mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-11 14:41:43 +02:00
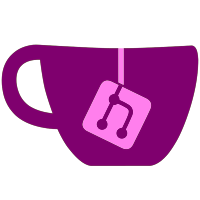
* generator/ClassBase.cs: New base class for classes and interfaces. * generator/InterfaceGen.cs: Inherit from ClassBase, generate declarations. * generator/ObjectGen.cs: Move half of this into ClassBase. * generator/Method.cs: Turn all applicable Get/Set functions into .NET accessors. Remove redundant == overload and move into Equals, as it was confusing "!= null". * generator/Parameters.cs: Alter signature creation to accept "is_set" option, add support for variable arguments. Add properties "Count", "IsVarArgs", "VAType". * generator/Ctor.cs: Fixup for changes in Parameters (indenting, signature creation). * generator/Signal.cs: Support generating declarations. * generator/SymbolTable: Change GetObjectGen to GetClassGen. * glib/IWrapper.cs: Move "Handle" declaration to here, so both classes and interfaces can benefit from it. * glib/Object.cs: Inherit from IWrapper.cs * parser/Metadata.pm: Support attribute changes on constructors, methods, signals, and paramater lists. * parser/gapi2xml.pl: Parse init funcs for interfaces. Ignore "_" functions here. * parser/gapi_pp.pl: Remove boxed_type_register check, as it will be caught in the init funcs. * parser/Atk.metadata: Added. * parser/Gtk.metadata: Add all needed signal/method collision renames. Rename GtkEditable.Editable accessors to IsEditable, as .NET does not like accessors with the same name as their declaring type. Tag TreeStore constructor as varargs. * samples/ButtonApp.cs: s/EmitAdd/Add. * samples/Menu.cs: s/EmitAdd/Add, s/Activate/Activated. svn path=/trunk/gtk-sharp/; revision=5394
317 lines
7.4 KiB
C#
317 lines
7.4 KiB
C#
// GtkSharp.Generation.Parameters.cs - The Parameters Generation Class.
|
|
//
|
|
// Author: Mike Kestner <mkestner@speakeasy.net>
|
|
//
|
|
// (c) 2001-2002 Mike Kestner
|
|
|
|
namespace GtkSharp.Generation {
|
|
|
|
using System;
|
|
using System.IO;
|
|
using System.Xml;
|
|
|
|
public class Parameters {
|
|
|
|
private XmlElement elem;
|
|
private string import_sig;
|
|
private string call_string;
|
|
private string signature;
|
|
private string signature_types;
|
|
|
|
public Parameters (XmlElement elem) {
|
|
|
|
this.elem = elem;
|
|
}
|
|
|
|
public string CallString {
|
|
get {
|
|
return call_string;
|
|
}
|
|
}
|
|
|
|
public string ImportSig {
|
|
get {
|
|
return import_sig;
|
|
}
|
|
}
|
|
|
|
public string Signature {
|
|
get {
|
|
return signature;
|
|
}
|
|
}
|
|
|
|
public string SignatureTypes {
|
|
get {
|
|
return signature_types;
|
|
}
|
|
}
|
|
|
|
public bool Validate ()
|
|
{
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
string type = p_elem.GetAttribute("type");
|
|
string cs_type = SymbolTable.GetCSType(type);
|
|
string m_type = SymbolTable.GetMarshalType(type);
|
|
string name = MangleName(p_elem.GetAttribute("name"));
|
|
string call_parm = SymbolTable.CallByName(type, name);
|
|
|
|
if ((cs_type == "") || (name == "") ||
|
|
(m_type == "") || (call_parm == "")) {
|
|
Console.Write("Name: " + name + " Type: " + type + " ");
|
|
return false;
|
|
}
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
public void CreateSignature (bool is_set)
|
|
{
|
|
signature_types = signature = import_sig = call_string = "";
|
|
bool need_sep = false;
|
|
|
|
int len = 0;
|
|
XmlElement last_param;
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
len++;
|
|
last_param = (XmlElement) parm;
|
|
}
|
|
|
|
int i = 0;
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
string type = p_elem.GetAttribute("type");
|
|
string cs_type = SymbolTable.GetCSType(type);
|
|
string m_type = SymbolTable.GetMarshalType(type);
|
|
string name = MangleName(p_elem.GetAttribute("name"));
|
|
string call_parm;
|
|
|
|
if (is_set && i == 0)
|
|
call_parm = SymbolTable.CallByName(type, "value");
|
|
else
|
|
call_parm = SymbolTable.CallByName(type, name);
|
|
|
|
if (p_elem.HasAttribute("array")) {
|
|
cs_type += "[]";
|
|
m_type += "[]";
|
|
}
|
|
|
|
if (IsVarArgs && i == (len - 1) && VAType == "length_param") {
|
|
cs_type = "params " + cs_type + "[]";
|
|
m_type += "[]";
|
|
}
|
|
|
|
if (need_sep) {
|
|
call_string += ", ";
|
|
import_sig += ", ";
|
|
if (type != "GError**" && !(IsVarArgs && i == (len - 1) && VAType == "length_param"))
