mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
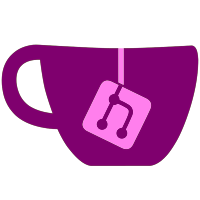
* dotnet format style --severity info Some changes were manually reverted. * dotnet format analyzers --serverity info Some changes have been minimally adapted. * Restore a few unused methods and variables * Silence dotnet format IDE0060 warnings * Silence dotnet format IDE0059 warnings * Address dotnet format CA1816 warnings * Fix new dotnet-format issues after rebase * Address most dotnet format whitespace warnings * Apply dotnet format whitespace formatting A few of them have been manually reverted and the corresponding warning was silenced * Format if-blocks correctly * Another rebase, another dotnet format run * Run dotnet format whitespace after rebase * Run dotnet format style after rebase * Run dotnet format analyzers after rebase * Run dotnet format style after rebase * Run dotnet format after rebase and remove unused usings - analyzers - style - whitespace * Disable 'prefer switch expression' rule * Add comments to disabled warnings * Simplify properties and array initialization, Use const when possible, Remove trailing commas * Run dotnet format after rebase * Address IDE0251 warnings * Address a few disabled IDE0060 warnings * Silence IDE0060 in .editorconfig * Revert "Simplify properties and array initialization, Use const when possible, Remove trailing commas" This reverts commit 9462e4136c0a2100dc28b20cf9542e06790aa67e. * dotnet format whitespace after rebase * First dotnet format pass * Fix naming rule violations * Remove redundant code * Rename generics * Address review feedback * Remove SetOrigin
101 lines
2.8 KiB
C#
101 lines
2.8 KiB
C#
using Silk.NET.Vulkan;
|
|
using System;
|
|
|
|
namespace Ryujinx.Graphics.Vulkan
|
|
{
|
|
public enum SpecConstType
|
|
{
|
|
Bool32,
|
|
Int16,
|
|
Int32,
|
|
Int64,
|
|
Float16,
|
|
Float32,
|
|
Float64,
|
|
}
|
|
|
|
sealed class SpecDescription
|
|
{
|
|
public readonly SpecializationInfo Info;
|
|
public readonly SpecializationMapEntry[] Map;
|
|
|
|
// For mapping a simple packed struct or single entry
|
|
public SpecDescription(params (uint Id, SpecConstType Type)[] description)
|
|
{
|
|
int count = description.Length;
|
|
Map = new SpecializationMapEntry[count];
|
|
|
|
uint structSize = 0;
|
|
|
|
for (int i = 0; i < Map.Length; ++i)
|
|
{
|
|
var typeSize = SizeOf(description[i].Type);
|
|
Map[i] = new SpecializationMapEntry(description[i].Id, structSize, typeSize);
|
|
structSize += typeSize;
|
|
}
|
|
|
|
Info = new SpecializationInfo
|
|
{
|
|
DataSize = structSize,
|
|
MapEntryCount = (uint)count,
|
|
};
|
|
}
|
|
|
|
// For advanced mapping with overlapping or staggered fields
|
|
public SpecDescription(SpecializationMapEntry[] map)
|
|
{
|
|
Map = map;
|
|
|
|
uint structSize = 0;
|
|
for (int i = 0; i < map.Length; ++i)
|
|
{
|
|
structSize = Math.Max(structSize, map[i].Offset + (uint)map[i].Size);
|
|
}
|
|
|
|
Info = new SpecializationInfo
|
|
{
|
|
DataSize = structSize,
|
|
MapEntryCount = (uint)map.Length,
|
|
};
|
|
}
|
|
|
|
private static uint SizeOf(SpecConstType type) => type switch
|
|
{
|
|
SpecConstType.Int16 or SpecConstType.Float16 => 2,
|
|
SpecConstType.Bool32 or SpecConstType.Int32 or SpecConstType.Float32 => 4,
|
|
SpecConstType.Int64 or SpecConstType.Float64 => 8,
|
|
_ => throw new ArgumentOutOfRangeException(nameof(type)),
|
|
};
|
|
|
|
private SpecDescription()
|
|
{
|
|
Info = new();
|
|
}
|
|
|
|
public static readonly SpecDescription Empty = new();
|
|
}
|
|
|
|
readonly struct SpecData : IRefEquatable<SpecData>
|
|
{
|
|
private readonly byte[] _data;
|
|
private readonly int _hash;
|
|
|
|
public int Length => _data.Length;
|
|
public ReadOnlySpan<byte> Span => _data.AsSpan();
|
|
public override int GetHashCode() => _hash;
|
|
|
|
public SpecData(ReadOnlySpan<byte> data)
|
|
{
|
|
_data = new byte[data.Length];
|
|
data.CopyTo(_data);
|
|
|
|
var hc = new HashCode();
|
|
hc.AddBytes(data);
|
|
_hash = hc.ToHashCode();
|
|
}
|
|
|
|
public override bool Equals(object obj) => obj is SpecData other && Equals(other);
|
|
public bool Equals(ref SpecData other) => _data.AsSpan().SequenceEqual(other._data);
|
|
}
|
|
}
|