mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
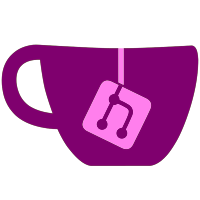
* dotnet format style --severity info Some changes were manually reverted. * dotnet format analyzers --serverity info Some changes have been minimally adapted. * Restore a few unused methods and variables * Silence dotnet format IDE0060 warnings * Silence dotnet format IDE0059 warnings * Address dotnet format CA1816 warnings * Fix new dotnet-format issues after rebase * Address most dotnet format whitespace warnings * Apply dotnet format whitespace formatting A few of them have been manually reverted and the corresponding warning was silenced * Format if-blocks correctly * Another rebase, another dotnet format run * Run dotnet format whitespace after rebase * Run dotnet format style after rebase * Run dotnet format analyzers after rebase * Run dotnet format style after rebase * Run dotnet format after rebase and remove unused usings - analyzers - style - whitespace * Disable 'prefer switch expression' rule * Add comments to disabled warnings * Simplify properties and array initialization, Use const when possible, Remove trailing commas * Run dotnet format after rebase * Address IDE0251 warnings * Address a few disabled IDE0060 warnings * Silence IDE0060 in .editorconfig * Revert "Simplify properties and array initialization, Use const when possible, Remove trailing commas" This reverts commit 9462e4136c0a2100dc28b20cf9542e06790aa67e. * dotnet format whitespace after rebase * First dotnet format pass * Fix naming rule violations * Remove redundant code * Rename generics * Address review feedback * Remove SetOrigin
122 lines
2.6 KiB
C#
122 lines
2.6 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
|
|
namespace Ryujinx.Graphics.Vulkan
|
|
{
|
|
class IdList<T> where T : class
|
|
{
|
|
private readonly List<T> _list;
|
|
private int _freeMin;
|
|
|
|
public IdList()
|
|
{
|
|
_list = new List<T>();
|
|
_freeMin = 0;
|
|
}
|
|
|
|
public int Add(T value)
|
|
{
|
|
int id;
|
|
int count = _list.Count;
|
|
id = _list.IndexOf(null, _freeMin);
|
|
|
|
if ((uint)id < (uint)count)
|
|
{
|
|
_list[id] = value;
|
|
}
|
|
else
|
|
{
|
|
id = count;
|
|
_freeMin = id + 1;
|
|
|
|
_list.Add(value);
|
|
}
|
|
|
|
return id + 1;
|
|
}
|
|
|
|
public void Remove(int id)
|
|
{
|
|
id--;
|
|
|
|
int count = _list.Count;
|
|
|
|
if ((uint)id >= (uint)count)
|
|
{
|
|
return;
|
|
}
|
|
|
|
if (id + 1 == count)
|
|
{
|
|
// Trim unused items.
|
|
int removeIndex = id;
|
|
|
|
while (removeIndex > 0 && _list[removeIndex - 1] == null)
|
|
{
|
|
removeIndex--;
|
|
}
|
|
|
|
_list.RemoveRange(removeIndex, count - removeIndex);
|
|
|
|
if (_freeMin > removeIndex)
|
|
{
|
|
_freeMin = removeIndex;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
_list[id] = null;
|
|
|
|
if (_freeMin > id)
|
|
{
|
|
_freeMin = id;
|
|
}
|
|
}
|
|
}
|
|
|
|
public bool TryGetValue(int id, out T value)
|
|
{
|
|
id--;
|
|
|
|
try
|
|
{
|
|
if ((uint)id < (uint)_list.Count)
|
|
{
|
|
value = _list[id];
|
|
return value != null;
|
|
}
|
|
|
|
value = null;
|
|
return false;
|
|
}
|
|
catch (ArgumentOutOfRangeException)
|
|
{
|
|
value = null;
|
|
return false;
|
|
}
|
|
catch (IndexOutOfRangeException)
|
|
{
|
|
value = null;
|
|
return false;
|
|
}
|
|
}
|
|
|
|
public void Clear()
|
|
{
|
|
_list.Clear();
|
|
_freeMin = 0;
|
|
}
|
|
|
|
public IEnumerator<T> GetEnumerator()
|
|
{
|
|
for (int i = 0; i < _list.Count; i++)
|
|
{
|
|
if (_list[i] != null)
|
|
{
|
|
yield return _list[i];
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|