mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-11 14:41:43 +02:00
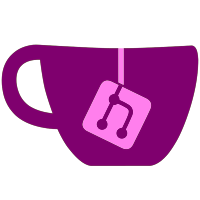
* configure.in, makefile, makefile.win32: add gnome. * doc/index.html, netdoc.xsl: Add gnome. * gdk/Event.cs: New manual wrap for GdkEvent. * generator/ClassBase.cs: Add methods GetProperty, GetPropertyRecursively, GetMethodRecursively. Move Parent property here from ObjectGen.cs. Pass this pointer into Property. * generator/Ctor.cs: Generate docs. * generator/Method.cs, Property.cs: Tag method as "new" if a Method/Property with the same name is found in the class hierarchy. * generator/SignalHandler.cs: Correctly wrap complex signal argument types. Add gnome directory. * generator/SymbolTable.cs: Add manually wrapped types hash (contains GLib.GSList and Gdk.Event). Add method IsManuallyWrapped. * glib/SList.cs: Add constructor from IntPtr. * glue/slist.c, glue/event.c: Added (field accessor glue). * glue/Makefile.am: Update. * parser/Gtk.metadata: Add new signal renames for new signals exposed by GdkEvent changes. * parser/README, parser/build.pl: Add libgnome, libgnomecanvas, libgnomeui. * parser/gapi2xml.pl: Handle literal-length array parameters, and NULL property doc strings. * sample/: Add new test GnomeHelloWorld.cs. * gnome/: Added. * parser/Gnome.metadata: Added. svn path=/trunk/gtk-sharp/; revision=5461
125 lines
3.4 KiB
C#
125 lines
3.4 KiB
C#
// GtkSharp.Generation.Ctor.cs - The Constructor Generation Class.
|
|
//
|
|
// Author: Mike Kestner <mkestner@speakeasy.net>
|
|
//
|
|
// (c) 2001-2002 Mike Kestner
|
|
|
|
namespace GtkSharp.Generation {
|
|
|
|
using System;
|
|
using System.Collections;
|
|
using System.IO;
|
|
using System.Xml;
|
|
|
|
public class Ctor {
|
|
|
|
private string libname;
|
|
private XmlElement elem;
|
|
private Parameters parms;
|
|
private bool preferred;
|
|
|
|
public bool Preferred {
|
|
get { return preferred; }
|
|
set { preferred = value; }
|
|
}
|
|
|
|
public Ctor (string libname, XmlElement elem) {
|
|
this.libname = libname;
|
|
this.elem = elem;
|
|
XmlElement parms_elem = elem ["parameters"];
|
|
if (parms_elem != null)
|
|
parms = new Parameters (parms_elem);
|
|
if (elem.HasAttribute ("preferred"))
|
|
preferred = true;
|
|
}
|
|
|
|
public bool Validate ()
|
|
{
|
|
if (parms != null) {
|
|
if (!parms.Validate ()) {
|
|
Console.Write("ctor ");
|
|
Statistics.ThrottledCount++;
|
|
return false;
|
|
}
|
|
parms.CreateSignature (false);
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
public void InitClashMap (Hashtable clash_map)
|
|
{
|
|
string sigtypes = (parms != null) ? parms.SignatureTypes : "";
|
|
if (clash_map.ContainsKey (sigtypes)) {
|
|
int num = (int) clash_map[sigtypes];
|
|
clash_map[sigtypes] = ++num;
|
|
Console.WriteLine ("CLASH: {0} {1}", elem.GetAttribute ("cname"), num);
|
|
}
|
|
else
|
|
clash_map[sigtypes] = 0;
|
|
}
|
|
|
|
public void Generate (StreamWriter sw, Hashtable clash_map)
|
|
{
|
|
string sigtypes = "";
|
|
string sig = "()";
|
|
string call = "()";
|
|
string isig = "();";
|
|
if (parms != null) {
|
|
call = "(" + parms.CallString + ")";
|
|
sig = "(" + parms.Signature + ")";
|
|
isig = "(" + parms.ImportSig + ");";
|
|
sigtypes = parms.SignatureTypes;
|
|
}
|
|
|
|
int clashes = (int) clash_map[sigtypes];
|
|
|
|
string cname = elem.GetAttribute("cname");
|
|
string name = ((XmlElement)elem.ParentNode).GetAttribute("name");
|
|
string safety;
|
|
if (parms != null && parms.ThrowsException)
|
|
safety = "unsafe ";
|
|
else
|
|
safety = "";
|
|
|
|
sw.WriteLine("\t\t[DllImport(\"" + libname + "\")]");
|
|
sw.WriteLine("\t\tstatic extern " + safety + "IntPtr " + cname + isig);
|
|
sw.WriteLine();
|
|
|
|
sw.WriteLine("\t\t/// <summary> " + name + " Constructor </summary>");
|
|
sw.WriteLine("\t\t/// <remarks> To be completed </remarks>");
|
|
if (clashes > 0 && !Preferred) {
|
|
String mname = cname.Substring(cname.IndexOf("new"));
|
|
mname = mname.Substring(0,1).ToUpper() + mname.Substring(1);
|
|
int idx;
|
|
while ((idx = mname.IndexOf("_")) > 0) {
|
|
mname = mname.Substring(0, idx) + mname.Substring(idx+1, 1).ToUpper() + mname.Substring(idx+2);
|
|
}
|
|
|
|
sw.WriteLine("\t\tpublic static " + safety + name + " " + mname + sig);
|
|
sw.WriteLine("\t\t{");
|
|
if (parms != null)
|
|
parms.Initialize(sw, false, "");
|
|
sw.WriteLine("\t\t\tIntPtr ret = " + cname + call + ";");
|
|
if (parms != null)
|
|
parms.HandleException (sw, "");
|
|
sw.WriteLine("\t\t\treturn new " + name + "(ret);");
|
|
} else {
|
|
sw.WriteLine("\t\tpublic " + safety + name + sig);
|
|
sw.WriteLine("\t\t{");
|
|
if (parms != null)
|
|
parms.Initialize(sw, false, "");
|
|
sw.WriteLine("\t\t\tRaw = " + cname + call + ";");
|
|
if (parms != null)
|
|
parms.HandleException (sw, "");
|
|
}
|
|
|
|
sw.WriteLine("\t\t}");
|
|
sw.WriteLine();
|
|
|
|
Statistics.CtorCount++;
|
|
}
|
|
}
|
|
}
|
|
|