mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
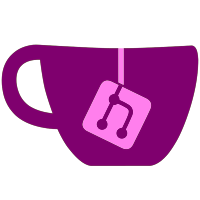
* Add workflow to perform automated checks for PRs * Downgrade Microsoft.CodeAnalysis to 4.4.0 This is a workaround to fix issues with dotnet-format. See: - https://github.com/dotnet/format/issues/1805 - https://github.com/dotnet/format/issues/1800 * Adjust editorconfig to be more compatible with Ryujinx code-style * Adjust .editorconfig line endings to match .gitattributes * Disable 'prefer switch expression' rule * Remove naming styles These are the default rules, so we don't need to override them. * Silence IDE0060 in .editorconfig * Slightly adjust .editorconfig * Add lost workflow changes * Move .editorconfig comment to the top * .editorconfig: private static readonly fields should be _lowerCamelCase * .editorconfig: Remove alignment for declarations as well * editorconfig: Add rule for local constants * Disable CA1822 for HLE services * Disable CA1822 for ViewModels Bindings won't work with static members, but this issue is silently ignored. * Run dotnet format for the whole solution * Check result code of SDL_GetDisplayBounds * Fix dotnet format style issues * Add missing trailing commas * Update Microsoft.CodeAnalysis.CSharp to 4.6.0 Skipping 4.5.0 since it breaks dotnet format * Restore old default naming rules for dotnet format * Add naming rule exception for CPU tests * checks: Include all files before excluding paths * Fix dotnet format issues * Check dotnet format version * checks: Run dotnet format with severity info again * checks: Disable naming style rules until they won't crash the process anymore * Remove unread private member * checks: Attempt to run analyzers 3 times before giving up * checks: Enable naming style rules again with the new retry logic
1702 lines
94 KiB
C#
1702 lines
94 KiB
C#
using System.Collections.Generic;
|
|
|
|
namespace Ryujinx.Horizon.Common
|
|
{
|
|
static class ResultNames
|
|
{
|
|
// Reference: https://github.com/Thealexbarney/LibHac/blob/master/build/CodeGen/results.csv
|
|
private static readonly IReadOnlyDictionary<int, string> _names = new Dictionary<int, string>()
|
|
{
|
|
{ 0x0, "Success" },
|
|
{ 0xE01, "OutOfSessions" },
|
|
{ 0x1C01, "InvalidArgument" },
|
|
{ 0x4201, "NotImplemented" },
|
|
{ 0x6C01, "StopProcessingException" },
|
|
{ 0x7201, "NoSynchronizationObject" },
|
|
{ 0x7601, "TerminationRequested" },
|
|
{ 0x8C01, "NoEvent" },
|
|
{ 0xCA01, "InvalidSize" },
|
|
{ 0xCC01, "InvalidAddress" },
|
|
{ 0xCE01, "OutOfResource" },
|
|
{ 0xD001, "OutOfMemory" },
|
|
{ 0xD201, "OutOfHandles" },
|
|
{ 0xD401, "InvalidCurrentMemory" },
|
|
{ 0xD801, "InvalidNewMemoryPermission" },
|
|
{ 0xDC01, "InvalidMemoryRegion" },
|
|
{ 0xE001, "InvalidPriority" },
|
|
{ 0xE201, "InvalidCoreId" },
|
|
{ 0xE401, "InvalidHandle" },
|
|
{ 0xE601, "InvalidPointer" },
|
|
{ 0xE801, "InvalidCombination" },
|
|
{ 0xEA01, "TimedOut" },
|
|
{ 0xEC01, "Cancelled" },
|
|
{ 0xEE01, "OutOfRange" },
|
|
{ 0xF001, "InvalidEnumValue" },
|
|
{ 0xF201, "NotFound" },
|
|
{ 0xF401, "Busy" },
|
|
{ 0xF601, "SessionClosed" },
|
|
{ 0xF801, "NotHandled" },
|
|
{ 0xFA01, "InvalidState" },
|
|
{ 0xFC01, "ReservedUsed" },
|
|
{ 0xFE01, "NotSupported" },
|
|
{ 0x10001, "Debug" },
|
|
{ 0x10201, "NoThread" },
|
|
{ 0x10401, "UnknownThread" },
|
|
{ 0x10601, "PortClosed" },
|
|
{ 0x10801, "LimitReached" },
|
|
{ 0x10A01, "InvalidMemoryPool" },
|
|
{ 0x20401, "ReceiveListBroken" },
|
|
{ 0x20601, "OutOfAddressSpace" },
|
|
{ 0x20801, "MessageTooLarge" },
|
|
{ 0x40A01, "InvalidProcessId" },
|
|
{ 0x40C01, "InvalidThreadId" },
|
|
{ 0x40E01, "InvalidId" },
|
|
{ 0x41001, "ProcessTerminated" },
|
|
{ 0x2, "HandledByAllProcess" },
|
|
{ 0x202, "PathNotFound" },
|
|
{ 0x402, "PathAlreadyExists" },
|
|
{ 0x1002, "DirectoryNotEmpty" },
|
|
{ 0x1A02, "DirectoryStatusChanged" },
|
|
{ 0x3C02, "UsableSpaceNotEnough" },
|
|
{ 0x3E02, "UsableSpaceNotEnoughForSaveData" },
|
|
{ 0x4002, "UsableSpaceNotEnoughForSaveDataEvenAssistanceSuccess" },
|
|
{ 0x4202, "UsableSpaceNotEnoughForCacheStorage" },
|
|
{ 0x4402, "UsableSpaceNotEnoughMmc" },
|
|
{ 0x4602, "UsableSpaceNotEnoughMmcCalibration" },
|
|
{ 0x4802, "UsableSpaceNotEnoughMmcSafe" },
|
|
{ 0x4A02, "UsableSpaceNotEnoughMmcUser" },
|
|
{ 0x4C02, "UsableSpaceNotEnoughMmcSystem" },
|
|
{ 0x4E02, "UsableSpaceNotEnoughSdCard" },
|
|
{ 0x6402, "UnsupportedSdkVersion" },
|
|
{ 0x7802, "MountNameAlreadyExists" },
|
|
{ 0x8C02, "IndividualFileDataCacheAlreadyEnabled" },
|
|
{ 0x7D002, "HandledBySystemProcess" },
|
|
{ 0x7D202, "PartitionNotFound" },
|
|
{ 0x7D402, "TargetNotFound" },
|
|
{ 0x7D602, "HasNotGottenPatrolCount" },
|
|
{ 0x7D802, "NcaExternalKeyUnregistered" },
|
|
{ 0xFA002, "SdCardAccessFailed" },
|
|
{ 0xFA202, "PortSdCardNoDevice" },
|
|
{ 0xFA402, "PortSdCardNotActivated" },
|
|
{ 0xFA602, "PortSdCardDeviceRemoved" },
|
|
{ 0xFA802, "PortSdCardNotAwakened" },
|
|
{ 0xFE002, "PortSdCardCommunicationError" },
|
|
{ 0xFE202, "PortSdCardCommunicationNotAttained" },
|
|
{ 0xFE402, "PortSdCardResponseIndexError" },
|
|
{ 0xFE602, "PortSdCardResponseEndBitError" },
|
|
{ 0xFE802, "PortSdCardResponseCrcError" },
|
|
{ 0xFEA02, "PortSdCardResponseTimeoutError" },
|
|
{ 0xFEC02, "PortSdCardDataEndBitError" },
|
|
{ 0xFEE02, "PortSdCardDataCrcError" },
|
|
{ 0xFF002, "PortSdCardDataTimeoutError" },
|
|
{ 0xFF202, "PortSdCardAutoCommandResponseIndexError" },
|
|
{ 0xFF402, "PortSdCardAutoCommandResponseEndBitError" },
|
|
{ 0xFF602, "PortSdCardAutoCommandResponseCrcError" },
|
|
{ 0xFF802, "PortSdCardAutoCommandResponseTimeoutError" },
|
|
{ 0xFFA02, "PortSdCardCommandCompleteSwTimeout" },
|
|
{ 0xFFC02, "PortSdCardTransferCompleteSwTimeout" },
|
|
{ 0x100002, "PortSdCardDeviceStatusHasError" },
|
|
{ 0x100202, "PortSdCardDeviceStatusAddressOutOfRange" },
|
|
{ 0x100402, "PortSdCardDeviceStatusAddressMisalign" },
|
|
{ 0x100602, "PortSdCardDeviceStatusBlockLenError" },
|
|
{ 0x100802, "PortSdCardDeviceStatusEraseSeqError" },
|
|
{ 0x100A02, "PortSdCardDeviceStatusEraseParam" },
|
|
{ 0x100C02, "PortSdCardDeviceStatusWpViolation" },
|
|
{ 0x100E02, "PortSdCardDeviceStatusLockUnlockFailed" },
|
|
{ 0x101002, "PortSdCardDeviceStatusComCrcError" },
|
|
{ 0x101202, "PortSdCardDeviceStatusIllegalCommand" },
|
|
{ 0x101402, "PortSdCardDeviceStatusDeviceEccFailed" },
|
|
{ 0x101602, "PortSdCardDeviceStatusCcError" },
|
|
{ 0x101802, "PortSdCardDeviceStatusError" },
|
|
{ 0x101A02, "PortSdCardDeviceStatusCidCsdOverwrite" },
|
|
{ 0x101C02, "PortSdCardDeviceStatusWpEraseSkip" },
|
|
{ 0x101E02, "PortSdCardDeviceStatusEraseReset" },
|
|
{ 0x102002, "PortSdCardDeviceStatusSwitchError" },
|
|
{ 0x103002, "PortSdCardUnexpectedDeviceState" },
|
|
{ 0x103202, "PortSdCardUnexpectedDeviceCsdValue" },
|
|
{ 0x103402, "PortSdCardAbortTransactionSwTimeout" },
|
|
{ 0x103602, "PortSdCardCommandInhibitCmdSwTimeout" },
|
|
{ 0x103802, "PortSdCardCommandInhibitDatSwTimeout" },
|
|
{ 0x103A02, "PortSdCardBusySwTimeout" },
|
|
{ 0x103C02, "PortSdCardIssueTuningCommandSwTimeout" },
|
|
{ 0x103E02, "PortSdCardTuningFailed" },
|
|
{ 0x104002, "PortSdCardMmcInitializationSwTimeout" },
|
|
{ 0x104202, "PortSdCardMmcNotSupportExtendedCsd" },
|
|
{ 0x104402, "PortSdCardUnexpectedMmcExtendedCsdValue" },
|
|
{ 0x104602, "PortSdCardMmcEraseSwTimeout" },
|
|
{ 0x104802, "PortSdCardSdCardValidationError" },
|
|
{ 0x104A02, "PortSdCardSdCardInitializationSwTimeout" },
|
|
{ 0x104C02, "PortSdCardSdCardGetValidRcaSwTimeout" },
|
|
{ 0x104E02, "PortSdCardUnexpectedSdCardAcmdDisabled" },
|
|
{ 0x105002, "PortSdCardSdCardNotSupportSwitchFunctionStatus" },
|
|
{ 0x105202, "PortSdCardUnexpectedSdCardSwitchFunctionStatus" },
|
|
{ 0x105402, "PortSdCardSdCardNotSupportAccessMode" },
|
|
{ 0x105602, "PortSdCardSdCardNot4BitBusWidthAtUhsIMode" },
|
|
{ 0x105802, "PortSdCardSdCardNotSupportSdr104AndSdr50" },
|
|
{ 0x105A02, "PortSdCardSdCardCannotSwitchedAccessMode" },
|
|
{ 0x105C02, "PortSdCardSdCardFailedSwitchedAccessMode" },
|
|
{ 0x105E02, "PortSdCardSdCardUnacceptableCurrentConsumption" },
|
|
{ 0x106002, "PortSdCardSdCardNotReadyToVoltageSwitch" },
|
|
{ 0x106202, "PortSdCardSdCardNotCompleteVoltageSwitch" },
|
|
{ 0x10A002, "PortSdCardHostControllerUnexpected" },
|
|
{ 0x10A202, "PortSdCardInternalClockStableSwTimeout" },
|
|
{ 0x10A402, "PortSdCardSdHostStandardUnknownAutoCmdError" },
|
|
{ 0x10A602, "PortSdCardSdHostStandardUnknownError" },
|
|
{ 0x10A802, "PortSdCardSdmmcDllCalibrationSwTimeout" },
|
|
{ 0x10AA02, "PortSdCardSdmmcDllApplicationSwTimeout" },
|
|
{ 0x10AC02, "PortSdCardSdHostStandardFailSwitchTo18V" },
|
|
{ 0x10E002, "PortSdCardInternalError" },
|
|
{ 0x10E202, "PortSdCardNoWaitedInterrupt" },
|
|
{ 0x10E402, "PortSdCardWaitInterruptSwTimeout" },
|
|
{ 0x112002, "PortSdCardAbortCommandIssued" },
|
|
{ 0x113002, "PortSdCardNotSupported" },
|
|
{ 0x113202, "PortSdCardNotImplemented" },
|
|
{ 0x138002, "PortSdCardStorageDeviceInvalidated" },
|
|
{ 0x138202, "PortSdCardWriteVerifyError" },
|
|
{ 0x138402, "PortSdCardFileSystemInvalidatedByRemoved" },
|
|
{ 0x138602, "PortSdCardUnexpected" },
|
|
{ 0x138802, "GameCardAccessFailed" },
|
|
{ 0x138A02, "GameCardUnknown" },
|
|