|
|
{
|
|
signature += ", ";
|
|
signature_types += ":";
|
|
}
|
|
} else {
|
|
need_sep = true;
|
|
}
|
|
|
|
if (p_elem.HasAttribute("pass_as")) {
|
|
string pass_as = p_elem.GetAttribute("pass_as");
|
|
signature += pass_as + " ";
|
|
// We only need to do this for value types
|
|
if (type != "GError**" && m_type != "IntPtr" && m_type != "System.IntPtr")
|
|
{
|
|
import_sig += pass_as + " ";
|
|
call_string += "out ";
|
|
}
|
|
}
|
|
else if (type == "GError**")
|
|
{
|
|
call_string += "out ";
|
|
import_sig += "out ";
|
|
}
|
|
|
|
if (IsVarArgs && i == (len - 2) && VAType == "length_param")
|
|
{
|
|
call_string += MangleName(last_param.GetAttribute("name")) + ".Length";
|
|
}
|
|
else
|
|
{
|
|
if (type != "GError**") {
|
|
signature += (cs_type + " " + name);
|
|
signature_types += cs_type;
|
|
}
|
|
call_string += call_parm;
|
|
}
|
|
import_sig += (m_type + " " + name);
|
|
|
|
i++;
|
|
}
|
|
}
|
|
|
|
public void Initialize (StreamWriter sw, bool is_get, string indent)
|
|
{
|
|
string name = "";
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
|
|
string c_type = p_elem.GetAttribute ("type");
|
|
string type = SymbolTable.GetCSType(c_type);
|
|
name = MangleName(p_elem.GetAttribute("name"));
|
|
if (is_get) {
|
|
sw.WriteLine (indent + "\t\t\t" + type + " " + name + ";");
|
|
}
|
|
|
|
if ((is_get || (p_elem.HasAttribute("pass_as") && p_elem.GetAttribute ("pass_as") == "out")) && (SymbolTable.IsObject (c_type) || SymbolTable.IsBoxed (c_type))) {
|
|
sw.WriteLine(indent + "\t\t\t" + name + " = new " + type + "();");
|
|
}
|
|
}
|
|
|
|
if (ThrowsException)
|
|
sw.WriteLine (indent + "\t\t\tIntPtr error;");
|
|
}
|
|
/*
|
|
public void Finish (StreamWriter sw)
|
|
{
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
string c_type = p_elem.GetAttribute ("type");
|
|
string name = MangleName(p_elem.GetAttribute("name"));
|
|
|
|
if ((p_elem.HasAttribute("pass_as") && p_elem.GetAttribute ("pass_as") == "out")) {
|
|
string call_parm = SymbolTable.CallByName(c_type, name);
|
|
string local_parm = GetPossibleLocal (call_parm);
|
|
if (call_parm != local_parm)
|
|
sw.WriteLine ("\t\t\t{0} = {1};", call_parm, local_parm);
|
|
}
|
|
}
|
|
}
|
|
*/
|
|
|
|
public void HandleException (StreamWriter sw, string indent)
|
|
{
|
|
if (!ThrowsException)
|
|
return;
|
|
sw.WriteLine (indent + "\t\t\tif (error != IntPtr.Zero) throw new GLib.GException (error);");
|
|
}
|
|
|
|
public int Count {
|
|
get {
|
|
int length = 0;
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
length++;
|
|
}
|
|
return length;
|
|
}
|
|
}
|
|
|
|
public bool IsAccessor {
|
|
get {
|
|
int length = 0;
|
|
string pass_as = "";
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
length++;
|
|
if (length > 1)
|
|
return false;
|
|
if (p_elem.HasAttribute("pass_as"))
|
|
pass_as = p_elem.GetAttribute("pass_as");
|
|
}
|
|
|
|
return (length == 1 && pass_as == "out");
|
|
}
|
|
}
|
|
|
|
public bool ThrowsException {
|
|
get {
|
|
if ((elem.ChildNodes == null) || (elem.ChildNodes.Count < 1))
|
|
return false;
|
|
|
|
XmlElement p_elem = (XmlElement) elem.ChildNodes[elem.ChildNodes.Count - 1];
|
|
string type = p_elem.GetAttribute("type");
|
|
return (type == "GError**");
|
|
}
|
|
}
|
|
|
|
public bool IsVarArgs {
|
|
get {
|
|
return elem.HasAttribute ("va_type");
|
|
}
|
|
}
|
|
|
|
public string VAType {
|
|
get {
|
|
return elem.GetAttribute ("va_type");
|
|
}
|
|
}
|
|
|
|
public string AccessorReturnType {
|
|
get {
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter")
|
|
continue;
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
return SymbolTable.GetCSType(p_elem.GetAttribute ("type"));
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
|
|
public string AccessorName {
|
|
get {
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter")
|
|
continue;
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
return MangleName (p_elem.GetAttribute("name"));
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
|
|
private string MangleName(string name)
|
|
{
|
|
switch (name) {
|
|
case "string":
|
|
return "str1ng";
|
|
case "event":
|
|
return "evnt";
|
|
case "object":
|
|
return "objekt";
|
|
default:
|
|
break;
|
|
}
|
|
|
|
return name;
|
|
}
|
|
}
|
|
}
|
|
|