mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-24 19:52:10 +02:00
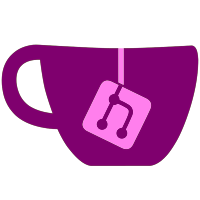
Create a GLibSynchronizationContext that sends code to be run on the GLib main loop, and set it as the current SynchronizationContext in Gtk.Init(). When you use the await keyword to do a task asynchronously, by default the awaiter will capture the current SynchronizationContext, and if there was one, when the task completes it’ll Post the supplied continuation back to that context, rather than running it on whatever thread it wants. This means that if you use the async/await keywords in your Gtk# app, things will now work as expected with the GTK main thread. For example: static async void DoWork () // called in the GTK main thread { // Do some stuff with the UI... label.Text = "Starting Work"; // Run something asynchronously, UI is not frozen int res = await DoLongOperation (); // Do some more UI stuff, it'll run on the GTK main thread label.Text = "Work done"; } Signed-off-by: Bertrand Lorentz <bertrand.lorentz@gmail.com>
72 lines
1.9 KiB
C#
72 lines
1.9 KiB
C#
//
|
|
// GLibSynchronizationContext.cs
|
|
//
|
|
// Author:
|
|
// Rickard Edström <ickard@gmail.com>
|
|
//
|
|
// Copyright (c) 2010 Rickard Edström
|
|
//
|
|
// Permission is hereby granted, free of charge, to any person obtaining a copy
|
|
// of this software and associated documentation files (the "Software"), to deal
|
|
// in the Software without restriction, including without limitation the rights
|
|
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
|
// copies of the Software, and to permit persons to whom the Software is
|
|
// furnished to do so, subject to the following conditions:
|
|
//
|
|
// The above copyright notice and this permission notice shall be included in
|
|
// all copies or substantial portions of the Software.
|
|
//
|
|
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
|
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
|
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
|
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
|
// THE SOFTWARE.
|
|
|
|
using System;
|
|
using System.Threading;
|
|
|
|
namespace GLib
|
|
{
|
|
public class GLibSynchronizationContext : SynchronizationContext
|
|
{
|
|
public override void Post (SendOrPostCallback d, object state)
|
|
{
|
|
PostAction (() => d (state));
|
|
}
|
|
|
|
public override void Send (SendOrPostCallback d, object state)
|
|
{
|
|
var mre = new ManualResetEvent (false);
|
|
Exception error = null;
|
|
|
|
PostAction (() =>
|
|
{
|
|
try {
|
|
d (state);
|
|
} catch (Exception ex) {
|
|
error = ex;
|
|
} finally {
|
|
mre.Set ();
|
|
}
|
|
});
|
|
|
|
mre.WaitOne ();
|
|
|
|
if (error != null) {
|
|
throw error;
|
|
}
|
|
}
|
|
|
|
void PostAction (Action action)
|
|
{
|
|
Idle.Add (() =>
|
|
{
|
|
action ();
|
|
return false;
|
|
});
|
|
}
|
|
}
|
|
}
|