{ 0x138C02, "GameCardUnexpectedDeadCode" },
|
|
{ 0x138E02, "GameCardPreconditionViolation" },
|
|
{ 0x139002, "GameCardNotImplemented" },
|
|
{ 0x139C02, "GameCardQueueFullFailure" },
|
|
{ 0x139E02, "GameCardLockerOutOfRange" },
|
|
{ 0x13A802, "GameCardFailedIoMappingForGpio" },
|
|
{ 0x13B002, "GameCardCardNotInserted" },
|
|
{ 0x13B202, "GameCardCardIdMismatch" },
|
|
{ 0x13B402, "GameCardCardNotActivated" },
|
|
{ 0x13B602, "GameCardNotAwakened" },
|
|
{ 0x13C402, "GameCardCardAccessFailure" },
|
|
{ 0x13C602, "GameCardCardAccessTimeout" },
|
|
{ 0x13C802, "GameCardCardFatal" },
|
|
{ 0x13CA02, "GameCardCardNeedRetry" },
|
|
{ 0x13CC02, "GameCardCardRetryFailure" },
|
|
{ 0x13D002, "GameCardRetryLimitOut" },
|
|
{ 0x13D202, "GameCardNeedRefresh" },
|
|
{ 0x13D402, "GameCardNeedRefreshAndCardNeedRetry" },
|
|
{ 0x13D802, "GameCardInvalidSecureAccess" },
|
|
{ 0x13DA02, "GameCardInvalidNormalAccess" },
|
|
{ 0x13DC02, "GameCardInvalidAccessAcrossMode" },
|
|
{ 0x13DE02, "GameCardWrongCard" },
|
|
{ 0x13E002, "GameCardInitialDataMismatch" },
|
|
{ 0x13E202, "GameCardInitialNotFilledWithZero" },
|
|
{ 0x13E402, "GameCardKekIndexMismatch" },
|
|
{ 0x13E802, "GameCardInvalidGetCardDeviceCertificate" },
|
|
{ 0x13EA02, "GameCardUnregisteredCardSecureMethod" },
|
|
{ 0x13EC02, "GameCardCardNeedRetryAfterAsicReinitialize" },
|
|
{ 0x13EE02, "GameCardCardHeaderReadFailure" },
|
|
{ 0x13F002, "GameCardCardReinitializeFailure" },
|
|
{ 0x13F202, "GameCardInvalidChallengeCardExistenceMode" },
|
|
{ 0x13F402, "GameCardInvalidCardHeader" },
|
|
{ 0x13F602, "GameCardInvalidT1CardCertificate" },
|
|
{ 0x13FA02, "GameCardInvalidCa10Certificate" },
|
|
{ 0x13FC02, "GameCardInvalidCa10CardHeader" },
|
|
{ 0x140A02, "GameCardCommunicationFailure" },
|
|
{ 0x140C02, "GameCardFinishOperationFailed" },
|
|
{ 0x144A02, "GameCardStateTransitionFailure" },
|
|
{ 0x144C02, "GameCardAlreadyTransitionedState" },
|
|
{ 0x144E02, "GameCardShouldTransitFromAsicInitialToSecure" },
|
|
{ 0x145002, "GameCardShouldTransitFromInitialToNormal" },
|
|
{ 0x145202, "GameCardShouldTransitFromNormalModeToSecure" },
|
|
{ 0x145402, "GameCardShouldTransitFromNormalModeToDebug" },
|
|
{ 0x148A02, "GameCardInitializeAsicFailure" },
|
|
{ 0x148C02, "GameCardAlreadyInitializedAsic" },
|
|
{ 0x148E02, "GameCardActivateAsicFailure" },
|
|
{ 0x149002, "GameCardAsicBootFailure" },
|
|
{ 0x149402, "GameCardSendFirmwareFailure" },
|
|
{ 0x149802, "GameCardVerifyCertificateFailure" },
|
|
{ 0x149A02, "GameCardReceiveCertificateFailure" },
|
|
{ 0x149C02, "GameCardParseCertificateFailure" },
|
|
{ 0x149E02, "GameCardInvalidCertificate" },
|
|
{ 0x14A002, "GameCardSendSocCertificateFailure" },
|
|
{ 0x14A802, "GameCardGenerateCommonKeyFailure" },
|
|
{ 0x14AA02, "GameCardReceiveRandomValueFailure" },
|
|
{ 0x14AC02, "GameCardSendRandomValueFailure" },
|
|
{ 0x14AE02, "GameCardDecryptRandomValueFailure" },
|
|
{ 0x14B402, "GameCardAuthenticateMutuallyFailure" },
|
|
{ 0x14B602, "GameCardReceiveDeviceChallengeFailure" },
|
|
{ 0x14B802, "GameCardRespondDeviceChallengeFailure" },
|
|
{ 0x14BA02, "GameCardSendHostChallengeFailure" },
|
|
{ 0x14BC02, "GameCardReceiveChallengeResponseFailure" },
|
|
{ 0x14BE02, "GameCardChallengeAndResponseFailure" },
|
|
{ 0x14C402, "GameCardChangeModeToSecureFailure" },
|
|
{ 0x14C602, "GameCardExchangeRandomValuesFailure" },
|
|
{ 0x14C802, "GameCardAsicChallengeCardExistenceFailure" },
|
|
{ 0x14CE02, "GameCardInitializeAsicTimeOut" },
|
|
{ 0x14D202, "GameCardSplFailure" },
|
|
{ 0x14D402, "GameCardSplDecryptAesKeyFailure" },
|
|
{ 0x14D602, "GameCardSplDecryptAndStoreGcKeyFailure" },
|
|
{ 0x14D802, "GameCardSplGenerateRandomBytesFailure" },
|
|
{ 0x14DA02, "GameCardSplDecryptGcMessageFailure" },
|
|
{ 0x14DE02, "GameCardReadRegisterFailure" },
|
|
{ 0x14E002, "GameCardWriteRegisterFailure" },
|
|
{ 0x14E202, "GameCardEnableCardBusFailure" },
|
|
{ 0x14E402, "GameCardGetCardHeaderFailure" },
|
|
{ 0x14E602, "GameCardAsicStatusError" },
|
|
{ 0x14E802, "GameCardChangeGcModeToSecureFailure" },
|
|
{ 0x14EA02, "GameCardChangeGcModeToDebugFailure" },
|
|
{ 0x14EC02, "GameCardReadRmaInfoFailure" },
|
|
{ 0x14F002, "GameCardUpdateKeyFailure" },
|
|
{ 0x14F202, "GameCardKeySourceNotFound" },
|
|
{ 0x150402, "GameCardStateFailure" },
|
|
{ 0x150602, "GameCardStateCardNormalModeRequired" },
|
|
{ 0x150802, "GameCardStateCardSecureModeRequired" },
|
|
{ 0x150A02, "GameCardStateCardDebugModeRequired" },
|
|
{ 0x150C02, "GameCardStateAsicInitialRequired" },
|
|
{ 0x150E02, "GameCardStateAsicSecureRequired" },
|
|
{ 0x151802, "GameCardGeneralIoFailure" },
|
|
{ 0x151A02, "GameCardGeneralIoReleaseAsicResetFailure" },
|
|
{ 0x151C02, "GameCardGeneralIoHoldAsicResetFailure" },
|
|
{ 0x151E02, "GameCardSetVoltageFailure" },
|
|
{ 0x152C02, "GameCardDataIoFailure" },
|
|
{ 0x152E02, "GameCardDataIoActivateFailure" },
|
|
{ 0x155402, "GameCardCardCommandFailure" },
|
|
{ 0x155602, "GameCardCommandReadId1Failure" },
|
|
{ 0x155802, "GameCardCommandReadId2Failure" },
|
|
{ 0x155A02, "GameCardCommandReadId3Failure" },
|
|
{ 0x155C02, "GameCardSendCardReadUidFailure" },
|
|
{ 0x155E02, "GameCardCommandReadPageFailure" },
|
|
{ 0x156002, "GameCardCommandReadPageUnalignedFailure" },
|
|
{ 0x156202, "GameCardCommandWritePageFailure" },
|
|
{ 0x156402, "GameCardCommandRefreshFailure" },
|
|
{ 0x156602, "GameCardCommandUpdateKeyFailure" },
|
|
{ 0x156802, "GameCardSendCardSelfRefreshFailure" },
|
|
{ 0x156A02, "GameCardSendCardReadRefreshStatusFailure" },
|
|
{ 0x156C02, "GameCardCommandReadCrcFailure" },
|
|
{ 0x156E02, "GameCardCommandEraseFailure" },
|
|
{ 0x157002, "GameCardCommandReadDevParamFailure" },
|
|
{ 0x157202, "GameCardCommandWriteDevParamFailure" },
|
|
{ 0x157402, "GameCardSendCardReadErrorCountFailure" },
|
|
{ 0x16A802, "GameCardDevCardUnexpectedFailure" },
|
|
{ 0x16AA02, "GameCardDebugParameterMismatch" },
|
|
{ 0x16AC02, "GameCardDebugEraseFailure" },
|
|
{ 0x16AE02, "GameCardDebugWriteCrcMismatch" },
|
|
{ 0x16B002, "GameCardDebugCardReceivedIdMismatch" },
|
|
{ 0x16B202, "GameCardDebugCardId1Mismatch" },
|
|
{ 0x16B402, "GameCardDebugCardId2Mismatch" },
|
|
{ 0x170C02, "GameCardFsFailure" },
|
|
{ 0x170E02, "GameCardFsGetHandleFailure" },
|
|
{ 0x171002, "GameCardFsCheckHandleInReadFailure" },
|
|
{ 0x171202, "GameCardFsCheckHandleInWriteFailure" },
|
|
{ 0x171402, "GameCardFsCheckHandleInGetStatusFailure" },
|
|
{ 0x171602, "GameCardFsCheckHandleInGetDeviceCertFailure" },
|
|
{ 0x171802, "GameCardFsCheckHandleInGetCardImageHashFailure" },
|
|
{ 0x171A02, "GameCardFsCheckHandleInChallengeCardExistence" },
|
|
{ 0x171C02, "GameCardFsCheckHandleInOnAcquireLock" },
|
|
{ 0x171E02, "GameCardFsCheckModeInOnAcquireSecureLock" },
|
|
{ 0x172002, "GameCardFsCheckHandleInCreateReadOnlyFailure" },
|
|
{ 0x172202, "GameCardFsCheckHandleInCreateSecureReadOnlyFailure" },
|
|
{ 0x172402, "GameCardFsInvalidCompatibilityType" },
|
|
{ 0x172602, "GameCardNotSupportedOnDeviceModel" },
|
|
{ 0x177002, "Internal" },
|
|
{ 0x177202, "NotImplemented" },
|
|
{ 0x177402, "UnsupportedVersion" },
|
|
{ 0x177602, "AlreadyExists" },
|
|
{ 0x177A02, "OutOfRange" },
|
|
{ 0x183602, "StorageDeviceInvalidOperation" },
|
|
{ 0x183802, "SystemPartitionNotReady" },
|
|
{ 0x183A02, "StorageDeviceNotReady" },
|
|
{ 0x190002, "AllocationMemoryFailed" },
|
|
{ 0x190202, "AllocationMemoryFailedInFatFileSystemA" },
|
|
{ 0x190602, "AllocationMemoryFailedInFatFileSystemC" },
|
|
{ 0x190802, "AllocationMemoryFailedInFatFileSystemD" },
|
|
{ 0x190A02, "AllocationMemoryFailedInFatFileSystemE" },
|
|
{ 0x190C02, "AllocationMemoryFailedInFatFileSystemF" },
|
|
{ 0x191002, "AllocationMemoryFailedInFatFileSystemH" },
|
|
{ 0x191602, "AllocationMemoryFailedInFileSystemAccessorA" },
|
|
{ 0x191802, "AllocationMemoryFailedInFileSystemAccessorB" },
|
|
{ 0x191A02, "AllocationMemoryFailedInApplicationA" },
|
|
{ 0x191C02, "AllocationMemoryFailedInBcatSaveDataA" },
|
|
{ 0x191E02, "AllocationMemoryFailedInBisA" },
|
|
{ 0x192002, "AllocationMemoryFailedInBisB" },
|
|
{ 0x192202, "AllocationMemoryFailedInBisC" },
|
|
{ 0x192402, "AllocationMemoryFailedInCodeA" },
|
|
{ 0x192602, "AllocationMemoryFailedInContentA" },
|
|
{ 0x192802, "AllocationMemoryFailedInContentStorageA" },
|
|
{ 0x192A02, "AllocationMemoryFailedInContentStorageB" },
|
|
{ 0x192C02, "AllocationMemoryFailedInDataA" },
|
|
{ 0x192E02, "AllocationMemoryFailedInDataB" },
|
|
{ 0x193002, "AllocationMemoryFailedInDeviceSaveDataA" },
|
|
{ 0x193202, "AllocationMemoryFailedInGameCardA" },
|
|
{ 0x193402, "AllocationMemoryFailedInGameCardB" },
|
|
{ 0x193602, "AllocationMemoryFailedInGameCardC" },
|
|
{ 0x193802, "AllocationMemoryFailedInGameCardD" },
|
|
{ 0x193A02, "AllocationMemoryFailedInHostA" },
|
|
{ 0x193C02, "AllocationMemoryFailedInHostB" },
|
|
{ 0x193E02, "AllocationMemoryFailedInHostC" },
|
|
{ 0x194002, "AllocationMemoryFailedInImageDirectoryA" },
|
|
{ 0x194202, "AllocationMemoryFailedInLogoA" },
|
|
{ 0x194402, "AllocationMemoryFailedInRomA" },
|
|
{ 0x194602, "AllocationMemoryFailedInRomB" },
|
|
{ 0x194802, "AllocationMemoryFailedInRomC" },
|
|
{ 0x194A02, "AllocationMemoryFailedInRomD" },
|
|
