mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
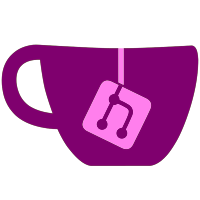
* dotnet format style --severity info Some changes were manually reverted. * dotnet format analyzers --serverity info Some changes have been minimally adapted. * Restore a few unused methods and variables * Silence dotnet format IDE0060 warnings * Address or silence dotnet format IDE1006 warnings * Fix IDE0090 after rebase * Address most dotnet format whitespace warnings * Apply dotnet format whitespace formatting A few of them have been manually reverted and the corresponding warning was silenced * Format if-blocks correctly * Another rebase, another dotnet format run * Run dotnet format after rebase and remove unused usings - analyzers - style - whitespace * Add comments to disabled warnings * Simplify properties and array initialization, Use const when possible, Remove trailing commas * Start working on disabled warnings * Address a few disabled IDE0060 warnings * Silence IDE0060 in .editorconfig * Revert "Simplify properties and array initialization, Use const when possible, Remove trailing commas" This reverts commit 9462e4136c0a2100dc28b20cf9542e06790aa67e. * dotnet format whitespace after rebase * First dotnet format pass * Address review feedback
110 lines
4.1 KiB
C#
110 lines
4.1 KiB
C#
using OpenTK.Graphics.OpenGL;
|
|
using Ryujinx.Common.Configuration;
|
|
using Ryujinx.Common.Logging;
|
|
using System;
|
|
using System.Runtime.InteropServices;
|
|
using System.Threading;
|
|
|
|
namespace Ryujinx.Graphics.OpenGL
|
|
{
|
|
public static class Debugger
|
|
{
|
|
private static DebugProc _debugCallback;
|
|
|
|
private static int _counter;
|
|
|
|
public static void Initialize(GraphicsDebugLevel logLevel)
|
|
{
|
|
// Disable everything
|
|
GL.DebugMessageControl(DebugSourceControl.DontCare, DebugTypeControl.DontCare, DebugSeverityControl.DontCare, 0, (int[])null, false);
|
|
|
|
if (logLevel == GraphicsDebugLevel.None)
|
|
{
|
|
GL.Disable(EnableCap.DebugOutputSynchronous);
|
|
GL.DebugMessageCallback(null, IntPtr.Zero);
|
|
|
|
return;
|
|
}
|
|
|
|
GL.Enable(EnableCap.DebugOutputSynchronous);
|
|
|
|
if (logLevel == GraphicsDebugLevel.Error)
|
|
{
|
|
GL.DebugMessageControl(DebugSourceControl.DontCare, DebugTypeControl.DebugTypeError, DebugSeverityControl.DontCare, 0, (int[])null, true);
|
|
}
|
|
else if (logLevel == GraphicsDebugLevel.Slowdowns)
|
|
{
|
|
GL.DebugMessageControl(DebugSourceControl.DontCare, DebugTypeControl.DebugTypeError, DebugSeverityControl.DontCare, 0, (int[])null, true);
|
|
GL.DebugMessageControl(DebugSourceControl.DontCare, DebugTypeControl.DebugTypePerformance, DebugSeverityControl.DontCare, 0, (int[])null, true);
|
|
}
|
|
else
|
|
{
|
|
GL.DebugMessageControl(DebugSourceControl.DontCare, DebugTypeControl.DontCare, DebugSeverityControl.DontCare, 0, (int[])null, true);
|
|
}
|
|
|
|
_counter = 0;
|
|
_debugCallback = GLDebugHandler;
|
|
|
|
GL.DebugMessageCallback(_debugCallback, IntPtr.Zero);
|
|
|
|
Logger.Warning?.Print(LogClass.Gpu, "OpenGL Debugging is enabled. Performance will be negatively impacted.");
|
|
}
|
|
|
|
private static void GLDebugHandler(
|
|
DebugSource source,
|
|
DebugType type,
|
|
int id,
|
|
DebugSeverity severity,
|
|
int length,
|
|
IntPtr message,
|
|
IntPtr userParam)
|
|
{
|
|
string msg = Marshal.PtrToStringUTF8(message).Replace('\n', ' ');
|
|
|
|
switch (type)
|
|
{
|
|
case DebugType.DebugTypeError:
|
|
Logger.Error?.Print(LogClass.Gpu, $"{severity}: {msg}\nCallStack={Environment.StackTrace}", "GLERROR");
|
|
break;
|
|
case DebugType.DebugTypePerformance:
|
|
Logger.Warning?.Print(LogClass.Gpu, $"{severity}: {msg}", "GLPERF");
|
|
break;
|
|
case DebugType.DebugTypePushGroup:
|
|
Logger.Info?.Print(LogClass.Gpu, $"{{ ({id}) {severity}: {msg}", "GLINFO");
|
|
break;
|
|
case DebugType.DebugTypePopGroup:
|
|
Logger.Info?.Print(LogClass.Gpu, $"}} ({id}) {severity}: {msg}", "GLINFO");
|
|
break;
|
|
default:
|
|
if (source == DebugSource.DebugSourceApplication)
|
|
{
|
|
Logger.Info?.Print(LogClass.Gpu, $"{type} {severity}: {msg}", "GLINFO");
|
|
}
|
|
else
|
|
{
|
|
Logger.Debug?.Print(LogClass.Gpu, $"{type} {severity}: {msg}", "GLDEBUG");
|
|
}
|
|
break;
|
|
}
|
|
}
|
|
|
|
// Useful debug helpers
|
|
public static void PushGroup(string dbgMsg)
|
|
{
|
|
int counter = Interlocked.Increment(ref _counter);
|
|
|
|
GL.PushDebugGroup(DebugSourceExternal.DebugSourceApplication, counter, dbgMsg.Length, dbgMsg);
|
|
}
|
|
|
|
public static void PopGroup()
|
|
{
|
|
GL.PopDebugGroup();
|
|
}
|
|
|
|
public static void Print(string dbgMsg, DebugType type = DebugType.DebugTypeMarker, DebugSeverity severity = DebugSeverity.DebugSeverityNotification, int id = 999999)
|
|
{
|
|
GL.DebugMessageInsert(DebugSourceExternal.DebugSourceApplication, type, id, severity, dbgMsg.Length, dbgMsg);
|
|
}
|
|
}
|
|
}
|