mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-09-09 00:13:20 +02:00
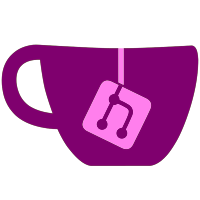
* api/*-api.xml : regenerated * gdk/Drawable.custom : DrawRectangle filled param is now bool * generator/Parameters.cs : studlify names ending w/ uscore * gtk/GtkSharp.GtkClipboardClearFuncNative : s/Opaque/Object * gtk/GtkSharp.GtkClipboardGetFuncNative : s/Opaque/Object * parser/gapi2xml.pl : put <ns>_string_* methods in Global * sample/Scribble.cs : update to new DrawRectangle api * sources/Gdk.metadata : hide some conflicting methods * sources/Gtk.metadata : finally fix the Progress crap and renames, hides and such to fix conflicts * sources/README : mention new gtk-2.2 reqs * sources/gtk-sharp.sources : update to new gtk-2.2 reqs * sources/makefile : update for 2.2 api svn path=/trunk/gtk-sharp/; revision=16254
517 lines
12 KiB
C#
517 lines
12 KiB
C#
// GtkSharp.Generation.Parameters.cs - The Parameters Generation Class.
|
|
//
|
|
// Author: Mike Kestner <mkestner@speakeasy.net>
|
|
//
|
|
// (c) 2001-2002 Mike Kestner
|
|
|
|
namespace GtkSharp.Generation {
|
|
|
|
using System;
|
|
using System.IO;
|
|
using System.Xml;
|
|
|
|
public class Parameter {
|
|
|
|
private XmlElement elem;
|
|
|
|
public Parameter (XmlElement e)
|
|
{
|
|
elem = e;
|
|
}
|
|
|
|
public string CType {
|
|
get {
|
|
string type = elem.GetAttribute("type");
|
|
if (type == "void*")
|
|
type = "gpointer";
|
|
return type;
|
|
}
|
|
}
|
|
|
|
public string CSType {
|
|
get {
|
|
string cstype = SymbolTable.Table.GetCSType( elem.GetAttribute("type"));
|
|
if (cstype == "void")
|
|
cstype = "System.IntPtr";
|
|
if (elem.HasAttribute("array")) {
|
|
cstype += "[]";
|
|
cstype = cstype.Replace ("ref ", "");
|
|
}
|
|
return cstype;
|
|
}
|
|
}
|
|
|
|
public IGeneratable Generatable {
|
|
get {
|
|
return SymbolTable.Table[CType];
|
|
}
|
|
}
|
|
|
|
public bool IsLength {
|
|
get {
|
|
return (CSType == "int" &&
|
|
(Name.EndsWith("len") || Name.EndsWith("length")));
|
|
}
|
|
}
|
|
|
|
public bool IsString {
|
|
get {
|
|
return (CSType == "string");
|
|
}
|
|
}
|
|
|
|
public string MarshalType {
|
|
get {
|
|
string type = SymbolTable.Table.GetMarshalType( elem.GetAttribute("type"));
|
|
if (type == "void")
|
|
type = "System.IntPtr";
|
|
if (elem.HasAttribute("array")) {
|
|
type += "[]";
|
|
type = type.Replace ("ref ", "");
|
|
}
|
|
return type;
|
|
}
|
|
}
|
|
|
|
public string Name {
|
|
get {
|
|
return MangleName (elem.GetAttribute("name"));
|
|
}
|
|
}
|
|
|
|
public bool NullOk {
|
|
get {
|
|
return elem.HasAttribute ("null_ok");
|
|
}
|
|
}
|
|
|
|
public string PassAs {
|
|
get {
|
|
return elem.GetAttribute ("pass_as");
|
|
}
|
|
}
|
|
|
|
private string MangleName(string name)
|
|
{
|
|
switch (name) {
|
|
case "string":
|
|
return "str1ng";
|
|
case "event":
|
|
return "evnt";
|
|
case "null":
|
|
return "is_null";
|
|
case "object":
|
|
return "objekt";
|
|
case "params":
|
|
return "parms";
|
|
case "ref":
|
|
return "reference";
|
|
case "in":
|
|
return "in_param";
|
|
case "out":
|
|
return "out_param";
|
|
case "fixed":
|
|
return "mfixed";
|
|
case "byte":
|
|
return "_byte";
|
|
case "new":
|
|
return "_new";
|
|
default:
|
|
break;
|
|
}
|
|
|
|
return name;
|
|
}
|
|
public string StudlyName {
|
|
get {
|
|