{ 0x194C02, "AllocationMemoryFailedInRomE" },
|
|
{ 0x194E02, "AllocationMemoryFailedInRomF" },
|
|
{ 0x195402, "AllocationMemoryFailedInSaveDataManagementA" },
|
|
{ 0x195602, "AllocationMemoryFailedInSaveDataThumbnailA" },
|
|
{ 0x195802, "AllocationMemoryFailedInSdCardA" },
|
|
{ 0x195A02, "AllocationMemoryFailedInSdCardB" },
|
|
{ 0x195C02, "AllocationMemoryFailedInSystemSaveDataA" },
|
|
{ 0x195E02, "AllocationMemoryFailedInRomFsFileSystemA" },
|
|
{ 0x196002, "AllocationMemoryFailedInRomFsFileSystemB" },
|
|
{ 0x196202, "AllocationMemoryFailedInRomFsFileSystemC" },
|
|
{ 0x196602, "AllocationMemoryFailedInGuidPartitionTableA" },
|
|
{ 0x196802, "AllocationMemoryFailedInDeviceDetectionEventManagerA" },
|
|
{ 0x196A02, "AllocationMemoryFailedInSaveDataFileSystemServiceImplA" },
|
|
{ 0x196C02, "AllocationMemoryFailedInFileSystemProxyCoreImplB" },
|
|
{ 0x196E02, "AllocationMemoryFailedInSdCardProxyFileSystemCreatorA" },
|
|
{ 0x197002, "AllocationMemoryFailedInNcaFileSystemServiceImplA" },
|
|
{ 0x197202, "AllocationMemoryFailedInNcaFileSystemServiceImplB" },
|
|
{ 0x197402, "AllocationMemoryFailedInProgramRegistryManagerA" },
|
|
{ 0x197602, "AllocationMemoryFailedInSdmmcStorageServiceA" },
|
|
{ 0x197802, "AllocationMemoryFailedInBuiltInStorageCreatorA" },
|
|
{ 0x197A02, "AllocationMemoryFailedInBuiltInStorageCreatorB" },
|
|
{ 0x197C02, "AllocationMemoryFailedInBuiltInStorageCreatorC" },
|
|
{ 0x198002, "AllocationMemoryFailedFatFileSystemWithBufferA" },
|
|
{ 0x198202, "AllocationMemoryFailedInFatFileSystemCreatorA" },
|
|
{ 0x198402, "AllocationMemoryFailedInFatFileSystemCreatorB" },
|
|
{ 0x198602, "AllocationMemoryFailedInGameCardFileSystemCreatorA" },
|
|
{ 0x198802, "AllocationMemoryFailedInGameCardFileSystemCreatorB" },
|
|
{ 0x198A02, "AllocationMemoryFailedInGameCardFileSystemCreatorC" },
|
|
{ 0x198C02, "AllocationMemoryFailedInGameCardFileSystemCreatorD" },
|
|
{ 0x198E02, "AllocationMemoryFailedInGameCardFileSystemCreatorE" },
|
|
{ 0x199002, "AllocationMemoryFailedInGameCardFileSystemCreatorF" },
|
|
{ 0x199202, "AllocationMemoryFailedInGameCardManagerA" },
|
|
{ 0x199402, "AllocationMemoryFailedInGameCardManagerB" },
|
|
{ 0x199602, "AllocationMemoryFailedInGameCardManagerC" },
|
|
{ 0x199802, "AllocationMemoryFailedInGameCardManagerD" },
|
|
{ 0x199A02, "AllocationMemoryFailedInGameCardManagerE" },
|
|
{ 0x199C02, "AllocationMemoryFailedInGameCardManagerF" },
|
|
{ 0x199E02, "AllocationMemoryFailedInLocalFileSystemCreatorA" },
|
|
{ 0x19A002, "AllocationMemoryFailedInPartitionFileSystemCreatorA" },
|
|
{ 0x19A202, "AllocationMemoryFailedInRomFileSystemCreatorA" },
|
|
{ 0x19A402, "AllocationMemoryFailedInSaveDataFileSystemCreatorA" },
|
|
{ 0x19A602, "AllocationMemoryFailedInSaveDataFileSystemCreatorB" },
|
|
{ 0x19A802, "AllocationMemoryFailedInSaveDataFileSystemCreatorC" },
|
|
{ 0x19AA02, "AllocationMemoryFailedInSaveDataFileSystemCreatorD" },
|
|
{ 0x19AC02, "AllocationMemoryFailedInSaveDataFileSystemCreatorE" },
|
|
{ 0x19B002, "AllocationMemoryFailedInStorageOnNcaCreatorA" },
|
|
{ 0x19B202, "AllocationMemoryFailedInStorageOnNcaCreatorB" },
|
|
{ 0x19B402, "AllocationMemoryFailedInSubDirectoryFileSystemCreatorA" },
|
|
{ 0x19B602, "AllocationMemoryFailedInTargetManagerFileSystemCreatorA" },
|
|
{ 0x19B802, "AllocationMemoryFailedInSaveDataIndexerA" },
|
|
{ 0x19BA02, "AllocationMemoryFailedInSaveDataIndexerB" },
|
|
{ 0x19BC02, "AllocationMemoryFailedInFileSystemBuddyHeapA" },
|
|
{ 0x19BE02, "AllocationMemoryFailedInFileSystemBufferManagerA" },
|
|
{ 0x19C002, "AllocationMemoryFailedInBlockCacheBufferedStorageA" },
|
|
{ 0x19C202, "AllocationMemoryFailedInBlockCacheBufferedStorageB" },
|
|
{ 0x19C402, "AllocationMemoryFailedInDuplexStorageA" },
|
|
{ 0x19D002, "AllocationMemoryFailedInIntegrityVerificationStorageA" },
|
|
{ 0x19D202, "AllocationMemoryFailedInIntegrityVerificationStorageB" },
|
|
{ 0x19D402, "AllocationMemoryFailedInJournalStorageA" },
|
|
{ 0x19D602, "AllocationMemoryFailedInJournalStorageB" },
|
|
{ 0x19DC02, "AllocationMemoryFailedInSaveDataFileSystemCoreA" },
|
|
{ 0x19DE02, "AllocationMemoryFailedInSaveDataFileSystemCoreB" },
|
|
{ 0x19E002, "AllocationMemoryFailedInAesXtsFileA" },
|
|
{ 0x19E202, "AllocationMemoryFailedInAesXtsFileB" },
|
|
{ 0x19E402, "AllocationMemoryFailedInAesXtsFileC" },
|
|
{ 0x19E602, "AllocationMemoryFailedInAesXtsFileD" },
|
|
{ 0x19E802, "AllocationMemoryFailedInAesXtsFileSystemA" },
|
|
{ 0x19EE02, "AllocationMemoryFailedInConcatenationFileSystemA" },
|
|
{ 0x19F002, "AllocationMemoryFailedInConcatenationFileSystemB" },
|
|
{ 0x19F202, "AllocationMemoryFailedInDirectorySaveDataFileSystemA" },
|
|
{ 0x19F402, "AllocationMemoryFailedInLocalFileSystemA" },
|
|
{ 0x19F602, "AllocationMemoryFailedInLocalFileSystemB" },
|
|
{ 0x1A1A02, "AllocationMemoryFailedInNcaFileSystemDriverI" },
|
|
{ 0x1A2602, "AllocationMemoryFailedInPartitionFileSystemA" },
|
|
{ 0x1A2802, "AllocationMemoryFailedInPartitionFileSystemB" },
|
|
{ 0x1A2A02, "AllocationMemoryFailedInPartitionFileSystemC" },
|
|
{ 0x1A2C02, "AllocationMemoryFailedInPartitionFileSystemMetaA" },
|
|
{ 0x1A2E02, "AllocationMemoryFailedInPartitionFileSystemMetaB" },
|
|
{ 0x1A3002, "AllocationMemoryFailedInRomFsFileSystemD" },
|
|
{ 0x1A3602, "AllocationMemoryFailedInSubdirectoryFileSystemA" },
|
|
{ 0x1A3802, "AllocationMemoryFailedInTmFileSystemA" },
|
|
{ 0x1A3A02, "AllocationMemoryFailedInTmFileSystemB" },
|
|
{ 0x1A3E02, "AllocationMemoryFailedInProxyFileSystemA" },
|
|
{ 0x1A4002, "AllocationMemoryFailedInProxyFileSystemB" },
|
|
{ 0x1A4402, "AllocationMemoryFailedInSaveDataExtraDataAccessorCacheManagerA" },
|
|
{ 0x1A4602, "AllocationMemoryFailedInNcaReaderA" },
|
|
{ 0x1A4A02, "AllocationMemoryFailedInRegisterA" },
|
|
{ 0x1A4C02, "AllocationMemoryFailedInRegisterB" },
|
|
{ 0x1A4E02, "AllocationMemoryFailedInPathNormalizer" },
|
|
{ 0x1A5E02, "AllocationMemoryFailedInDbmRomKeyValueStorage" },
|
|
{ 0x1A6002, "AllocationMemoryFailedInDbmHierarchicalRomFileTable" },
|
|
{ 0x1A6202, "AllocationMemoryFailedInRomFsFileSystemE" },
|
|
{ 0x1A6402, "AllocationMemoryFailedInISaveFileSystemA" },
|
|
{ 0x1A6602, "AllocationMemoryFailedInISaveFileSystemB" },
|
|
{ 0x1A6802, "AllocationMemoryFailedInRomOnFileA" },
|
|
{ 0x1A6A02, "AllocationMemoryFailedInRomOnFileB" },
|
|
{ 0x1A6C02, "AllocationMemoryFailedInRomOnFileC" },
|
|
{ 0x1A6E02, "AllocationMemoryFailedInAesXtsFileE" },
|
|
{ 0x1A7002, "AllocationMemoryFailedInAesXtsFileF" },
|
|
{ 0x1A7202, "AllocationMemoryFailedInAesXtsFileG" },
|
|
{ 0x1A7402, "AllocationMemoryFailedInReadOnlyFileSystemA" },
|
|
{ 0x1A8402, "AllocationMemoryFailedInEncryptedFileSystemCreatorA" },
|
|
{ 0x1A8E02, "AllocationMemoryFailedInAesCtrCounterExtendedStorageA" },
|
|
{ 0x1A9002, "AllocationMemoryFailedInAesCtrCounterExtendedStorageB" },
|
|
{ 0x1A9C02, "AllocationMemoryFailedInSdmmcStorageServiceB" },
|
|
{ 0x1A9E02, "AllocationMemoryFailedInFileSystemInterfaceAdapterA" },
|
|
{ 0x1AA002, "AllocationMemoryFailedInGameCardFileSystemCreatorG" },
|
|
{ 0x1AA202, "AllocationMemoryFailedInGameCardFileSystemCreatorH" },
|
|
{ 0x1AA402, "AllocationMemoryFailedInAesXtsFileSystemB" },
|
|
{ 0x1AA602, "AllocationMemoryFailedInBufferedStorageA" },
|
|
{ 0x1AA802, "AllocationMemoryFailedInIntegrityRomFsStorageA" },
|
|
{ 0x1AB002, "AllocationMemoryFailedInSaveDataFileSystemServiceImplB" },
|
|
{ 0x1AB802, "AllocationMemoryFailedNew" },
|
|
{ 0x1ABA02, "AllocationMemoryFailedInFileSystemProxyImplA" },
|
|
{ 0x1ABC02, "AllocationMemoryFailedMakeUnique" },
|
|
{ 0x1ABE02, "AllocationMemoryFailedAllocateShared" },
|
|
{ 0x1AC002, "AllocationPooledBufferNotEnoughSize" },
|
|
{ 0x1AC802, "AllocationMemoryFailedInWriteThroughCacheStorageA" },
|
|
{ 0x1ACA02, "AllocationMemoryFailedInSaveDataTransferManagerA" },
|
|
{ 0x1ACC02, "AllocationMemoryFailedInSaveDataTransferManagerB" },
|
|
{ 0x1ACE02, "AllocationMemoryFailedInHtcFileSystemA" },
|
|
{ 0x1AD002, "AllocationMemoryFailedInHtcFileSystemB" },
|
|
{ 0x1AD202, "AllocationMemoryFailedInGameCardManagerG" },
|
|
{ 0x1B5802, "MmcAccessFailed" },
|
|
{ 0x1B5A02, "PortMmcNoDevice" },
|
|
{ 0x1B5C02, "PortMmcNotActivated" },
|
|
{ 0x1B5E02, "PortMmcDeviceRemoved" },
|
|
{ 0x1B6002, "PortMmcNotAwakened" },
|
|
{ 0x1B9802, "PortMmcCommunicationError" },
|
|
{ 0x1B9A02, "PortMmcCommunicationNotAttained" },
|
|
{ 0x1B9C02, "PortMmcResponseIndexError" },
|
|
{ 0x1B9E02, "PortMmcResponseEndBitError" },
|
|
{ 0x1BA002, "PortMmcResponseCrcError" },
|
|
{ 0x1BA202, "PortMmcResponseTimeoutError" },
|
|
{ 0x1BA402, "PortMmcDataEndBitError" },
|
|
{ 0x1BA602, "PortMmcDataCrcError" },
|
|
{ 0x1BA802, "PortMmcDataTimeoutError" },
|
|
{ 0x1BAA02, "PortMmcAutoCommandResponseIndexError" },
|
|
{ 0x1BAC02, "PortMmcAutoCommandResponseEndBitError" },
|
|
{ 0x1BAE02, "PortMmcAutoCommandResponseCrcError" },
|
|
{ 0x1BB002, "PortMmcAutoCommandResponseTimeoutError" },
|
|
{ 0x1BB202, "PortMmcCommandCompleteSwTimeout" },
|
|
{ 0x1BB402, "PortMmcTransferCompleteSwTimeout" },
|
|
{ 0x1BB802, "PortMmcDeviceStatusHasError" },
|
|
{ 0x1BBA02, "PortMmcDeviceStatusAddressOutOfRange" },
|
|
{ 0x1BBC02, "PortMmcDeviceStatusAddressMisalign" },
|
|
