mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-11 14:41:43 +02:00
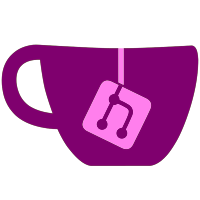
* generator/ClassBase.cs: New base class for classes and interfaces. * generator/InterfaceGen.cs: Inherit from ClassBase, generate declarations. * generator/ObjectGen.cs: Move half of this into ClassBase. * generator/Method.cs: Turn all applicable Get/Set functions into .NET accessors. Remove redundant == overload and move into Equals, as it was confusing "!= null". * generator/Parameters.cs: Alter signature creation to accept "is_set" option, add support for variable arguments. Add properties "Count", "IsVarArgs", "VAType". * generator/Ctor.cs: Fixup for changes in Parameters (indenting, signature creation). * generator/Signal.cs: Support generating declarations. * generator/SymbolTable: Change GetObjectGen to GetClassGen. * glib/IWrapper.cs: Move "Handle" declaration to here, so both classes and interfaces can benefit from it. * glib/Object.cs: Inherit from IWrapper.cs * parser/Metadata.pm: Support attribute changes on constructors, methods, signals, and paramater lists. * parser/gapi2xml.pl: Parse init funcs for interfaces. Ignore "_" functions here. * parser/gapi_pp.pl: Remove boxed_type_register check, as it will be caught in the init funcs. * parser/Atk.metadata: Added. * parser/Gtk.metadata: Add all needed signal/method collision renames. Rename GtkEditable.Editable accessors to IsEditable, as .NET does not like accessors with the same name as their declaring type. Tag TreeStore constructor as varargs. * samples/ButtonApp.cs: s/EmitAdd/Add. * samples/Menu.cs: s/EmitAdd/Add, s/Activate/Activated. svn path=/trunk/gtk-sharp/; revision=5394
58 lines
1.2 KiB
C#
Executable File
58 lines
1.2 KiB
C#
Executable File
// Menus.cs : Menu testing sample app
|
|
//
|
|
// Author: Mike Kestner <mkestner@speakeasy.net>
|
|
//
|
|
// <c> 2002 Mike Kestner
|
|
|
|
namespace GtkSharp.Samples {
|
|
|
|
using System;
|
|
using System.Drawing;
|
|
using Gtk;
|
|
using GtkSharp;
|
|
|
|
public class MenuApp {
|
|
|
|
public static void Main (string[] args)
|
|
{
|
|
Application.Init();
|
|
Window win = new Window ("Menu Sample App");
|
|
win.DeleteEvent += new EventHandler (delete_cb);
|
|
win.DefaultSize = new Size(200, 150);
|
|
|
|
VBox box = new VBox (false, 2);
|
|
|
|
MenuBar mb = new MenuBar ();
|
|
Menu file_menu = new Menu ();
|
|
MenuItem exit_item = new MenuItem("Exit");
|
|
exit_item.Activated += new EventHandler (exit_cb);
|
|
file_menu.Append (exit_item);
|
|
MenuItem file_item = new MenuItem("File");
|
|
file_item.Submenu = file_menu;
|
|
mb.Append (file_item);
|
|
box.PackStart(mb, false, false, 0);
|
|
|
|
Button btn = new Button ("Yep, that's a menu");
|
|
box.PackStart(btn, true, true, 0);
|
|
|
|
win.Add (box);
|
|
win.ShowAll ();
|
|
|
|
Application.Run ();
|
|
}
|
|
|
|
static void delete_cb (object o, EventArgs args)
|
|
{
|
|
SignalArgs sa = (SignalArgs) args;
|
|
Application.Quit ();
|
|
sa.RetVal = true;
|
|
}
|
|
|
|
static void exit_cb (object o, EventArgs args)
|
|
{
|
|
Application.Quit ();
|
|
}
|
|
}
|
|
}
|
|
|