mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
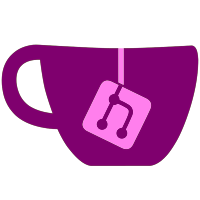
* dotnet format style --severity info Some changes were manually reverted. * dotnet format analyzers --serverity info Some changes have been minimally adapted. * Restore a few unused methods and variables * Silence dotnet format IDE0060 warnings * Silence dotnet format IDE0052 warnings * Silence dotnet format IDE0059 warnings * Address or silence dotnet format IDE1006 warnings * Address dotnet format CA1816 warnings * Address most dotnet format whitespace warnings * Run dotnet format after rebase and remove unused usings - analyzers - style - whitespace * Add comments to disabled warnings * Remove a few unused parameters * Adjust namespaces * Simplify properties and array initialization, Use const when possible, Remove trailing commas * Start working on disabled warnings * Fix and silence a few dotnet-format warnings again * Address a few disabled IDE0060 warnings * Silence IDE0060 in .editorconfig * Revert "Simplify properties and array initialization, Use const when possible, Remove trailing commas" This reverts commit 9462e4136c0a2100dc28b20cf9542e06790aa67e. * dotnet format whitespace after rebase * Address review feedback * Remove redundant unsafe modifiers * Fix build issues * Add GC.SuppressFinalize() call * Add trailing commas and fix naming rule violations * Remove unused members and assignments
42 lines
1.1 KiB
C#
42 lines
1.1 KiB
C#
using Ryujinx.Memory;
|
|
using System;
|
|
|
|
namespace Ryujinx.Cpu
|
|
{
|
|
readonly struct PrivateMemoryAllocation : IDisposable
|
|
{
|
|
private readonly PrivateMemoryAllocator _owner;
|
|
private readonly PrivateMemoryAllocator.Block _block;
|
|
|
|
public bool IsValid => _owner != null;
|
|
public MemoryBlock Memory => _block?.Memory;
|
|
public ulong Offset { get; }
|
|
public ulong Size { get; }
|
|
|
|
public PrivateMemoryAllocation(
|
|
PrivateMemoryAllocator owner,
|
|
PrivateMemoryAllocator.Block block,
|
|
ulong offset,
|
|
ulong size)
|
|
{
|
|
_owner = owner;
|
|
_block = block;
|
|
Offset = offset;
|
|
Size = size;
|
|
}
|
|
|
|
public (PrivateMemoryAllocation, PrivateMemoryAllocation) Split(ulong splitOffset)
|
|
{
|
|
PrivateMemoryAllocation left = new(_owner, _block, Offset, splitOffset);
|
|
PrivateMemoryAllocation right = new(_owner, _block, Offset + splitOffset, Size - splitOffset);
|
|
|
|
return (left, right);
|
|
}
|
|
|
|
public void Dispose()
|
|
{
|
|
_owner.Free(_block, Offset, Size);
|
|
}
|
|
}
|
|
}
|