string name = elem.GetAttribute("name");
|
|
string[] segs = name.Split('_');
|
|
string studly = "";
|
|
foreach (string s in segs) {
|
|
if (s.Trim () == "")
|
|
continue;
|
|
studly += (s.Substring(0,1).ToUpper() + s.Substring(1));
|
|
}
|
|
return studly;
|
|
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
public class Parameters {
|
|
|
|
private XmlElement elem;
|
|
private string impl_ns;
|
|
private string import_sig;
|
|
private string call_string;
|
|
private string signature;
|
|
private string signature_types;
|
|
private bool hide_data;
|
|
private bool is_static;
|
|
|
|
public Parameters (XmlElement elem, string impl_ns) {
|
|
|
|
this.elem = elem;
|
|
this.impl_ns = impl_ns;
|
|
}
|
|
|
|
public string CallString {
|
|
get {
|
|
return call_string;
|
|
}
|
|
}
|
|
|
|
public Parameter this [int idx] {
|
|
get {
|
|
int count = 0;
|
|
foreach (XmlNode node in elem.ChildNodes) {
|
|
if ((node is XmlElement) && (idx == count++))
|
|
return new Parameter ((XmlElement) node);
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
|
|
public string ImportSig {
|
|
get {
|
|
return import_sig;
|
|
}
|
|
}
|
|
|
|
public string Signature {
|
|
get {
|
|
return signature;
|
|
}
|
|
}
|
|
|
|
public string SignatureTypes {
|
|
get {
|
|
return signature_types;
|
|
}
|
|
}
|
|
|
|
public bool HideData {
|
|
get { return hide_data; }
|
|
set { hide_data = value; }
|
|
}
|
|
|
|
public bool Static {
|
|
set { is_static = value; }
|
|
}
|
|
|
|
public bool Validate ()
|
|
{
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (!(parm is XmlElement) || parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
|
|
if (p_elem.HasAttribute("ellipsis")) {
|
|
Console.Write("Ellipsis parameter ");
|
|
return false;
|
|
}
|
|
|
|
Parameter p = new Parameter (p_elem);
|
|
|
|
if ((p.CSType == "") || (p.Name == "") ||
|
|
(p.MarshalType == "") || (SymbolTable.Table.CallByName(p.CType, p.Name) == "")) {
|
|
Console.Write("Name: " + p.Name + " Type: " + p.CType + " ");
|
|
return false;
|
|
}
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
public void CreateSignature (bool is_set)
|
|
{
|
|
signature_types = signature = import_sig = call_string = "";
|
|
bool need_sep = false;
|
|
bool has_callback = hide_data;
|
|
bool last_was_user_data = false;
|
|
bool has_user_data = false;
|
|
|
|
SymbolTable table = SymbolTable.Table;
|
|
int len = 0;
|
|
Parameter last_param = null;
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
if (p_elem.GetAttribute("type") == "gpointer" && (p_elem.GetAttribute("name").EndsWith ("data") || p_elem.GetAttribute("name").EndsWith ("data_or_owner")))
|
|
has_user_data = true;
|
|
len++;
|
|
last_param = new Parameter ((XmlElement) parm);
|
|
}
|
|
|
|
int i = 0;
|
|
Parameter prev = null;
|
|
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
Parameter curr = new Parameter (p_elem);
|
|
|
|
string type = curr.CType;
|
|
string cs_type = curr.CSType;
|
|
string m_type = curr.MarshalType;
|
|
string name = curr.Name;
|
|
|
|
if (prev != null && prev.IsString && curr.IsLength) {
|
|
call_string += ", " + prev.Name + ".Length";
|
|
import_sig += ", " + m_type + " " + name;
|
|
prev = curr;
|
|
i++;
|
|
continue;
|
|
}
|
|
|
|
string call_parm_name = name;
|
|
if (is_set && i == 0)
|
|
call_parm_name = "value";
|
|
|
|
