mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
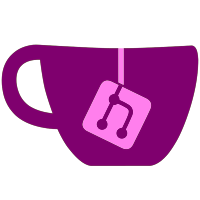
* dotnet format style --severity info Some changes were manually reverted. * dotnet format analyzers --serverity info Some changes have been minimally adapted. * Restore a few unused methods and variables * Silence dotnet format IDE0060 warnings * Silence dotnet format IDE0059 warnings * Address dotnet format CA1816 warnings * Fix new dotnet-format issues after rebase * Address most dotnet format whitespace warnings * Apply dotnet format whitespace formatting A few of them have been manually reverted and the corresponding warning was silenced * Format if-blocks correctly * Another rebase, another dotnet format run * Run dotnet format whitespace after rebase * Run dotnet format style after rebase * Run dotnet format analyzers after rebase * Run dotnet format style after rebase * Run dotnet format after rebase and remove unused usings - analyzers - style - whitespace * Disable 'prefer switch expression' rule * Add comments to disabled warnings * Simplify properties and array initialization, Use const when possible, Remove trailing commas * Run dotnet format after rebase * Address IDE0251 warnings * Address a few disabled IDE0060 warnings * Silence IDE0060 in .editorconfig * Revert "Simplify properties and array initialization, Use const when possible, Remove trailing commas" This reverts commit 9462e4136c0a2100dc28b20cf9542e06790aa67e. * dotnet format whitespace after rebase * First dotnet format pass * Fix naming rule violations * Remove redundant code * Rename generics * Address review feedback * Remove SetOrigin
108 lines
3.1 KiB
C#
108 lines
3.1 KiB
C#
using Ryujinx.Graphics.GAL;
|
|
using Silk.NET.Vulkan;
|
|
using System;
|
|
using System.Collections.Concurrent;
|
|
using System.Collections.ObjectModel;
|
|
|
|
namespace Ryujinx.Graphics.Vulkan
|
|
{
|
|
class PipelineLayoutCache
|
|
{
|
|
private readonly struct PlceKey : IEquatable<PlceKey>
|
|
{
|
|
public readonly ReadOnlyCollection<ResourceDescriptorCollection> SetDescriptors;
|
|
public readonly bool UsePushDescriptors;
|
|
|
|
public PlceKey(ReadOnlyCollection<ResourceDescriptorCollection> setDescriptors, bool usePushDescriptors)
|
|
{
|
|
SetDescriptors = setDescriptors;
|
|
UsePushDescriptors = usePushDescriptors;
|
|
}
|
|
|
|
public override int GetHashCode()
|
|
{
|
|
HashCode hasher = new();
|
|
|
|
if (SetDescriptors != null)
|
|
{
|
|
foreach (var setDescriptor in SetDescriptors)
|
|
{
|
|
hasher.Add(setDescriptor);
|
|
}
|
|
}
|
|
|
|
hasher.Add(UsePushDescriptors);
|
|
|
|
return hasher.ToHashCode();
|
|
}
|
|
|
|
public override bool Equals(object obj)
|
|
{
|
|
return obj is PlceKey other && Equals(other);
|
|
}
|
|
|
|
public bool Equals(PlceKey other)
|
|
{
|
|
if ((SetDescriptors == null) != (other.SetDescriptors == null))
|
|
{
|
|
return false;
|
|
}
|
|
|
|
if (SetDescriptors != null)
|
|
{
|
|
if (SetDescriptors.Count != other.SetDescriptors.Count)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
for (int index = 0; index < SetDescriptors.Count; index++)
|
|
{
|
|
if (!SetDescriptors[index].Equals(other.SetDescriptors[index]))
|
|
{
|
|
return false;
|
|
}
|
|
}
|
|
}
|
|
|
|
return UsePushDescriptors == other.UsePushDescriptors;
|
|
}
|
|
}
|
|
|
|
private readonly ConcurrentDictionary<PlceKey, PipelineLayoutCacheEntry> _plces;
|
|
|
|
public PipelineLayoutCache()
|
|
{
|
|
_plces = new ConcurrentDictionary<PlceKey, PipelineLayoutCacheEntry>();
|
|
}
|
|
|
|
public PipelineLayoutCacheEntry GetOrCreate(
|
|
VulkanRenderer gd,
|
|
Device device,
|
|
ReadOnlyCollection<ResourceDescriptorCollection> setDescriptors,
|
|
bool usePushDescriptors)
|
|
{
|
|
var key = new PlceKey(setDescriptors, usePushDescriptors);
|
|
|
|
return _plces.GetOrAdd(key, newKey => new PipelineLayoutCacheEntry(gd, device, setDescriptors, usePushDescriptors));
|
|
}
|
|
|
|
protected virtual void Dispose(bool disposing)
|
|
{
|
|
if (disposing)
|
|
{
|
|
foreach (var plce in _plces.Values)
|
|
{
|
|
plce.Dispose();
|
|
}
|
|
|
|
_plces.Clear();
|
|
}
|
|
}
|
|
|
|
public void Dispose()
|
|
{
|
|
Dispose(true);
|
|
}
|
|
}
|
|
}
|