mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
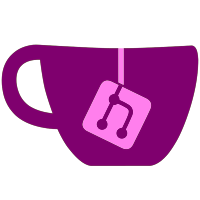
* Vulkan: Add Render Pass / Framebuffer Cache Cache is owned by each texture view. - Window's way of getting framebuffer cache for swapchain images is really messy - it creates a TextureView out of just a vk image view, with invalid info and no storage. * Clear up limited use of alternate TextureView constructor * Formatting and messages * More formatting and messages I apologize for `_colorsCanonical[index]?.Storage?.InsertReadToWriteBarrier`, the compiler made me do it * Self review, change GetFramebuffer to GetPassAndFramebuffer * Avoid allocations on Remove for HashTableSlim * Member can be readonly * Generate texture create info for swapchain images * Improve hashcode * Remove format, samples, size and isDepthStencil when possible Tested in a number of games, seems fine. * Removed load op barriers These can be introduced later. * Reintroduce UpdateModifications Technically meant to be replaced by load op stuff.
44 lines
1.1 KiB
C#
44 lines
1.1 KiB
C#
using System;
|
|
using System.Linq;
|
|
|
|
namespace Ryujinx.Graphics.Vulkan
|
|
{
|
|
internal readonly struct RenderPassCacheKey : IRefEquatable<RenderPassCacheKey>
|
|
{
|
|
private readonly TextureView _depthStencil;
|
|
private readonly TextureView[] _colors;
|
|
|
|
public RenderPassCacheKey(TextureView depthStencil, TextureView[] colors)
|
|
{
|
|
_depthStencil = depthStencil;
|
|
_colors = colors;
|
|
}
|
|
|
|
public override int GetHashCode()
|
|
{
|
|
HashCode hc = new();
|
|
|
|
hc.Add(_depthStencil);
|
|
|
|
if (_colors != null)
|
|
{
|
|
foreach (var color in _colors)
|
|
{
|
|
hc.Add(color);
|
|
}
|
|
}
|
|
|
|
return hc.ToHashCode();
|
|
}
|
|
|
|
public bool Equals(ref RenderPassCacheKey other)
|
|
{
|
|
bool colorsNull = _colors == null;
|
|
bool otherNull = other._colors == null;
|
|
return other._depthStencil == _depthStencil &&
|
|
colorsNull == otherNull &&
|
|
(colorsNull || other._colors.SequenceEqual(_colors));
|
|
}
|
|
}
|
|
}
|