string call_parm;
|
|
if (table.IsCallback (type)) {
|
|
has_callback = true;
|
|
call_parm = table.CallByName (type, call_parm_name + "_wrapper");
|
|
} else
|
|
call_parm = table.CallByName(type, call_parm_name);
|
|
|
|
if (p_elem.HasAttribute ("null_ok") && cs_type != "IntPtr" && cs_type != "System.IntPtr" && !table.IsStruct (type))
|
|
call_parm = String.Format ("({0} != null) ? {1} : {2}", call_parm_name, call_parm, table.IsCallback (type) ? "null" : "IntPtr.Zero");
|
|
|
|
if (p_elem.HasAttribute("array"))
|
|
call_parm = call_parm.Replace ("ref ", "");
|
|
|
|
if (IsVarArgs && i == (len - 1) && VAType == "length_param") {
|
|
cs_type = "params " + cs_type + "[]";
|
|
m_type += "[]";
|
|
}
|
|
|
|
if (need_sep) {
|
|
call_string += ", ";
|
|
import_sig += ", ";
|
|
if (!(type == "GError**" || last_was_user_data) && !(IsVarArgs && i == (len - 1) && VAType == "length_param"))
|
|
{
|
|
signature += ", ";
|
|
signature_types += ":";
|
|
}
|
|
} else {
|
|
need_sep = true;
|
|
}
|
|
|
|
if (p_elem.HasAttribute("pass_as")) {
|
|
string pass_as = p_elem.GetAttribute("pass_as");
|
|
signature += pass_as + " ";
|
|
// We only need to do this for value types
|
|
if (type != "GError**" && m_type != "IntPtr" && m_type != "System.IntPtr")
|
|
{
|
|
import_sig += pass_as + " ";
|
|
call_string += pass_as + " ";
|
|
}
|
|
|
|
if (table.IsEnum (type))
|
|
call_parm = name + "_as_int";
|
|
}
|
|
else if (type == "GError**")
|
|
{
|
|
call_string += "out ";
|
|
import_sig += "out ";
|
|
}
|
|
|
|
if (IsVarArgs && i == (len - 2) && VAType == "length_param")
|
|
{
|
|
call_string += last_param.Name + ".Length";
|
|
last_was_user_data = false;
|
|
}
|
|
else
|
|
{
|
|
if (!(type == "GError**" || (has_callback && (type == "gpointer" || type == "void*") && (i == Count - 1) && (name.EndsWith ("data") || name.EndsWith ("data_or_owner"))))) {
|
|
signature += (cs_type + " " + name);
|
|
signature_types += cs_type;
|
|
last_was_user_data = false;
|
|
} else if (type == "GError**") {
|
|
call_parm = call_parm.Replace (name, "error");
|
|
last_was_user_data = false;
|
|
} else if ((type == "gpointer" || type == "void*") && (i == Count - 1) && (name.EndsWith ("data") || name.EndsWith ("data_or_owner"))) {
|
|
call_parm = "IntPtr.Zero";
|
|
last_was_user_data = true;
|
|
} else
|
|
last_was_user_data = false;
|
|
|
|
call_string += call_parm;
|
|
}
|
|
if (table.IsCallback (type))
|
|
m_type = impl_ns + "Sharp" + m_type.Substring(m_type.IndexOf("."));
|
|
import_sig += (m_type + " " + name);
|
|
prev = curr;
|
|
i++;
|
|
}
|
|
|
|
// FIXME: lame
|
|
call_string = call_string.Replace ("out ref", "out");
|
|
import_sig = import_sig.Replace ("out ref", "out");
|
|
call_string = call_string.Replace ("ref ref", "ref");
|
|
import_sig = import_sig.Replace ("ref ref", "ref");
|
|
|
|
// FIXME: this is also lame, I need to fix the need_sep algo
|
|
if (signature.EndsWith (", "))
|
|
signature = signature.Remove (signature.Length - 2, 2);
|
|
}
|
|
|
|
public void Initialize (StreamWriter sw, bool is_get, bool is_set, string indent)
|
|
{
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter")
|
|
continue;
|
|
|
|
Parameter p = new Parameter ((XmlElement) parm);
|
|
IGeneratable gen = p.Generatable;
|
|
string name = p.Name;
|
|
if (is_set)
|
|
name = "value";
|
|
|
|
if (is_get)
