mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
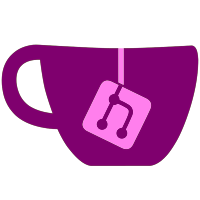
* Implement vertex and geometry shader conversion to compute * Call InitializeReservedCounts for compute too * PR feedback * Set clip distance mask for geometry and tessellation shaders too * Transform feedback emulation only for vertex
47 lines
1.5 KiB
C#
47 lines
1.5 KiB
C#
using Ryujinx.Graphics.Shader.Translation;
|
|
using System;
|
|
|
|
namespace Ryujinx.Graphics.Shader
|
|
{
|
|
public enum AttributeType : byte
|
|
{
|
|
// Generic types.
|
|
Float,
|
|
Sint,
|
|
Uint,
|
|
Sscaled,
|
|
Uscaled,
|
|
|
|
Packed = 1 << 6,
|
|
PackedRgb10A2Signed = 1 << 7,
|
|
AnyPacked = Packed | PackedRgb10A2Signed,
|
|
}
|
|
|
|
static class AttributeTypeExtensions
|
|
{
|
|
public static AggregateType ToAggregateType(this AttributeType type)
|
|
{
|
|
return (type & ~AttributeType.AnyPacked) switch
|
|
{
|
|
AttributeType.Float => AggregateType.FP32,
|
|
AttributeType.Sint => AggregateType.S32,
|
|
AttributeType.Uint => AggregateType.U32,
|
|
_ => throw new ArgumentException($"Invalid attribute type \"{type}\"."),
|
|
};
|
|
}
|
|
|
|
public static AggregateType ToAggregateType(this AttributeType type, bool supportsScaledFormats)
|
|
{
|
|
return (type & ~AttributeType.AnyPacked) switch
|
|
{
|
|
AttributeType.Float => AggregateType.FP32,
|
|
AttributeType.Sint => AggregateType.S32,
|
|
AttributeType.Uint => AggregateType.U32,
|
|
AttributeType.Sscaled => supportsScaledFormats ? AggregateType.FP32 : AggregateType.S32,
|
|
AttributeType.Uscaled => supportsScaledFormats ? AggregateType.FP32 : AggregateType.U32,
|
|
_ => throw new ArgumentException($"Invalid attribute type \"{type}\"."),
|
|
};
|
|
}
|
|
}
|
|
}
|