{ 0x1BBE02, "PortMmcDeviceStatusBlockLenError" },
|
|
{ 0x1BC002, "PortMmcDeviceStatusEraseSeqError" },
|
|
{ 0x1BC202, "PortMmcDeviceStatusEraseParam" },
|
|
{ 0x1BC402, "PortMmcDeviceStatusWpViolation" },
|
|
{ 0x1BC602, "PortMmcDeviceStatusLockUnlockFailed" },
|
|
{ 0x1BC802, "PortMmcDeviceStatusComCrcError" },
|
|
{ 0x1BCA02, "PortMmcDeviceStatusIllegalCommand" },
|
|
{ 0x1BCC02, "PortMmcDeviceStatusDeviceEccFailed" },
|
|
{ 0x1BCE02, "PortMmcDeviceStatusCcError" },
|
|
{ 0x1BD002, "PortMmcDeviceStatusError" },
|
|
{ 0x1BD202, "PortMmcDeviceStatusCidCsdOverwrite" },
|
|
{ 0x1BD402, "PortMmcDeviceStatusWpEraseSkip" },
|
|
{ 0x1BD602, "PortMmcDeviceStatusEraseReset" },
|
|
{ 0x1BD802, "PortMmcDeviceStatusSwitchError" },
|
|
{ 0x1BE802, "PortMmcUnexpectedDeviceState" },
|
|
{ 0x1BEA02, "PortMmcUnexpectedDeviceCsdValue" },
|
|
{ 0x1BEC02, "PortMmcAbortTransactionSwTimeout" },
|
|
{ 0x1BEE02, "PortMmcCommandInhibitCmdSwTimeout" },
|
|
{ 0x1BF002, "PortMmcCommandInhibitDatSwTimeout" },
|
|
{ 0x1BF202, "PortMmcBusySwTimeout" },
|
|
{ 0x1BF402, "PortMmcIssueTuningCommandSwTimeout" },
|
|
{ 0x1BF602, "PortMmcTuningFailed" },
|
|
{ 0x1BF802, "PortMmcMmcInitializationSwTimeout" },
|
|
{ 0x1BFA02, "PortMmcMmcNotSupportExtendedCsd" },
|
|
{ 0x1BFC02, "PortMmcUnexpectedMmcExtendedCsdValue" },
|
|
{ 0x1BFE02, "PortMmcMmcEraseSwTimeout" },
|
|
{ 0x1C0002, "PortMmcSdCardValidationError" },
|
|
{ 0x1C0202, "PortMmcSdCardInitializationSwTimeout" },
|
|
{ 0x1C0402, "PortMmcSdCardGetValidRcaSwTimeout" },
|
|
{ 0x1C0602, "PortMmcUnexpectedSdCardAcmdDisabled" },
|
|
{ 0x1C0802, "PortMmcSdCardNotSupportSwitchFunctionStatus" },
|
|
{ 0x1C0A02, "PortMmcUnexpectedSdCardSwitchFunctionStatus" },
|
|
{ 0x1C0C02, "PortMmcSdCardNotSupportAccessMode" },
|
|
{ 0x1C0E02, "PortMmcSdCardNot4BitBusWidthAtUhsIMode" },
|
|
{ 0x1C1002, "PortMmcSdCardNotSupportSdr104AndSdr50" },
|
|
{ 0x1C1202, "PortMmcSdCardCannotSwitchedAccessMode" },
|
|
{ 0x1C1402, "PortMmcSdCardFailedSwitchedAccessMode" },
|
|
{ 0x1C1602, "PortMmcSdCardUnacceptableCurrentConsumption" },
|
|
{ 0x1C1802, "PortMmcSdCardNotReadyToVoltageSwitch" },
|
|
{ 0x1C1A02, "PortMmcSdCardNotCompleteVoltageSwitch" },
|
|
{ 0x1C5802, "PortMmcHostControllerUnexpected" },
|
|
{ 0x1C5A02, "PortMmcInternalClockStableSwTimeout" },
|
|
{ 0x1C5C02, "PortMmcSdHostStandardUnknownAutoCmdError" },
|
|
{ 0x1C5E02, "PortMmcSdHostStandardUnknownError" },
|
|
{ 0x1C6002, "PortMmcSdmmcDllCalibrationSwTimeout" },
|
|
{ 0x1C6202, "PortMmcSdmmcDllApplicationSwTimeout" },
|
|
{ 0x1C6402, "PortMmcSdHostStandardFailSwitchTo18V" },
|
|
{ 0x1C9802, "PortMmcInternalError" },
|
|
{ 0x1C9A02, "PortMmcNoWaitedInterrupt" },
|
|
{ 0x1C9C02, "PortMmcWaitInterruptSwTimeout" },
|
|
{ 0x1CD802, "PortMmcAbortCommandIssued" },
|
|
{ 0x1CE802, "PortMmcNotSupported" },
|
|
{ 0x1CEA02, "PortMmcNotImplemented" },
|
|
{ 0x1F3C02, "PortMmcStorageDeviceInvalidated" },
|
|
{ 0x1F3E02, "PortMmcUnexpected" },
|
|
{ 0x1F4002, "DataCorrupted" },
|
|
{ 0x1F4202, "RomCorrupted" },
|
|
{ 0x1F4402, "UnsupportedRomVersion" },
|
|
{ 0x1F5602, "AesCtrCounterExtendedStorageCorrupted" },
|
|
{ 0x1F5802, "InvalidAesCtrCounterExtendedEntryOffset" },
|
|
{ 0x1F5A02, "InvalidAesCtrCounterExtendedTableSize" },
|
|
{ 0x1F5C02, "InvalidAesCtrCounterExtendedGeneration" },
|
|
{ 0x1F5E02, "InvalidAesCtrCounterExtendedOffset" },
|
|
{ 0x1F6002, "InvalidAesCtrCounterExtendedDataStorageSize" },
|
|
{ 0x1F6202, "InvalidAesCtrCounterExtendedMetaStorageSize" },
|
|
{ 0x1F6A02, "IndirectStorageCorrupted" },
|
|
{ 0x1F6C02, "InvalidIndirectEntryOffset" },
|
|
{ 0x1F6E02, "InvalidIndirectEntryStorageIndex" },
|
|
{ 0x1F7002, "InvalidIndirectStorageSize" },
|
|
{ 0x1F7202, "InvalidIndirectVirtualOffset" },
|
|
{ 0x1F7402, "InvalidIndirectPhysicalOffset" },
|
|
{ 0x1F7602, "InvalidIndirectStorageIndex" },
|
|
{ 0x1F7802, "InvalidIndirectStorageBucketTreeSize" },
|
|
{ 0x1F7E02, "BucketTreeCorrupted" },
|
|
{ 0x1F8002, "InvalidBucketTreeSignature" },
|
|
{ 0x1F8202, "InvalidBucketTreeEntryCount" },
|
|
{ 0x1F8402, "InvalidBucketTreeNodeEntryCount" },
|
|
{ 0x1F8602, "InvalidBucketTreeNodeOffset" },
|
|
{ 0x1F8802, "InvalidBucketTreeEntryOffset" },
|
|
{ 0x1F8A02, "InvalidBucketTreeEntrySetOffset" },
|
|
{ 0x1F8C02, "InvalidBucketTreeNodeIndex" },
|
|
{ 0x1F8E02, "InvalidBucketTreeVirtualOffset" },
|
|
{ 0x1F9202, "RomNcaCorrupted" },
|
|
{ 0x1FA602, "RomNcaFileSystemCorrupted" },
|
|
{ 0x1FA802, "InvalidRomNcaFileSystemType" },
|
|
{ 0x1FAA02, "InvalidRomAcidFileSize" },
|
|
{ 0x1FAC02, "InvalidRomAcidSize" },
|
|
{ 0x1FAE02, "InvalidRomAcid" },
|
|
{ 0x1FB002, "RomAcidVerificationFailed" },
|
|
{ 0x1FB202, "InvalidRomNcaSignature" },
|
|
{ 0x1FB402, "RomNcaHeaderSignature1VerificationFailed" },
|
|
{ 0x1FB602, "RomNcaHeaderSignature2VerificationFailed" },
|
|
{ 0x1FB802, "RomNcaFsHeaderHashVerificationFailed" },
|
|
{ 0x1FBA02, "InvalidRomNcaKeyIndex" },
|
|
{ 0x1FBC02, "InvalidRomNcaFsHeaderHashType" },
|
|
{ 0x1FBE02, "InvalidRomNcaFsHeaderEncryptionType" },
|
|
{ 0x1FC002, "InvalidRomNcaPatchInfoIndirectSize" },
|
|
{ 0x1FC202, "InvalidRomNcaPatchInfoAesCtrExSize" },
|
|
{ 0x1FC402, "InvalidRomNcaPatchInfoAesCtrExOffset" },
|
|
{ 0x1FC602, "InvalidRomNcaId" },
|
|
{ 0x1FC802, "InvalidRomNcaHeader" },
|
|
{ 0x1FCA02, "InvalidRomNcaFsHeader" },
|
|
{ 0x1FCC02, "InvalidRomNcaPatchInfoIndirectOffset" },
|
|
{ 0x1FCE02, "RomNcaHierarchicalSha256StorageCorrupted" },
|
|
{ 0x1FD002, "InvalidRomHierarchicalSha256BlockSize" },
|
|
{ 0x1FD202, "InvalidRomHierarchicalSha256LayerCount" },
|
|
{ 0x1FD402, "RomHierarchicalSha256BaseStorageTooLarge" },
|
|
{ 0x1FD602, "RomHierarchicalSha256HashVerificationFailed" },
|
|
{ 0x1FE202, "InvalidRomHierarchicalIntegrityVerificationLayerCount" },
|
|
{ 0x1FE402, "RomNcaIndirectStorageOutOfRange" },
|
|
{ 0x1FE602, "RomNcaInvalidCompressionInfo" },
|
|
{ 0x205A02, "RomIntegrityVerificationStorageCorrupted" },
|
|
{ 0x205C02, "IncorrectRomIntegrityVerificationMagicCode" },
|
|
{ 0x205E02, "InvalidRomZeroSignature" },
|
|
{ 0x206002, "RomNonRealDataVerificationFailed" },
|
|
{ 0x206E02, "RomRealDataVerificationFailed" },
|
|
{ 0x207002, "ClearedRomRealDataVerificationFailed" },
|
|
{ 0x207202, "UnclearedRomRealDataVerificationFailed" },
|
|
{ 0x20AA02, "RomPartitionFileSystemCorrupted" },
|
|
{ 0x20AC02, "InvalidRomSha256PartitionHashTarget" },
|
|
{ 0x20AE02, "RomSha256PartitionHashVerificationFailed" },
|
|
{ 0x20B002, "RomPartitionSignatureVerificationFailed" },
|
|
{ 0x20B202, "RomSha256PartitionSignatureVerificationFailed" },
|
|
{ 0x20B402, "InvalidRomPartitionEntryOffset" },
|
|
{ 0x20B602, "InvalidRomSha256PartitionMetaDataSize" },
|
|
{ 0x20D202, "RomBuiltInStorageCorrupted" },
|
|
{ 0x20D402, "RomGptHeaderSignatureVerificationFailed" },
|
|
{ 0x212202, "RomHostFileSystemCorrupted" },
|
|
{ 0x212402, "RomHostEntryCorrupted" },
|
|
{ 0x212602, "RomHostFileDataCorrupted" },
|
|
{ 0x212802, "RomHostFileCorrupted" },
|
|
{ 0x212A02, "InvalidRomHostHandle" },
|
|
{ 0x214A02, "RomDatabaseCorrupted" },
|
|
{ 0x214C02, "InvalidRomAllocationTableBlock" },
|
|
{ 0x214E02, "InvalidRomKeyValueListElementIndex" },
|
|
{ 0x217002, "RomStorageCorrupted" },
|
|
{ 0x217202, "InvalidRomStorageSize" },
|
|
{ 0x219A02, "SaveDataCorrupted" },
|
|
{ 0x219C02, "UnsupportedSaveDataVersion" },
|
|
{ 0x219E02, "InvalidSaveDataEntryType" },
|
|
{ 0x21A002, "ReconstructibleSaveDataCorrupted" },
|
|
{ 0x21AE02, "SaveDataFileSystemCorrupted" },
|
|
{ 0x21B002, "InvalidJournalIntegritySaveDataHashSize" },
|
|
{ 0x21B202, "InvalidJournalIntegritySaveDataCommitState" },
|
|
{ 0x21B402, "InvalidJournalIntegritySaveDataControlAreaSize" },
|
|
{ 0x21B602, "JournalIntegritySaveDataControlAreaVerificationFailed" },
|
|
{ 0x21B802, "JournalIntegritySaveDataMasterSignatureVerificationFailed" },
|
|
{ 0x21BA02, "IncorrectJournalIntegritySaveDataMagicCode" },
|
|
{ 0x21C202, "SaveDataDuplexStorageCorrupted" },
|
|
{ 0x21C402, "IncorrectDuplexMagicCode" },
|
|
{ 0x21C602, "DuplexStorageAccessOutOfRange" },
|
|
{ 0x21D602, "SaveDataMapCorrupted" },
|
|
{ 0x21D802, "InvalidMapEntryCount" },
|
|
{ 0x21DA02, "InvalidMapOffset" },
|
|
{ 0x21DC02, "InvalidMapSize" },
|
|
{ 0x21DE02, "InvalidMapAlignment" },
|
|
{ 0x21E002, "InvalidMapStorageType" },
|
|
{ 0x21E202, "MapAddressAlreadyRegistered" },
|
|
{ 0x21E402, "MapStorageNotFound" },
|
|
{ 0x21E602, "InvalidMapStorageSize" },
|
|
{ 0x21EA02, "SaveDataLogCorrupted" },
|
|
{ 0x21EC02, "InvalidLogBlockSize" },
|
|
{ 0x21EE02, "InvalidLogOffset" },
|
|
{ 0x21F002, "UnexpectedEndOfLog" },
|
|
{ 0x21F202, "LogNotFound" },
|
|
{ 0x220002, "ThumbnailHashVerificationFailed" },
|
|
{ 0x220A02, "InvalidSaveDataInternalStorageIntegritySeedSize" },
|
|
{ 0x220C02, "InvalidSaveDataInternalStorageAllocationTableFreeBitmapSizeA" },
|
|
{ 0x220E02, "InvalidSaveDataInternalStorageAllocationTableFreeBitmapSizeB" },
|
|
{ 0x221202, "SaveDataIntegrityVerificationStorageCorrupted" },
|
|
{ 0x221402, "IncorrectSaveDataIntegrityVerificationMagicCode" },
|
|
{ 0x221602, "InvalidSaveDataZeroHash" },
|
|
{ 0x221802, "SaveDataNonRealDataVerificationFailed" },
|
|
{ 0x222602, "SaveDataRealDataVerificationFailed" },
|
|
{ 0x222802, "ClearedSaveDataRealDataVerificationFailed" },