|
|
sw.WriteLine (indent + "\t\t\t" + p.CSType + " " + name + ";");
|
|
|
|
if ((is_get || p.PassAs == "out") && (gen is ObjectGen || gen is OpaqueGen || p.CSType == "GLib.Value"))
|
|
sw.WriteLine(indent + "\t\t\t" + name + " = new " + p.CSType + "();");
|
|
|
|
if (p.PassAs == "out" && gen is EnumGen)
|
|
sw.WriteLine(indent + "\t\t\tint " + name + "_as_int;");
|
|
|
|
if (gen is CallbackGen) {
|
|
CallbackGen cbgen = gen as CallbackGen;
|
|
string wrapper = cbgen.GenWrapper(impl_ns);
|
|
sw.WriteLine (indent + "\t\t\t{0} {1}_wrapper = null;", wrapper, name);
|
|
sw.Write (indent + "\t\t\t");
|
|
if (p.NullOk)
|
|
sw.Write ("if ({0} != null) ", name);
|
|
sw.WriteLine ("{1}_wrapper = new {0} ({1}, {2});", wrapper, name, is_static ? "null" : "this");
|
|
}
|
|
}
|
|
|
|
if (ThrowsException)
|
|
sw.WriteLine (indent + "\t\t\tIntPtr error = IntPtr.Zero;");
|
|
}
|
|
|
|
public void Finish (StreamWriter sw, string indent)
|
|
{
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter")
|
|
continue;
|
|
|
|
Parameter p = new Parameter ((XmlElement) parm);
|
|
|
|
if (p.PassAs == "out" && p.Generatable is EnumGen) {
|
|
sw.WriteLine(indent + "\t\t\t" + p.Name + " = (" + p.CSType + ") " + p.Name + "_as_int;");
|
|
}
|
|
}
|
|
}
|
|
|
|
|
|
public void HandleException (StreamWriter sw, string indent)
|
|
{
|
|
if (!ThrowsException)
|
|
return;
|
|
sw.WriteLine (indent + "\t\t\tif (error != IntPtr.Zero) throw new GLib.GException (error);");
|
|
}
|
|
|
|
public int Count {
|
|
get {
|
|
int length = 0;
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
length++;
|
|
}
|
|
return length;
|
|
}
|
|
}
|
|
|
|
public bool IsAccessor {
|
|
get {
|
|
int length = 0;
|
|
string pass_as = "";
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (!(parm is XmlElement) || parm.Name != "parameter") {
|
|
continue;
|
|
}
|
|
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
length++;
|
|
if (length > 1)
|
|
return false;
|
|
if (p_elem.HasAttribute("pass_as"))
|
|
pass_as = p_elem.GetAttribute("pass_as");
|
|
}
|
|
|
|
return (length == 1 && pass_as == "out");
|
|
}
|
|
}
|
|
|
|
public bool ThrowsException {
|
|
get {
|
|
if ((elem.ChildNodes == null) || (elem.ChildNodes.Count < 1))
|
|
return false;
|
|
|
|
XmlElement p_elem = null;
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (!(parm is XmlElement) || parm.Name != "parameter")
|
|
continue;
|
|
p_elem = (XmlElement) parm;
|
|
}
|
|
string type = p_elem.GetAttribute("type");
|
|
return (type == "GError**");
|
|
}
|
|
}
|
|
|
|
public bool IsVarArgs {
|
|
get {
|
|
return elem.HasAttribute ("va_type");
|
|
}
|
|
}
|
|
|
|
public string VAType {
|
|
get {
|
|
return elem.GetAttribute ("va_type");
|
|
}
|
|
}
|
|
|
|
public string AccessorReturnType {
|
|
get {
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter")
|
|
continue;
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
return SymbolTable.Table.GetCSType(p_elem.GetAttribute ("type"));
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
|
|
public string AccessorName {
|
|
get {
|
|
foreach (XmlNode parm in elem.ChildNodes) {
|
|
if (parm.Name != "parameter")
|
|
continue;
|
|
XmlElement p_elem = (XmlElement) parm;
|
|
Parameter p = new Parameter (p_elem);
|
|
return p.Name;
|
|
}
|
|
return null;
|
|
}
|
|
}
|
|
|
|
}
|
|
}
|
|
|