mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-09-09 00:13:20 +02:00
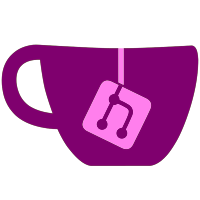
If we set it to null background is set to parent brackground. So we must handle null case and sent it to native.
189 lines
6.3 KiB
Plaintext
189 lines
6.3 KiB
Plaintext
// Gdk.Window.custom - Gdk Window class customizations
|
|
//
|
|
// Author: Moritz Balz <ich@mbalz.de>
|
|
// Mike Kestner <mkestner@ximian.com>
|
|
//
|
|
// Copyright (c) 2003 Moritz Balz
|
|
// Copyright (c) 2004 - 2008 Novell, Inc.
|
|
//
|
|
// This code is inserted after the automatically generated code.
|
|
//
|
|
// This program is free software; you can redistribute it and/or
|
|
// modify it under the terms of version 2 of the Lesser GNU General
|
|
// Public License as published by the Free Software Foundation.
|
|
//
|
|
// This program is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
// Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public
|
|
// License along with this program; if not, write to the
|
|
// Free Software Foundation, Inc., 59 Temple Place - Suite 330,
|
|
// Boston, MA 02111-1307, USA.
|
|
|
|
public Window (Gdk.Window parent, Gdk.WindowAttr attributes, Gdk.WindowAttributesType attributes_mask) : this (parent, attributes, (int)attributes_mask) {}
|
|
|
|
[DllImport("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern IntPtr gdk_window_get_background_pattern(IntPtr raw);
|
|
|
|
[DllImport("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern void gdk_window_set_background_pattern(IntPtr raw, IntPtr pattern);
|
|
|
|
public Cairo.Pattern BackgroundPattern {
|
|
get {
|
|
IntPtr raw_ret = gdk_window_get_background_pattern(Handle);
|
|
Cairo.Pattern ret = Cairo.Pattern.Lookup (raw_ret);
|
|
return ret;
|
|
}
|
|
set {
|
|
gdk_window_set_background_pattern(Handle, (value == null) ? IntPtr.Zero : value.Handle);
|
|
}
|
|
}
|
|
|
|
[DllImport ("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern IntPtr gdk_window_get_children(IntPtr raw);
|
|
|
|
public Window[] Children {
|
|
get {
|
|
IntPtr raw_ret = gdk_window_get_children(Handle);
|
|
if (raw_ret == IntPtr.Zero)
|
|
return new Window [0];
|
|
GLib.List list = new GLib.List(raw_ret);
|
|
Window[] result = new Window [list.Count];
|
|
for (int i = 0; i < list.Count; i++)
|
|
result [i] = list [i] as Window;
|
|
return result;
|
|
}
|
|
}
|
|
|
|
[DllImport ("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern void gdk_window_set_icon_list(IntPtr raw, IntPtr pixbufs);
|
|
|
|
public Pixbuf[] IconList {
|
|
set {
|
|
GLib.List list = new GLib.List(IntPtr.Zero);
|
|
foreach (Pixbuf val in value)
|
|
list.Append (val.Handle);
|
|
gdk_window_set_icon_list(Handle, list.Handle);
|
|
}
|
|
}
|
|
|
|
[DllImport ("libgobject-2.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern IntPtr g_object_ref (IntPtr raw);
|
|
|
|
[DllImport ("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern void gdk_window_destroy(IntPtr raw);
|
|
|
|
public void Destroy ()
|
|
{
|
|
// native method assumes an outstanding normal ref, but we hold a
|
|
// toggle ref. take out a normal ref for it to release, and let
|
|
// Dispose release our toggle ref.
