GInterfaceFinalizeHandler FinalizeHandler;
|
|
internal IntPtr Data;
|
|
}
|
|
|
|
public abstract class GInterfaceAdapter {
|
|
|
|
GInterfaceInfo info;
|
|
|
|
protected GInterfaceAdapter ()
|
|
{
|
|
}
|
|
|
|
protected GInterfaceInitHandler InitHandler {
|
|
set {
|
|
info.InitHandler = value;
|
|
}
|
|
}
|
|
|
|
public abstract GType GInterfaceGType { get; }
|
|
|
|
public abstract IntPtr Handle { get; }
|
|
|
|
internal GInterfaceInfo Info {
|
|
get { return info; }
|
|
}
|
|
}
|
|
}
|