mirror of
https://github.com/Ryujinx/GtkSharp.git
synced 2024-07-25 12:12:08 +02:00
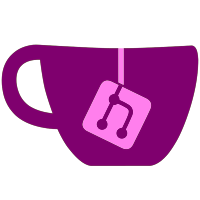
This commit makes it possible to build any project of the gtk-sharp.sln from an IDE (except audit and sample projects, which require a bit more work). This doesn't mean that autotools is deprecated, but just that it is more comfortable to use an IDE when working on gtk-sharp because it will offer better auto-completion, and will stop highlight misleading semantic errors, from now on.
79 lines
1.8 KiB
C#
79 lines
1.8 KiB
C#
// ManagedTreeViewDemo.cs - Another TreeView demo
|
|
//
|
|
// Author: Rachel Hestilow <hestilow@ximian.com>
|
|
//
|
|
// (c) 2003 Rachel Hestilow
|
|
|
|
namespace GtkSamples {
|
|
using System;
|
|
using System.Runtime.InteropServices;
|
|
|
|
using Gtk;
|
|
|
|
public class ManagedTreeViewDemo {
|
|
private static ListStore store = null;
|
|
|
|
private class Pair {
|
|
public string a, b;
|
|
public Pair (string a, string b) {
|
|
this.a = a;
|
|
this.b = b;
|
|
}
|
|
}
|
|
|
|
private static void PopulateStore ()
|
|
{
|
|
store = new ListStore (typeof (Pair));
|
|
string[] combs = {null, "foo", "bar", "baz"};
|
|
foreach (string a in combs) {
|
|
foreach (string b in combs) {
|
|
store.AppendValues (new Pair (a, b));
|
|
}
|
|
}
|
|
}
|
|
|
|
private static void CellDataA (Gtk.TreeViewColumn tree_column, Gtk.CellRenderer cell, Gtk.ITreeModel tree_model, Gtk.TreeIter iter)
|
|
{
|
|
Pair val = (Pair) store.GetValue (iter, 0);
|
|
((CellRendererText) cell).Text = val.a;
|
|
}
|
|
|
|
private static void CellDataB (Gtk.TreeViewColumn tree_column, Gtk.CellRenderer cell, Gtk.ITreeModel tree_model, Gtk.TreeIter iter)
|
|
{
|
|
Pair val = (Pair) store.GetValue (iter, 0);
|
|
((CellRendererText) cell).Text = val.b;
|
|
}
|
|
|
|
public static void Main (string[] args)
|
|
{
|
|
Application.Init ();
|
|
|
|
PopulateStore ();
|
|
|
|
Window win = new Window ("TreeView demo");
|
|
win.DeleteEvent += new DeleteEventHandler (DeleteCB);
|
|
win.DefaultWidth = 320;
|
|
win.DefaultHeight = 480;
|
|
|
|
ScrolledWindow sw = new ScrolledWindow ();
|
|
win.Add (sw);
|
|
|
|
TreeView tv = new TreeView (store);
|
|
tv.HeadersVisible = true;
|
|
|
|
tv.AppendColumn ("One", new CellRendererText (), new TreeCellDataFunc (CellDataA));
|
|
tv.AppendColumn ("Two", new CellRendererText (), new TreeCellDataFunc (CellDataB));
|
|
|
|
sw.Add (tv);
|
|
win.ShowAll ();
|
|
|
|
Application.Run ();
|
|
}
|
|
|
|
private static void DeleteCB (System.Object o, DeleteEventArgs args)
|
|
{
|
|
Application.Quit ();
|
|
}
|
|
}
|
|
}
|