|
|
{ 0x222A02, "UnclearedSaveDataRealDataVerificationFailed" },
|
|
{ 0x226202, "SaveDataBuiltInStorageCorrupted" },
|
|
{ 0x226402, "SaveDataGptHeaderSignatureVerificationFailed" },
|
|
{ 0x227602, "SaveDataCoreFileSystemCorrupted" },
|
|
{ 0x227802, "IncorrectSaveDataFileSystemMagicCode" },
|
|
{ 0x227A02, "InvalidSaveDataFileReadOffset" },
|
|
{ 0x227C02, "InvalidSaveDataCoreDataStorageSize" },
|
|
{ 0x229602, "IncompleteBlockInZeroBitmapHashStorageFileSaveData" },
|
|
{ 0x229E02, "JournalStorageCorrupted" },
|
|
{ 0x22A002, "JournalStorageAccessOutOfRange" },
|
|
{ 0x22A202, "InvalidJournalStorageDataStorageSize" },
|
|
{ 0x22B202, "SaveDataHostFileSystemCorrupted" },
|
|
{ 0x22B402, "SaveDataHostEntryCorrupted" },
|
|
{ 0x22B602, "SaveDataHostFileDataCorrupted" },
|
|
{ 0x22B802, "SaveDataHostFileCorrupted" },
|
|
{ 0x22BA02, "InvalidSaveDataHostHandle" },
|
|
{ 0x22C602, "MappingTableCorrupted" },
|
|
{ 0x22C802, "InvalidMappingTableEntryCount" },
|
|
{ 0x22CA02, "InvalidMappingTablePhysicalIndex" },
|
|
{ 0x22CC02, "InvalidMappingTableVirtualIndex" },
|
|
{ 0x22DA02, "SaveDataDatabaseCorrupted" },
|
|
{ 0x22DC02, "InvalidSaveDataAllocationTableBlock" },
|
|
{ 0x22DE02, "InvalidSaveDataKeyValueListElementIndex" },
|
|
{ 0x22E002, "InvalidSaveDataAllocationTableChainEntry" },
|
|
{ 0x22E202, "InvalidSaveDataAllocationTableOffset" },
|
|
{ 0x22E402, "InvalidSaveDataAllocationTableBlockCount" },
|
|
{ 0x22E602, "InvalidSaveDataKeyValueListEntryIndex" },
|
|
{ 0x22E802, "InvalidSaveDataBitmapIndex" },
|
|
{ 0x230202, "SaveDataExtensionContextCorrupted" },
|
|
{ 0x230402, "IncorrectSaveDataExtensionContextMagicCode" },
|
|
{ 0x230602, "InvalidSaveDataExtensionContextState" },
|
|
{ 0x230802, "DifferentSaveDataExtensionContextParameter" },
|
|
{ 0x230A02, "InvalidSaveDataExtensionContextParameter" },
|
|
{ 0x231602, "IntegritySaveDataCorrupted" },
|
|
{ 0x231802, "InvalidIntegritySaveDataHashSize" },
|
|
{ 0x231C02, "InvalidIntegritySaveDataControlAreaSize" },
|
|
{ 0x231E02, "IntegritySaveDataControlAreaVerificationFailed" },
|
|
{ 0x232002, "IntegritySaveDataMasterSignatureVerificationFailed" },
|
|
{ 0x232202, "IncorrectIntegritySaveDataMagicCode" },
|
|
{ 0x232A02, "NcaCorrupted" },
|
|
{ 0x233802, "NcaBaseStorageOutOfRangeA" },
|
|
{ 0x233A02, "NcaBaseStorageOutOfRangeB" },
|
|
{ 0x233C02, "NcaBaseStorageOutOfRangeC" },
|
|
{ 0x233E02, "NcaFileSystemCorrupted" },
|
|
{ 0x234002, "InvalidNcaFileSystemType" },
|
|
{ 0x234202, "InvalidAcidFileSize" },
|
|
{ 0x234402, "InvalidAcidSize" },
|
|
{ 0x234602, "InvalidAcid" },
|
|
{ 0x234802, "AcidVerificationFailed" },
|
|
{ 0x234A02, "InvalidNcaSignature" },
|
|
{ 0x234C02, "NcaHeaderSignature1VerificationFailed" },
|
|
{ 0x234E02, "NcaHeaderSignature2VerificationFailed" },
|
|
{ 0x235002, "NcaFsHeaderHashVerificationFailed" },
|
|
{ 0x235202, "InvalidNcaKeyIndex" },
|
|
{ 0x235402, "InvalidNcaFsHeaderHashType" },
|
|
{ 0x235602, "InvalidNcaFsHeaderEncryptionType" },
|
|
{ 0x235802, "InvalidNcaPatchInfoIndirectSize" },
|
|
{ 0x235A02, "InvalidNcaPatchInfoAesCtrExSize" },
|
|
{ 0x235C02, "InvalidNcaPatchInfoAesCtrExOffset" },
|
|
{ 0x235E02, "InvalidNcaId" },
|
|
{ 0x236002, "InvalidNcaHeader" },
|
|
{ 0x236202, "InvalidNcaFsHeader" },
|
|
{ 0x236402, "InvalidNcaPatchInfoIndirectOffset" },
|
|
{ 0x236602, "NcaHierarchicalSha256StorageCorrupted" },
|
|
{ 0x236802, "InvalidHierarchicalSha256BlockSize" },
|
|
{ 0x236A02, "InvalidHierarchicalSha256LayerCount" },
|
|
{ 0x236C02, "HierarchicalSha256BaseStorageTooLarge" },
|
|
{ 0x236E02, "HierarchicalSha256HashVerificationFailed" },
|
|
{ 0x237A02, "InvalidHierarchicalIntegrityVerificationLayerCount" },
|
|
{ 0x237C02, "NcaIndirectStorageOutOfRange" },
|
|
{ 0x237E02, "InvalidNcaHeader1SignatureKeyGeneration" },
|
|
{ 0x238202, "InvalidNspdVerificationData" },
|
|
{ 0x238402, "MissingNspdVerificationData" },
|
|
{ 0x238602, "NcaInvalidCompressionInfo" },
|
|
{ 0x23F202, "IntegrityVerificationStorageCorrupted" },
|
|
{ 0x23F402, "IncorrectIntegrityVerificationMagicCode" },
|
|
{ 0x23F602, "InvalidZeroHash" },
|
|
{ 0x23F802, "NonRealDataVerificationFailed" },
|
|
{ 0x240602, "RealDataVerificationFailed" },
|
|
{ 0x240802, "ClearedRealDataVerificationFailed" },
|
|
{ 0x240A02, "UnclearedRealDataVerificationFailed" },
|
|
{ 0x244202, "PartitionFileSystemCorrupted" },
|
|
{ 0x244402, "InvalidSha256PartitionHashTarget" },
|
|
{ 0x244602, "Sha256PartitionHashVerificationFailed" },
|
|
{ 0x244802, "PartitionSignatureVerificationFailed" },
|
|
{ 0x244A02, "Sha256PartitionSignatureVerificationFailed" },
|
|
{ 0x244C02, "InvalidPartitionEntryOffset" },
|
|
{ 0x244E02, "InvalidSha256PartitionMetaDataSize" },
|
|
{ 0x246A02, "BuiltInStorageCorrupted" },
|
|
{ 0x246C02, "GptHeaderSignatureVerificationFailed" },
|
|
{ 0x247002, "GptHeaderInvalidPartitionSize" },
|
|
{ 0x249202, "FatFileSystemCorrupted" },
|
|
{ 0x249602, "InvalidFatFormat" },
|
|
{ 0x249802, "InvalidFatFileNumber" },
|
|
{ 0x249A02, "ExFatUnavailable" },
|
|
{ 0x249C02, "InvalidFatFormatBisUser" },
|
|
{ 0x249E02, "InvalidFatFormatBisSystem" },
|
|
{ 0x24A002, "InvalidFatFormatBisSafe" },
|
|
{ 0x24A202, "InvalidFatFormatBisCalibration" },
|
|
{ 0x24A402, "InvalidFatFormatSd" },
|
|
{ 0x24BA02, "HostFileSystemCorrupted" },
|
|
{ 0x24BC02, "HostEntryCorrupted" },
|
|
{ 0x24BE02, "HostFileDataCorrupted" },
|
|
{ 0x24C002, "HostFileCorrupted" },
|
|
{ 0x24C202, "InvalidHostHandle" },
|
|
{ 0x24E202, "DatabaseCorrupted" },
|
|
{ 0x24E402, "InvalidAllocationTableBlock" },
|
|
{ 0x24E602, "InvalidKeyValueListElementIndex" },
|
|
{ 0x24E802, "InvalidAllocationTableChainEntry" },
|
|
{ 0x24EA02, "InvalidAllocationTableOffset" },
|
|
{ 0x24EC02, "InvalidAllocationTableBlockCount" },
|
|
{ 0x24EE02, "InvalidKeyValueListEntryIndex" },
|
|
{ 0x24F002, "InvalidBitmapIndex" },
|
|
{ 0x250A02, "AesXtsFileSystemCorrupted" },
|
|
{ 0x250C02, "AesXtsFileSystemFileHeaderSizeCorruptedOnFileOpen" },
|
|
{ 0x250E02, "AesXtsFileSystemFileHeaderCorruptedOnFileOpen" },
|
|
{ 0x251002, "AesXtsFileSystemFileNoHeaderOnFileOpen" },
|
|
{ 0x251202, "AesXtsFileSystemFileSizeCorruptedOnFileOpen" },
|
|
{ 0x251402, "AesXtsFileSystemFileSizeCorruptedOnFileSetSize" },
|
|
{ 0x251602, "AesXtsFileSystemFileHeaderCorruptedOnRename" },
|
|
{ 0x251802, "AesXtsFileSystemFileHeaderCorruptedOnFileSetSize" },
|
|
{ 0x253202, "SaveDataTransferDataCorrupted" },
|
|
{ 0x253402, "SaveDataTransferTokenMacVerificationFailed" },
|
|
{ 0x253602, "SaveDataTransferTokenSignatureVerificationFailed" },
|
|
{ 0x253802, "SaveDataTransferTokenChallengeVerificationFailed" },
|
|
{ 0x253A02, "SaveDataTransferImportMacVerificationFailed" },
|
|
{ 0x253C02, "SaveDataTransferInitialDataMacVerificationFailed" },
|
|
{ 0x253E02, "SaveDataTransferInitialDataVersionVerificationFailed" },
|
|
{ 0x254602, "SignedSystemPartitionDataCorrupted" },
|
|
{ 0x254802, "SignedSystemPartitionInvalidSize" },
|
|
{ 0x254A02, "SignedSystemPartitionSignatureVerificationFailed" },
|
|
{ 0x254C02, "SignedSystemPartitionHashVerificationFailed" },
|
|
{ 0x254E02, "SignedSystemPartitionPackage2HashVerificationFailed" },
|
|
{ 0x255002, "SignedSystemPartitionInvalidAppendHashCount" },
|
|
{ 0x255A02, "GameCardLogoDataCorrupted" },
|
|
{ 0x256202, "SimulatedDeviceDataCorrupted" },
|
|
{ 0x256C02, "MultiCommitContextCorrupted" },
|
|
{ 0x256E02, "InvalidMultiCommitContextVersion" },
|
|
{ 0x257002, "InvalidMultiCommitContextState" },
|
|
{ 0x258402, "ConcatenationFsInvalidInternalFileCount" },
|
|
{ 0x259602, "ZeroBitmapFileCorrupted" },
|
|
{ 0x259802, "IncompleteBlockInZeroBitmapHashStorageFile" },
|
|
{ 0x271002, "Unexpected" },
|
|
{ 0x271202, "FatFsUnexpected" },
|
|
{ 0x271402, "FatFsUnclassified" },
|
|
{ 0x271602, "FatFsStorageStateMissmatch" },
|
|
{ 0x274002, "FatFsTooManyFilesOpenedS" },
|
|
{ 0x274202, "FatFsTooManyFilesOpenedU" },
|
|
{ 0x274402, "FatFsNotAFile" },
|
|
{ 0x274802, "FatFsLockError" },
|
|
{ 0x274A02, "FatFsInternalError" },
|
|
{ 0x277E02, "FatFsModuleSafeError" },
|
|
{ 0x27EC02, "FatFsUnexpectedSystemError" },
|
|
{ 0x280002, "FatFsFormatUnexpected" },
|
|
{ 0x280202, "FatFsFormatUnsupportedSize" },
|
|
{ 0x280402, "FatFsFormatInvalidBpb" },
|
|
{ 0x280602, "FatFsFormatInvalidParameter" },
|
|
{ 0x280802, "FatFsFormatIllegalSectorsA" },
|
|
{ 0x280A02, "FatFsFormatIllegalSectorsB" },
|
|
{ 0x280C02, "FatFsFormatIllegalSectorsC" },
|
|
{ 0x280E02, "FatFsFormatIllegalSectorsD" },
|
|
{ 0x281602, "FatFsWriteVerifyError" },
|
|
{ 0x296A02, "UnexpectedInMountTableA" },
|
|
{ 0x296C02, "UnexpectedInJournalIntegritySaveDataFileSystemA" },
|
|
{ 0x296E02, "UnexpectedInJournalIntegritySaveDataFileSystemB" },
|
|
{ 0x297002, "UnexpectedInJournalIntegritySaveDataFileSystemC" },
|
|
{ 0x297202, "UnexpectedInLocalFileSystemA" },
|
|
{ 0x297402, "UnexpectedInLocalFileSystemB" },
|
|
{ 0x297602, "UnexpectedInLocalFileSystemC" },
|
|
{ 0x297802, "UnexpectedInLocalFileSystemD" },
|
|
{ 0x297A02, "UnexpectedInLocalFileSystemE" },
|
|
{ 0x297C02, "UnexpectedInLocalFileSystemF" },
|
|
{ 0x297E02, "UnexpectedInPathToolA" },
|
|
{ 0x298002, "UnexpectedInPathOnExecutionDirectoryA" },
|
|
{ 0x298202, "UnexpectedInPathOnExecutionDirectoryB" },
