mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-09-09 00:13:20 +02:00
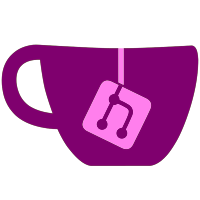
* generator/BoxedGen.cs : s/uint/GLib.GType * generator/ManualGen.cs : add a ctor to pass ToNative handle name * generator/ObjectGen.cs : s/uint/GLib.GType * generator/Signal.cs : use GLib.GType and call OverrideVirtualMethod * generator/SymbolTable.cs : make GType a ManualGen and update a few ManualGens to the new signatures. * glib/DefaultSignalHandler.cs : s/Type/System.Type * glib/ManagedValue.cs : s/uint/GLib.GType * glib/Object.cs : s/uint/GLib.GType, add OverrideVirtualMethod. * glib/Type.cs : s/uint/IntPtr, add static fields for fundamentals. make it a value type and add ==, !=, Equals, and GetHashCode. * glib/TypeConverter.cs : use new GType statics, not fundamentals. * glib/Value.cs : use new GType statics, not fundamentals. * gnome/*.custom : s/uint/GLib.GType * gtk/*Store.custom : use GType statics, not fundamentals. * sample/Subclass.cs : s/uint/GLib.GType. svn path=/trunk/gtk-sharp/; revision=21181
86 lines
2.2 KiB
C#
Executable File
86 lines
2.2 KiB
C#
Executable File
// GLib.Type.cs - GLib GType class implementation
|
|
//
|
|
// Author: Mike Kestner <mkestner@speakeasy.net>
|
|
//
|
|
// (c) 2003 Mike Kestner, Novell, Inc.
|
|
|
|
namespace GLib {
|
|
|
|
using System;
|
|
using System.Runtime.InteropServices;
|
|
|
|
/// <summary>
|
|
/// GType Class
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// An arbitrary data type similar to a CORBA Any which is used
|
|
/// to get and set properties on Objects.
|
|
/// </remarks>
|
|
|
|
[StructLayout(LayoutKind.Sequential)]
|
|
public struct GType {
|
|
|
|
IntPtr val;
|
|
|
|
/// <summary>
|
|
/// GType Constructor
|
|
/// </summary>
|
|
///
|
|
/// <remarks>
|
|
/// Constructs a new GType from a native GType value.
|
|
/// </remarks>
|
|
|
|
public GType (IntPtr val) {
|
|
this.val = val;
|
|
}
|
|
|
|
public static readonly GType Invalid = new GType ((IntPtr) TypeFundamentals.TypeInvalid);
|
|
public static readonly GType None = new GType ((IntPtr) TypeFundamentals.TypeNone);
|
|
public static readonly GType String = new GType ((IntPtr) TypeFundamentals.TypeString);
|
|
public static readonly GType Boolean = new GType ((IntPtr) TypeFundamentals.TypeBoolean);
|
|
public static readonly GType Int = new GType ((IntPtr) TypeFundamentals.TypeInt);
|
|
public static readonly GType Double = new GType ((IntPtr) TypeFundamentals.TypeDouble);
|
|
public static readonly GType Float = new GType ((IntPtr) TypeFundamentals.TypeFloat);
|
|
public static readonly GType Char = new GType ((IntPtr) TypeFundamentals.TypeChar);
|
|
public static readonly GType UInt = new GType ((IntPtr) TypeFundamentals.TypeUInt);
|
|
public static readonly GType Object = new GType ((IntPtr) TypeFundamentals.TypeObject);
|
|
public static readonly GType Pointer = new GType ((IntPtr) TypeFundamentals.TypePointer);
|
|
public static readonly GType Boxed = new GType ((IntPtr) TypeFundamentals.TypeBoxed);
|
|
|
|
public IntPtr Val {
|
|
get {
|
|
return val;
|
|
}
|
|
}
|
|
|
|
public override bool Equals (object o)
|
|
{
|
|
if (!(o is GType))
|
|
return false;
|
|
|
|
return ((GType) o) == this;
|
|
}
|
|
|
|
public static bool operator == (GType a, GType b)
|
|
{
|
|
return a.Val == b.Val;
|
|
}
|
|
|
|
public static bool operator != (GType a, GType b)
|
|
{
|
|
return a.Val != b.Val;
|
|
}
|
|
|
|
public override int GetHashCode ()
|
|
{
|
|
return val.GetHashCode ();
|
|
}
|
|
|
|
public override string ToString ()
|
|
{
|
|
return val.ToString();
|
|
}
|
|
}
|
|
}
|