|
|
g_object_ref (Handle);
|
|
gdk_window_destroy(Handle);
|
|
Dispose ();
|
|
}
|
|
|
|
public void MoveResize (Gdk.Rectangle rect) {
|
|
gdk_window_move_resize (Handle, rect.X, rect.Y, rect.Width, rect.Height);
|
|
}
|
|
|
|
#if FIXME30
|
|
public void ClearArea (Gdk.Rectangle rect, bool expose) {
|
|
if (expose)
|
|
gdk_window_clear_area_e (Handle, rect.X, rect.Y, rect.Width, rect.Height);
|
|
else
|
|
gdk_window_clear_area (Handle, rect.X, rect.Y, rect.Width, rect.Height);
|
|
}
|
|
#endif
|
|
|
|
[DllImport ("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern void gdk_window_get_user_data (IntPtr raw, out IntPtr data);
|
|
|
|
[DllImport ("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern void gdk_window_set_user_data(IntPtr raw, IntPtr user_data);
|
|
public IntPtr UserData {
|
|
get {
|
|
IntPtr data;
|
|
gdk_window_get_user_data (Handle, out data);
|
|
return data;
|
|
}
|
|
set {
|
|
gdk_window_set_user_data(Handle, value);
|
|
}
|
|
}
|
|
|
|
[DllImport ("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern void gdk_window_add_filter (IntPtr handle, GdkSharp.FilterFuncNative wrapper, IntPtr data);
|
|
|
|
[DllImport ("libgdk-win32-3.0-0.dll", CallingConvention = CallingConvention.Cdecl)]
|
|
static extern void gdk_window_remove_filter (IntPtr handle, GdkSharp.FilterFuncNative wrapper, IntPtr data);
|
|
|
|
static Hashtable filter_all_hash;
|
|
static Hashtable FilterAllHash {
|
|
get {
|
|
if (filter_all_hash == null)
|
|
filter_all_hash = new Hashtable ();
|
|
return filter_all_hash;
|
|
}
|
|
}
|
|
|
|
public static void AddFilterForAll (FilterFunc func)
|
|
{
|
|
GdkSharp.FilterFuncWrapper wrapper = new GdkSharp.FilterFuncWrapper (func);
|
|
FilterAllHash [func] = wrapper;
|
|
gdk_window_add_filter (IntPtr.Zero, wrapper.NativeDelegate, IntPtr.Zero);
|
|
}
|
|
|
|
public static void RemoveFilterForAll (FilterFunc func)
|
|
{
|
|
GdkSharp.FilterFuncWrapper wrapper = FilterAllHash [func] as GdkSharp.FilterFuncWrapper;
|
|
if (wrapper == null)
|
|
return;
|
|
FilterAllHash.Remove (func);
|
|
gdk_window_remove_filter (IntPtr.Zero, wrapper.NativeDelegate, IntPtr.Zero);
|
|
}
|
|
|
|
public void AddFilter (FilterFunc function)
|
|
{
|
|
if (!Data.Contains ("filter_func_hash"))
|
|
Data ["filter_func_hash"] = new Hashtable ();
|
|
Hashtable hash = Data ["filter_func_hash"] as Hashtable;
|
|
GdkSharp.FilterFuncWrapper wrapper = new GdkSharp.FilterFuncWrapper (function);
|
|
hash [function] = wrapper;
|
|
gdk_window_add_filter (Handle, wrapper.NativeDelegate, IntPtr.Zero);
|
|
}
|
|
|
|
public void RemoveFilter (FilterFunc function)
|
|
{
|
|
Hashtable hash = Data ["filter_func_hash"] as Hashtable;
|
|
GdkSharp.FilterFuncWrapper wrapper = hash [function] as GdkSharp.FilterFuncWrapper;
|
|
if (wrapper == null)
|
|
return;
|
|
hash.Remove (function);
|
|
gdk_window_remove_filter (Handle, wrapper.NativeDelegate, IntPtr.Zero);
|
|
}
|
|
|
|
#if MANLY_ENOUGH_TO_INCLUDE
|
|
public Cairo.Graphics CairoGraphics (out int offset_x, out int offset_y)
|
|
{
|
|
IntPtr real_drawable;
|
|
Cairo.Graphics o = new Cairo.Graphics ();
|
|
|
|
gdk_window_get_internal_paint_info (Handle, out real_drawable, out offset_x, out offset_y);
|
|
IntPtr x11 = gdk_x11_drawable_get_xid (real_drawable);
|
|
IntPtr display = gdk_x11_drawable_get_xdisplay (real_drawable);
|
|
o.SetTargetDrawable (display, x11);
|
|
|
|
return o;
|
|
}
|
|
|
|
|
|
public override Cairo.Graphics CairoGraphics ()
|
|
{
|
|
int x, y;
|
|
return CairoGraphics (out x, out y);
|
|
}
|
|
#endif
|