|
|
{ 0x298402, "UnexpectedInPathOnExecutionDirectoryC" },
|
|
{ 0x298602, "UnexpectedInAesCtrStorageA" },
|
|
{ 0x298802, "UnexpectedInAesXtsStorageA" },
|
|
{ 0x298A02, "UnexpectedInSaveDataInternalStorageFileSystemA" },
|
|
{ 0x298C02, "UnexpectedInSaveDataInternalStorageFileSystemB" },
|
|
{ 0x298E02, "UnexpectedInMountUtilityA" },
|
|
{ 0x299002, "UnexpectedInNcaFileSystemServiceImplA" },
|
|
{ 0x299202, "UnexpectedInRamDiskFileSystemA" },
|
|
{ 0x299402, "UnexpectedInBisWiperA" },
|
|
{ 0x299602, "UnexpectedInBisWiperB" },
|
|
{ 0x299802, "UnexpectedInCompressedStorageA" },
|
|
{ 0x299A02, "UnexpectedInCompressedStorageB" },
|
|
{ 0x299C02, "UnexpectedInCompressedStorageC" },
|
|
{ 0x299E02, "UnexpectedInCompressedStorageD" },
|
|
{ 0x29A002, "UnexpectedInPathA" },
|
|
{ 0x2EE002, "PreconditionViolation" },
|
|
{ 0x2EE202, "InvalidArgument" },
|
|
{ 0x2EE402, "InvalidPath" },
|
|
{ 0x2EE602, "TooLongPath" },
|
|
{ 0x2EE802, "InvalidCharacter" },
|
|
{ 0x2EEA02, "InvalidPathFormat" },
|
|
{ 0x2EEC02, "DirectoryUnobtainable" },
|
|
{ 0x2EEE02, "NotNormalized" },
|
|
{ 0x2F1C02, "InvalidPathForOperation" },
|
|
{ 0x2F1E02, "DirectoryUndeletable" },
|
|
{ 0x2F2002, "DirectoryUnrenamable" },
|
|
{ 0x2F2202, "IncompatiblePath" },
|
|
{ 0x2F2402, "RenameToOtherFileSystem" },
|
|
{ 0x2F5A02, "InvalidOffset" },
|
|
{ 0x2F5C02, "InvalidSize" },
|
|
{ 0x2F5E02, "NullptrArgument" },
|
|
{ 0x2F6002, "InvalidAlignment" },
|
|
{ 0x2F6202, "InvalidMountName" },
|
|
{ 0x2F6402, "ExtensionSizeTooLarge" },
|
|
{ 0x2F6602, "ExtensionSizeInvalid" },
|
|
{ 0x2F6802, "InvalidHandle" },
|
|
{ 0x2F6A02, "CacheStorageSizeTooLarge" },
|
|
{ 0x2F6C02, "CacheStorageIndexTooLarge" },
|
|
{ 0x2F6E02, "InvalidCommitNameCount" },
|
|
{ 0x2F7002, "InvalidModeForFileOpen" },
|
|
{ 0x2F7202, "InvalidFileSize" },
|
|
{ 0x2F7402, "InvalidModeForDirectoryOpen" },
|
|
{ 0x2F7602, "InvalidCommitOption" },
|
|
{ 0x2F8002, "InvalidEnumValue" },
|
|
{ 0x2F8202, "InvalidSaveDataState" },
|
|
{ 0x2F8402, "InvalidSaveDataSpaceId" },
|
|
{ 0x2FAA02, "GameCardLogoDataTooLarge" },
|
|
{ 0x2FAC02, "FileDataCacheMemorySizeTooSmall" },
|
|
{ 0x307002, "InvalidOperationForOpenMode" },
|
|
{ 0x307202, "FileExtensionWithoutOpenModeAllowAppend" },
|
|
{ 0x307402, "ReadUnpermitted" },
|
|
{ 0x307602, "WriteUnpermitted" },
|
|
{ 0x313802, "UnsupportedOperation" },
|
|
{ 0x313A02, "UnsupportedCommitTarget" },
|
|
{ 0x313C02, "UnsupportedSetSizeForNotResizableSubStorage" },
|
|
{ 0x313E02, "UnsupportedSetSizeForResizableSubStorage" },
|
|
{ 0x314002, "UnsupportedSetSizeForMemoryStorage" },
|
|
{ 0x314202, "UnsupportedOperateRangeForMemoryStorage" },
|
|
{ 0x314402, "UnsupportedOperateRangeForFileStorage" },
|
|
{ 0x314602, "UnsupportedOperateRangeForFileHandleStorage" },
|
|
{ 0x314802, "UnsupportedOperateRangeForSwitchStorage" },
|
|
{ 0x314A02, "UnsupportedOperateRangeForStorageServiceObjectAdapter" },
|
|
{ 0x314C02, "UnsupportedWriteForAesCtrCounterExtendedStorage" },
|
|
{ 0x314E02, "UnsupportedSetSizeForAesCtrCounterExtendedStorage" },
|
|
{ 0x315002, "UnsupportedOperateRangeForAesCtrCounterExtendedStorage" },
|
|
{ 0x315202, "UnsupportedWriteForAesCtrStorageExternal" },
|
|
{ 0x315402, "UnsupportedSetSizeForAesCtrStorageExternal" },
|
|
{ 0x315602, "UnsupportedSetSizeForAesCtrStorage" },
|
|
{ 0x315802, "UnsupportedSetSizeForHierarchicalIntegrityVerificationStorage" },
|
|
{ 0x315A02, "UnsupportedOperateRangeForHierarchicalIntegrityVerificationStorage" },
|
|
{ 0x315C02, "UnsupportedSetSizeForIntegrityVerificationStorage" },
|
|
{ 0x315E02, "UnsupportedOperateRangeForWritableIntegrityVerificationStorage" },
|
|
{ 0x316002, "UnsupportedOperateRangeForIntegrityVerificationStorage" },
|
|
{ 0x316202, "UnsupportedSetSizeForBlockCacheBufferedStorage" },
|
|
{ 0x316402, "UnsupportedOperateRangeForWritableBlockCacheBufferedStorage" },
|
|
{ 0x316602, "UnsupportedOperateRangeForBlockCacheBufferedStorage" },
|
|
{ 0x316802, "UnsupportedWriteForIndirectStorage" },
|
|
{ 0x316A02, "UnsupportedSetSizeForIndirectStorage" },
|
|
{ 0x316C02, "UnsupportedOperateRangeForIndirectStorage" },
|
|
{ 0x316E02, "UnsupportedWriteForZeroStorage" },
|
|
{ 0x317002, "UnsupportedSetSizeForZeroStorage" },
|
|
{ 0x317202, "UnsupportedSetSizeForHierarchicalSha256Storage" },
|
|
{ 0x317402, "UnsupportedWriteForReadOnlyBlockCacheStorage" },
|
|
{ 0x317602, "UnsupportedSetSizeForReadOnlyBlockCacheStorage" },
|
|
{ 0x317802, "UnsupportedSetSizeForIntegrityRomFsStorage" },
|
|
{ 0x317A02, "UnsupportedSetSizeForDuplexStorage" },
|
|
{ 0x317C02, "UnsupportedOperateRangeForDuplexStorage" },
|
|
{ 0x317E02, "UnsupportedSetSizeForHierarchicalDuplexStorage" },
|
|
{ 0x318002, "UnsupportedGetSizeForRemapStorage" },
|
|
{ 0x318202, "UnsupportedSetSizeForRemapStorage" },
|
|
{ 0x318402, "UnsupportedOperateRangeForRemapStorage" },
|
|
{ 0x318602, "UnsupportedSetSizeForIntegritySaveDataStorage" },
|
|
{ 0x318802, "UnsupportedOperateRangeForIntegritySaveDataStorage" },
|
|
{ 0x318A02, "UnsupportedSetSizeForJournalIntegritySaveDataStorage" },
|
|
{ 0x318C02, "UnsupportedOperateRangeForJournalIntegritySaveDataStorage" },
|
|
{ 0x318E02, "UnsupportedGetSizeForJournalStorage" },
|
|
{ 0x319002, "UnsupportedSetSizeForJournalStorage" },
|
|
{ 0x319202, "UnsupportedOperateRangeForJournalStorage" },
|
|
{ 0x319402, "UnsupportedSetSizeForUnionStorage" },
|
|
{ 0x319602, "UnsupportedSetSizeForAllocationTableStorage" },
|
|
{ 0x319802, "UnsupportedReadForWriteOnlyGameCardStorage" },
|
|
{ 0x319A02, "UnsupportedSetSizeForWriteOnlyGameCardStorage" },
|
|
{ 0x319C02, "UnsupportedWriteForReadOnlyGameCardStorage" },
|
|
{ 0x319E02, "UnsupportedSetSizeForReadOnlyGameCardStorage" },
|
|
{ 0x31A002, "UnsupportedOperateRangeForReadOnlyGameCardStorage" },
|
|
{ 0x31A202, "UnsupportedSetSizeForSdmmcStorage" },
|
|
{ 0x31A402, "UnsupportedOperateRangeForSdmmcStorage" },
|
|
{ 0x31A602, "UnsupportedOperateRangeForFatFile" },
|
|
{ 0x31A802, "UnsupportedOperateRangeForStorageFile" },
|
|
{ 0x31AA02, "UnsupportedSetSizeForInternalStorageConcatenationFile" },
|
|
{ 0x31AC02, "UnsupportedOperateRangeForInternalStorageConcatenationFile" },
|
|
{ 0x31AE02, "UnsupportedQueryEntryForConcatenationFileSystem" },
|
|
{ 0x31B002, "UnsupportedOperateRangeForConcatenationFile" },
|
|
{ 0x31B202, "UnsupportedSetSizeForZeroBitmapFile" },
|
|
{ 0x31B402, "UnsupportedOperateRangeForFileServiceObjectAdapter" },
|
|
{ 0x31B602, "UnsupportedOperateRangeForAesXtsFile" },
|
|
{ 0x31B802, "UnsupportedWriteForRomFsFileSystem" },
|
|
{ 0x31BA02, "UnsupportedCommitProvisionallyForRomFsFileSystem" },
|
|
{ 0x31BC02, "UnsupportedGetTotalSpaceSizeForRomFsFileSystem" },
|
|
{ 0x31BE02, "UnsupportedWriteForRomFsFile" },
|
|
{ 0x31C002, "UnsupportedOperateRangeForRomFsFile" },
|
|
{ 0x31C202, "UnsupportedWriteForReadOnlyFileSystem" },
|
|
{ 0x31C402, "UnsupportedCommitProvisionallyForReadOnlyFileSystem" },
|
|
{ 0x31C602, "UnsupportedGetTotalSpaceSizeForReadOnlyFileSystem" },
|
|
{ 0x31C802, "UnsupportedWriteForReadOnlyFile" },
|
|
{ 0x31CA02, "UnsupportedOperateRangeForReadOnlyFile" },
|
|
{ 0x31CC02, "UnsupportedWriteForPartitionFileSystem" },
|
|
{ 0x31CE02, "UnsupportedCommitProvisionallyForPartitionFileSystem" },
|
|
{ 0x31D002, "UnsupportedWriteForPartitionFile" },
|
|
{ 0x31D202, "UnsupportedOperateRangeForPartitionFile" },
|
|
{ 0x31D402, "UnsupportedOperateRangeForTmFileSystemFile" },
|
|
{ 0x31D602, "UnsupportedWriteForSaveDataInternalStorageFileSystem" },
|
|
{ 0x31DC02, "UnsupportedCommitProvisionallyForApplicationTemporaryFileSystem" },
|
|
{ 0x31DE02, "UnsupportedCommitProvisionallyForSaveDataFileSystem" },
|
|
{ 0x31E002, "UnsupportedCommitProvisionallyForDirectorySaveDataFileSystem" },
|
|
{ 0x31E202, "UnsupportedWriteForZeroBitmapHashStorageFile" },
|
|
{ 0x31E402, "UnsupportedSetSizeForZeroBitmapHashStorageFile" },
|
|
{ 0x31E602, "UnsupportedWriteForCompressedStorage" },
|
|
{ 0x31E802, "UnsupportedOperateRangeForCompressedStorage" },
|
|
{ 0x31F602, "UnsupportedRollbackOnlyModifiedForApplicationTemporaryFileSystem" },
|
|
{ 0x31F802, "UnsupportedRollbackOnlyModifiedForDirectorySaveDataFileSystem" },
|
|
{ 0x31FA02, "UnsupportedOperateRangeForRegionSwitchStorage" },
|
|
{ 0x320002, "PermissionDenied" },
|
|
{ 0x320602, "HostFileSystemOperationDisabled" },
|
|
{ 0x326402, "PortAcceptableCountLimited" },
|
|
{ 0x326802, "NcaExternalKeyInconsistent" },
|
|
{ 0x326C02, "NeedFlush" },
|
|
{ 0x326E02, "FileNotClosed" },
|
|
{ 0x327002, "DirectoryNotClosed" },
|
|
{ 0x327202, "WriteModeFileNotClosed" },
|
|
{ 0x327402, "AllocatorAlreadyRegistered" },
|
|
{ 0x327602, "DefaultAllocatorAlreadyUsed" },
|
|
{ 0x327802, "GameCardLogoDataSizeInvalid" },
|
|
{ 0x327A02, "AllocatorAlignmentViolation" },
|
|
{ 0x327C02, "GlobalFileDataCacheAlreadyEnabled" },
|
|
{ 0x327E02, "MultiCommitHasOverlappingTargets" },
|
|
{ 0x328002, "MultiCommitAlreadyInProgress" },
|
|
{ 0x328202, "UserNotExist" },
|
|
{ 0x328402, "DefaultGlobalFileDataCacheEnabled" },
|
|
{ 0x328602, "SaveDataRootPathUnavailable" },
|
|
{ 0x339002, "NotFound" },
|
|
{ 0x339402, "FileNotFound" },
|
|
{ 0x339602, "DirectoryNotFound" },
|
|
{ 0x339802, "DatabaseKeyNotFound" },
|
|
{ 0x339A02, "ProgramInfoNotFound" },
|
|
{ 0x339C02, "ProgramIndexNotFound" },
|
|
{ 0x345802, "OutOfResource" },
|
|
{ 0x346202, "BufferAllocationFailed" },
|
|
{ 0x346402, "MappingTableFull" },
|
|
{ 0x346602, "AllocationTableFull" },
|
|
{ 0x346A02, "OpenCountLimit" },
|
|
{ 0x346C02, "MultiCommitFileSystemLimit" },
|
|
{ 0x352002, "MappingFailed" },
|
|
{ 0x353602, "MapFull" },
|
|
{ 0x35E802, "BadState" },
|
|
{ 0x35EC02, "NotInitialized" },
|
|
{ 0x35EE02, "BisProxyInvalidated" },
|
|
{ 0x35F002, "NcaDigestInconsistent" },
|
|
{ 0x35F202, "NotMounted" },
|
|
{ 0x35F402, "SaveDataExtending" },
|
|
{ 0x35F602, "SaveDataToExpandIsProvisionallyCommitted" },
|
|
{ 0x36B402, "SaveDataTransferV2KeySeedPackageMacVerificationFailed" },
|
|
{ 0x36B602, "SaveDataTransferV2KeySeedPackageSignatureVerificationFailed" },
|
|
{ 0x36B802, "SaveDataTransferV2KeySeedPackageChallengeVerificationFailed" },
|
|
{ 0x36BA02, "SaveDataTransferV2ImportDataVerificationFailed" },
|
|
{ 0x36BC02, "SaveDataTransferV2InitialDataGcmMacVerificationFailed" },
|
|
{ 0x36C202, "SaveDataTransferV2InitialDataMacVerificationFailed" },
|
|
{ 0x36C402, "SaveDataTransferV2ImportDataDecompressionFailed" },
|
|
{ 0x36C602, "SaveDataTransferV2PortContextMacVerificationFailed" },
|
|
{ 0x36EE02, "SaveDataPorterInvalidated" },
|
|
{ 0x36F002, "SaveDataDivisionExporterChunkExportIncomplete" },
|
|
{ 0x36F202, "SaveDataDivisionImporterChunkImportIncomplete" },
|
|
{ 0x36F402, "SaveDataPorterInitialDataVersionVerificationFailed" },
|
|
{ 0x36F602, "SaveDataChunkDecryptorGcmStreamVersionVerificationFailed" },
|
|
{ 0x36F802, "SaveDataPorterSaveDataModified" },
|
|
{ 0x36FA02, "SaveDataPorterVersionUnsupported" },
|
|
{ 0x36FC02, "SaveDataTransferV2SecondarySaveCorrupted" },
|
|
{ 0x372C02, "SaveDataTransferForSaveDataRepairKeyPackageMacVerificationFailed" },
|
|
{ 0x372E02, "SaveDataTransferForSaveDataRepairKeyPackageSignatureVerificationFailed" },
|
|
{ 0x373002, "SaveDataTransferForSaveDataRepairKeyPackageChallengeVerificationFailed" },
|
|
{ 0x373202, "SaveDataTransferForSaveDataRepairUnsupportedKeyGeneration" },
|
|
{ 0x373402, "SaveDataTransferForSaveDataRepairInitialDataMacVerificationFailed" },
|
|
{ 0x373A02, "SaveDataTransferForSaveDataRepairIncorrectInitialData" },
|
|
{ 0x373C02, "SaveDataTransferForSaveDataRepairInconsistentInitialData" },
|
|
{ 0x373E02, "SaveDataTransferForSaveDataRepairInitialDataIncorrectUserId" },
|
|
{ 0x377802, "RamDiskCorrupted" },
|
|
{ 0x377A02, "RamDiskVerifiedStorageVerificationFailed" },
|
|
{ 0x378E02, "RamDiskSaveDataCoreFileSystemCorrupted" },
|
|
{ 0x379002, "IncorrectRamDiskSaveDataFileSystemMagicCode" },
|
|
{ 0x379202, "InvalidRamDiskSaveDataFileReadOffset" },
|
|
{ 0x379402, "InvalidRamDiskSaveDataCoreDataStorageSize" },
|
|
{ 0x37A202, "RamDiskDatabaseCorrupted" },
|
|
{ 0x37A402, "InvalidRamDiskAllocationTableBlock" },
|
|
{ 0x37A602, "InvalidRamDiskKeyValueListElementIndex" },
|
|
{ 0x37A802, "InvalidRamDiskAllocationTableChainEntry" },
|
|
{ 0x37AA02, "InvalidRamDiskAllocationTableOffset" },
|
|
{ 0x37AC02, "InvalidRamDiskAllocationTableBlockCount" },
|
|
{ 0x37AE02, "InvalidRamDiskKeyValueListEntryIndex" },
|
|
{ 0x37CC02, "SaveDataTransferForRepairInitialDataMacVerificationFailed" },
|
|
{ 0x3DB802, "Unknown" },
|
|
{ 0x3DBA02, "DbmNotFound" },
|
|
{ 0x3DBC02, "DbmKeyNotFound" },
|
|
{ 0x3DBE02, "DbmFileNotFound" },
|
|
{ 0x3DC002, "DbmDirectoryNotFound" },
|
|
{ 0x3DC402, "DbmAlreadyExists" },
|
|
{ 0x3DC602, "DbmKeyFull" },
|
|
{ 0x3DC802, "DbmDirectoryEntryFull" },
|
|
{ 0x3DCA02, "DbmFileEntryFull" },
|
|
{ 0x3DCC02, "DbmFindFinished" },
|
|
{ 0x3DCE02, "DbmFindKeyFinished" },
|
|
{ 0x3DD002, "DbmIterationFinished" },
|
|
{ 0x3DD402, "DbmInvalidOperation" },
|
|
{ 0x3DD602, "DbmInvalidPathFormat" },
|
|
{ 0x3DD802, "DbmDirectoryNameTooLong" },
|
|
{ 0x3DDA02, "DbmFileNameTooLong" },
|
|
{ 0x803, "Busy" },
|
|
{ 0x1003, "OutOfMemory" },
|
|
{ 0x1203, "OutOfResource" },
|
|
{ 0x1803, "OutOfVirtualAddressSpace" },
|
|
{ 0x1A03, "ResourceLimit" },
|
|
{ 0x3E803, "OutOfHandles" },
|
|
{ 0x3EA03, "InvalidHandle" },
|
|
{ 0x3EC03, "InvalidCurrentMemoryState" },
|
|
{ 0x3EE03, "InvalidTransferMemoryState" },
|
|
{ 0x3F003, "InvalidTransferMemorySize" },
|
|
{ 0x3F203, "OutOfTransferMemory" },
|
|
{ 0x3F403, "OutOfAddressSpace" },
|
|
{ 0x3FC03, "SessionClosedForReceive" },
|
|
{ 0x3FE03, "SessionClosedForReply" },
|
|
{ 0x40003, "ReceiveListBroken" },
|
|
{ 0x1204, "InvalidHandle" },
|
|
{ 0xFA204, "InvalidArgument" },
|
|
{ 0xFA604, "InvalidServerHandle" },
|
|
{ 0xFBC04, "InvalidSize" },
|
|
{ 0xFCA04, "Cancelled" },
|
|
{ 0xFCE04, "Completed" },
|
|
{ 0x106E04, "InvalidTask" },
|
|
{ 0x205, "InvalidContentStorageBase" },
|
|
{ 0x405, "PlaceHolderAlreadyExists" },
|
|
{ 0x605, "PlaceHolderNotFound" },
|
|
{ 0x805, "ContentAlreadyExists" },
|
|
{ 0xA05, "ContentNotFound" },
|
|
{ 0xE05, "ContentMetaNotFound" },
|
|
{ 0x1005, "AllocationFailed" },
|
|
{ 0x1805, "UnknownStorage" },
|
|
{ 0xC805, "InvalidContentStorage" },
|
|
{ 0xDC05, "InvalidContentMetaDatabase" },
|
|
{ 0x10405, "InvalidPackageFormat" },
|
|
{ 0x11805, "InvalidContentHash" },
|
|
{ 0x14005, "InvalidInstallTaskState" },
|
|
{ 0x15405, "InvalidPlaceHolderFile" },
|
|
{ 0x16805, "BufferInsufficient" },
|
|
{ 0x17C05, "WriteToReadOnlyContentStorage" },
|
|
{ 0x19005, "NotEnoughInstallSpace" },
|
|
{ 0x1A405, "SystemUpdateNotFoundInPackage" },
|
|
{ 0x1B805, "ContentInfoNotFound" },
|
|
{ 0x1DA05, "DeltaNotFound" },
|
|
{ 0x1E005, "InvalidContentMetaKey" },
|
|
{ 0x1F405, "ContentStorageNotActive" },
|
|
{ 0x1F605, "GameCardContentStorageNotActive" },
|
|
{ 0x1F805, "BuiltInSystemContentStorageNotActive" },
|
|
{ 0x1FA05, "BuiltInUserContentStorageNotActive" },
|
|
{ 0x1FC05, "SdCardContentStorageNotActive" },
|
|
{ 0x20405, "UnknownContentStorageNotActive" },
|
|
{ 0x20805, "ContentMetaDatabaseNotActive" },
|
|
{ 0x20A05, "GameCardContentMetaDatabaseNotActive" },
|
|
{ 0x20C05, "BuiltInSystemContentMetaDatabaseNotActive" },
|
|
{ 0x20E05, "BuiltInUserContentMetaDatabaseNotActive" },
|
|
{ 0x21005, "SdCardContentMetaDatabaseNotActive" },
|
|
{ 0x21805, "UnknownContentMetaDatabaseNotActive" },
|
|
{ 0x23005, "IgnorableInstallTicketFailure" },
|
|
{ 0x24405, "InstallTaskCancelled" },
|
|
{ 0x24605, "CreatePlaceHolderCancelled" },
|
|
{ 0x24805, "WritePlaceHolderCancelled" },
|
|
{ 0x26C05, "ContentStorageBaseNotFound" },
|
|
{ 0x29405, "ListPartiallyNotCommitted" },
|
|
{ 0x2D005, "UnexpectedContentMetaPrepared" },
|
|
{ 0x2F805, "InvalidFirmwareVariation" },
|
|
{ 0x3FEA05, "InvalidArgument" },
|
|
{ 0x3FEC05, "InvalidOffset" },
|
|
{ 0x206, "EndOfQuery" },
|
|
{ 0x406, "InvalidCurrentMemory" },
|
|
{ 0x606, "NotSingleRegion" },
|
|
{ 0x806, "InvalidMemoryState" },
|
|
{ 0xA06, "OutOfMemory" },
|
|
{ 0xC06, "OutOfResource" },
|
|
{ 0xE06, "NotSupported" },
|
|
{ 0x1006, "InvalidHandle" },
|
|
{ 0x7FE06, "InternalError" },
|
|
{ 0x208, "ResolverNotFound" },
|
|
{ 0x408, "ProgramNotFound" },
|
|
{ 0x608, "DataNotFound" },
|
|
{ 0x808, "UnknownResolver" },
|
|
{ 0xA08, "ApplicationNotFound" },
|
|
{ 0xC08, "HtmlDocumentNotFound" },
|
|
{ 0xE08, "AddOnContentNotFound" },
|
|
{ 0x1008, "ControlNotFound" },
|
|
{ 0x1208, "LegalInformationNotFound" },
|
|
{ 0x1408, "DebugProgramNotFound" },
|
|
{ 0xB408, "TooManyRegisteredPaths" },
|
|
{ 0x209, "TooLongArgument" },
|
|
{ 0x409, "TooManyArguments" },
|
|
{ 0x609, "TooLargeMeta" },
|
|
{ 0x809, "InvalidMeta" },
|
|
{ 0xA09, "InvalidNso" },
|
|
{ 0xC09, "InvalidPath" },
|
|
{ 0xE09, "TooManyProcesses" },
|
|
{ 0x1009, "NotPinned" },
|
|
{ 0x1209, "InvalidProgramId" },
|
|
{ 0x1409, "InvalidVersion" },
|
|
{ 0x1609, "InvalidAcidSignature" },
|
|
{ 0x1809, "InvalidNcaSignature" },
|
|
{ 0x6609, "InsufficientAddressSpace" },
|
|
{ 0x6809, "InvalidNro" },
|
|
{ 0x6A09, "InvalidNrr" },
|
|
{ 0x6C09, "InvalidSignature" },
|
|
{ 0x6E09, "InsufficientNroRegistrations" },
|
|
{ 0x7009, "InsufficientNrrRegistrations" },
|
|
{ 0x7209, "NroAlreadyLoaded" },
|
|
{ 0xA209, "InvalidAddress" },
|
|
{ 0xA409, "InvalidSize" },
|
|
{ 0xA809, "NotLoaded" },
|
|
{ 0xAA09, "NotRegistered" },
|
|
{ 0xAC09, "InvalidSession" },
|
|
{ 0xAE09, "InvalidProcess" },
|
|
{ 0xC809, "UnknownCapability" },
|
|
{ 0xCE09, "InvalidCapabilityKernelFlags" },
|
|
{ 0xD009, "InvalidCapabilitySyscallMask" },
|
|
{ 0xD409, "InvalidCapabilityMapRange" },
|
|
{ 0xD609, "InvalidCapabilityMapPage" },
|
|
{ 0xDE09, "InvalidCapabilityInterruptPair" },
|
|
{ 0xE209, "InvalidCapabilityApplicationType" },
|
|
{ 0xE409, "InvalidCapabilityKernelVersion" },
|
|
{ 0xE609, "InvalidCapabilityHandleTable" },
|
|
{ 0xE809, "InvalidCapabilityDebugFlags" },
|
|
{ 0x19009, "InternalError" },
|
|
{ 0x20A, "NotSupported" },
|
|
{ 0x60A, "PreconditionViolation" },
|
|
{ 0x140A, "MemoryAllocationFailed" },
|
|
{ 0x160A, "CmifProxyAllocationFailed" },
|
|
{ 0x1940A, "InvalidCmifHeaderSize" },
|
|
{ 0x1A60A, "InvalidCmifInHeader" },
|
|
{ 0x1A80A, "InvalidCmifOutHeader" },
|
|
{ 0x1BA0A, "UnknownMethodId" },
|
|
{ 0x1CE0A, "InvalidInRawSize" },
|
|
{ 0x1D00A, "InvalidOutRawSize" },
|
|
{ 0x1D60A, "InvalidInObjectCount" },
|
|
{ 0x1D80A, "InvalidOutObjectCount" },
|
|
{ 0x1DE0A, "InvalidInObject" },
|
|
{ 0x20A0A, "TargetObjectNotFound" },
|
|
{ 0x25A0A, "OutOfDomainEntry" },
|
|
{ 0x6400A, "RequestContextChanged" },
|
|
{ 0x6420A, "RequestInvalidated" },
|
|
{ 0x6440A, "RequestInvalidatedByUser" },
|
|
{ 0x6560A, "RequestDeferred" },
|
|
{ 0x6580A, "RequestDeferredByUser" },
|
|
{ 0x20B, "NotSupported" },
|
|
{ 0xC80B, "OutOfResource" },
|
|
{ 0xCC0B, "OutOfSessionMemory" },
|
|
{ 0x1060B, "OutOfSessions" },
|
|
{ 0x11A0B, "InsufficientPointerTransferBuffer" },
|
|
{ 0x1900B, "OutOfDomains" },
|
|
{ 0x2580B, "CommunicationError" },
|
|
{ 0x25A0B, "SessionClosed" },
|
|
{ 0x3240B, "InvalidRequestSize" },
|
|
{ 0x3260B, "UnknownCommandType" },
|
|
{ 0x3480B, "InvalidCmifRequest" },
|
|
{ 0x3D60B, "TargetNotDomain" },
|
|
{ 0x3D80B, "DomainObjectNotFound" },
|
|
{ 0x20C, "Unknown" },
|
|
{ 0x20D, "Unknown" },
|
|
{ 0x40D, "DebuggingDisabled" },
|
|
{ 0x20F, "ProcessNotFound" },
|
|
{ 0x40F, "AlreadyStarted" },
|
|
{ 0x60F, "NotTerminated" },
|
|
{ 0x80F, "DebugHookInUse" },
|
|
{ 0xA0F, "ApplicationRunning" },
|
|
{ 0xC0F, "InvalidSize" },
|
|
{ 0xB410, "Canceled" },
|
|
{ 0xDC10, "OutOfMaxRunningTask" },
|
|
{ 0x21C10, "CardUpdateNotSetup" },
|
|
{ 0x23010, "CardUpdateNotPrepared" },
|
|
{ 0x24410, "CardUpdateAlreadySetup" },
|
|
{ 0x39810, "PrepareCardUpdateAlreadyRequested" },
|
|
{ 0x212, "ConnectionFailure" },
|
|
{ 0x412, "NotFound" },
|
|
{ 0x612, "NotEnoughBuffer" },
|
|
{ 0xCA12, "Cancelled" },
|
|
{ 0x7FE12, "" },
|
|
{ 0xFA212, "" },
|
|
{ 0xFA612, "InvalidTaskId" },
|
|
{ 0xFB612, "InvalidSize" },
|
|
{ 0xFCA12, "TaskCancelled" },
|
|
{ 0xFCC12, "TaskNotCompleted" },
|
|
{ 0xFCE12, "TaskQueueNotAvailable" },
|
|
{ 0x106A12, "" },
|
|
{ 0x106C12, "OutOfRpcTask" },
|
|
{ 0x109612, "InvalidCategory" },
|
|
{ 0x214, "OutOfKeyResource" },
|
|
{ 0x414, "KeyNotFound" },
|
|
{ 0x814, "AllocationFailed" },
|
|
{ 0xA14, "InvalidKeyValue" },
|
|
{ 0xC14, "BufferInsufficient" },
|
|
{ 0x1014, "InvalidFileSystemState" },
|
|
{ 0x1214, "NotCreated" },
|
|
{ 0x215, "OutOfProcesses" },
|
|
{ 0x415, "InvalidClient" },
|
|
{ 0x615, "OutOfSessions" },
|
|
{ 0x815, "AlreadyRegistered" },
|
|
{ 0xA15, "OutOfServices" },
|
|
{ 0xC15, "InvalidServiceName" },
|
|
{ 0xE15, "NotRegistered" },
|
|
{ 0x1015, "NotAllowed" },
|
|
{ 0x1215, "TooLargeAccessControl" },
|
|
{ 0x216, "RoError" },
|
|
{ 0x416, "OutOfAddressSpace" },
|
|
{ 0x616, "AlreadyLoaded" },
|
|
{ 0x816, "InvalidNro" },
|
|
{ 0xC16, "InvalidNrr" },
|
|
{ 0xE16, "TooManyNro" },
|
|
{ 0x1016, "TooManyNrr" },
|
|
{ 0x1216, "NotAuthorized" },
|
|
{ 0x1416, "InvalidNrrKind" },
|
|
{ 0x7FE16, "InternalError" },
|
|
{ 0x80216, "InvalidAddress" },
|
|
{ 0x80416, "InvalidSize" },
|
|
{ 0x80816, "NotLoaded" },
|
|
{ 0x80A16, "NotRegistered" },
|
|
{ 0x80C16, "InvalidSession" },
|
|
{ 0x80E16, "InvalidProcess" },
|
|
{ 0x218, "NoDevice" },
|
|
{ 0x418, "NotActivated" },
|
|
{ 0x618, "DeviceRemoved" },
|
|
{ 0x818, "NotAwakened" },
|
|
{ 0x4018, "CommunicationError" },
|
|
{ 0x4218, "CommunicationNotAttained" },
|
|
{ 0x4418, "ResponseIndexError" },
|
|
{ 0x4618, "ResponseEndBitError" },
|
|
{ 0x4818, "ResponseCrcError" },
|
|
{ 0x4A18, "ResponseTimeoutError" },
|
|
{ 0x4C18, "DataEndBitError" },
|
|
{ 0x4E18, "DataCrcError" },
|
|
{ 0x5018, "DataTimeoutError" },
|
|
{ 0x5218, "AutoCommandResponseIndexError" },
|
|
{ 0x5418, "AutoCommandResponseEndBitError" },
|
|
{ 0x5618, "AutoCommandResponseCrcError" },
|
|
{ 0x5818, "AutoCommandResponseTimeoutError" },
|
|
{ 0x5A18, "CommandCompleteSoftwareTimeout" },
|
|
{ 0x5C18, "TransferCompleteSoftwareTimeout" },
|
|
{ 0x6018, "DeviceStatusHasError" },
|
|
{ 0x6218, "DeviceStatusAddressOutOfRange" },
|
|
{ 0x6418, "DeviceStatusAddressMisaligned" },
|
|
{ 0x6618, "DeviceStatusBlockLenError" },
|
|
{ 0x6818, "DeviceStatusEraseSeqError" },
|
|
{ 0x6A18, "DeviceStatusEraseParam" },
|
|
{ 0x6C18, "DeviceStatusWpViolation" },
|
|
{ 0x6E18, "DeviceStatusLockUnlockFailed" },
|
|
{ 0x7018, "DeviceStatusComCrcError" },
|
|
{ 0x7218, "DeviceStatusIllegalCommand" },
|
|
{ 0x7418, "DeviceStatusDeviceEccFailed" },
|
|
{ 0x7618, "DeviceStatusCcError" },
|
|
{ 0x7818, "DeviceStatusError" },
|
|
{ 0x7A18, "DeviceStatusCidCsdOverwrite" },
|
|
{ 0x7C18, "DeviceStatusWpEraseSkip" },
|
|
{ 0x7E18, "DeviceStatusEraseReset" },
|
|
{ 0x8018, "DeviceStatusSwitchError" },
|
|
{ 0x9018, "UnexpectedDeviceState" },
|
|
{ 0x9218, "UnexpectedDeviceCsdValue" },
|
|
{ 0x9418, "AbortTransactionSoftwareTimeout" },
|
|
{ 0x9618, "CommandInhibitCmdSoftwareTimeout" },
|
|
{ 0x9818, "CommandInhibitDatSoftwareTimeout" },
|
|
{ 0x9A18, "BusySoftwareTimeout" },
|
|
{ 0x9C18, "IssueTuningCommandSoftwareTimeout" },
|
|
{ 0x9E18, "TuningFailed" },
|
|
{ 0xA018, "MmcInitializationSoftwareTimeout" },
|
|
{ 0xA218, "MmcNotSupportExtendedCsd" },
|
|
{ 0xA418, "UnexpectedMmcExtendedCsdValue" },
|
|
{ 0xA618, "MmcEraseSoftwareTimeout" },
|
|
{ 0xA818, "SdCardValidationError" },
|
|
{ 0xAA18, "SdCardInitializationSoftwareTimeout" },
|
|
{ 0xAC18, "SdCardGetValidRcaSoftwareTimeout" },
|
|
{ 0xAE18, "UnexpectedSdCardAcmdDisabled" },
|
|
{ 0xB018, "SdCardNotSupportSwitchFunctionStatus" },
|
|
{ 0xB218, "UnexpectedSdCardSwitchFunctionStatus" },
|
|
{ 0xB418, "SdCardNotSupportAccessMode" },
|
|
{ 0xB618, "SdCardNot4BitBusWidthAtUhsIMode" },
|
|
{ 0xB818, "SdCardNotSupportSdr104AndSdr50" },
|
|
{ 0xBA18, "SdCardCannotSwitchAccessMode" },
|
|
{ 0xBC18, "SdCardFailedSwitchAccessMode" },
|
|
{ 0xBE18, "SdCardUnacceptableCurrentConsumption" },
|
|
{ 0xC018, "SdCardNotReadyToVoltageSwitch" },
|
|
{ 0xC218, "SdCardNotCompleteVoltageSwitch" },
|
|
{ 0x10018, "HostControllerUnexpected" },
|
|
{ 0x10218, "InternalClockStableSoftwareTimeout" },
|
|
{ 0x10418, "SdHostStandardUnknownAutoCmdError" },
|
|
{ 0x10618, "SdHostStandardUnknownError" },
|
|
{ 0x10818, "SdmmcDllCalibrationSoftwareTimeout" },
|
|
{ 0x10A18, "SdmmcDllApplicationSoftwareTimeout" },
|
|
{ 0x10C18, "SdHostStandardFailSwitchTo18V" },
|
|
{ 0x10E18, "DriveStrengthCalibrationNotCompleted" },
|
|
{ 0x11018, "DriveStrengthCalibrationSoftwareTimeout" },
|
|
{ 0x11218, "SdmmcCompShortToGnd" },
|
|
{ 0x11418, "SdmmcCompOpen" },
|
|
{ 0x14018, "InternalError" },
|
|
{ 0x14218, "NoWaitedInterrupt" },
|
|
{ 0x14418, "WaitInterruptSoftwareTimeout" },
|
|
{ 0x18018, "AbortCommandIssued" },
|
|
{ 0x19018, "NotSupported" },
|
|
{ 0x19218, "NotImplemented" },
|
|
{ 0x1A, "SecureMonitorError" },
|
|
{ 0x21A, "SecureMonitorNotImplemented" },
|
|
{ 0x41A, "SecureMonitorInvalidArgument" },
|
|
{ 0x61A, "SecureMonitorBusy" },
|
|
{ 0x81A, "SecureMonitorNoAsyncOperation" },
|
|
{ 0xA1A, "SecureMonitorInvalidAsyncOperation" },
|
|
{ 0xC1A, "SecureMonitorNotPermitted" },
|
|
{ 0xE1A, "SecureMonitorNotInitialized" },
|
|
{ 0xC81A, "InvalidSize" },
|
|
{ 0xCA1A, "UnknownSecureMonitorError" },
|
|
{ 0xCC1A, "DecryptionFailed" },
|
|
{ 0xD01A, "OutOfKeySlots" },
|
|
{ 0xD21A, "InvalidKeySlot" },
|
|
{ 0xD41A, "BootReasonAlreadySet" },
|
|
{ 0xD61A, "BootReasonNotSet" },
|
|
{ 0xD81A, "InvalidArgument" },
|
|
{ 0x21B, "InsufficientProvidedMemory" },
|
|
{ 0x21D, "ConnectionFailure" },
|
|
{ 0x61D, "UnknownDriverType" },
|
|
{ 0xA1D, "NonBlockingReceiveFailed" },
|
|
{ 0x101D, "ChannelWaitCancelled" },
|
|
{ 0x121D, "ChannelAlreadyExist" },
|
|
{ 0x141D, "ChannelNotExist" },
|
|
{ 0x12E1D, "OutOfChannel" },
|
|
{ 0x1301D, "OutOfTask" },
|
|
{ 0x1901D, "InvalidChannelState" },
|
|
{ 0x1921D, "InvalidChannelStateDisconnected" },
|
|
{ 0x7D01D, "InternalError" },
|
|
{ 0x7D21D, "Overflow" },
|
|
{ 0x7D41D, "OutOfMemory" },
|
|
{ 0x7D61D, "InvalidArgument" },
|
|
{ 0x7D81D, "ProtocolError" },
|
|
{ 0x7DA1D, "Cancelled" },
|
|
{ 0x8981D, "MuxError" },
|
|
{ 0x89A1D, "ChannelBufferOverflow" },
|
|
{ 0x89C1D, "ChannelBufferHasNotEnoughData" },
|
|
{ 0x89E1D, "ChannelVersionNotMatched" },
|
|
{ 0x8A01D, "ChannelStateTransitionError" },
|
|
{ 0x8A41D, "ChannelReceiveBufferEmpty" },
|
|
{ 0x8A61D, "ChannelSequenceIdNotMatched" },
|
|
{ 0x8A81D, "ChannelCannotDiscard" },
|
|
{ 0x9601D, "DriverError" },
|
|
{ 0x9621D, "DriverOpened" },
|
|
{ 0xA281D, "SocketDriverError" },
|
|
{ 0xA2A1D, "SocketSocketExemptError" },
|
|
{ 0xA2C1D, "SocketBindError" },
|
|
{ 0xA301D, "SocketListenError" },
|
|
{ 0xA321D, "SocketAcceptError" },
|
|
{ 0xA341D, "SocketReceiveError" },
|
|
{ 0xA361D, "SocketSendError" },
|
|
{ 0xA381D, "SocketReceiveFromError" },
|
|
{ 0xA3A1D, "SocketSendToError" },
|
|
{ 0xA3C1D, "SocketSetSockOptError" },
|
|
{ 0xA3E1D, "SocketGetSockNameError" },
|
|
{ 0xAF01D, "UsbDriverError" },
|
|
{ 0xAF21D, "UsbDriverUnknownError" },
|
|
{ 0xAF41D, "UsbDriverBusyError" },
|
|
{ 0xAF61D, "UsbDriverReceiveError" },
|
|
{ 0xAF81D, "UsbDriverSendError" },
|
|
{ 0xFA01D, "HtcctrlError" },
|
|
{ 0xFA21D, "HtcctrlStateTransitionNotAllowed" },
|
|
{ 0xFA41D, "HtcctrlReceiveUnexpectedPacket" },
|
|
{ 0x21E, "OutOfResource" },
|
|
{ 0x41E, "NotSupported" },
|
|
{ 0x61E, "InvalidArgument" },
|
|
{ 0x81E, "PermissionDenied" },
|
|
{ 0xA1E, "AccessModeDenied" },
|
|
{ 0xC1E, "DeviceCodeNotFound" },
|
|
{ 0x61F, "InvalidArgument" },
|
|
{ 0xC81F, "ConnectionFailure" },
|
|
{ 0xCA1F, "HtclowChannelClosed" },
|
|
{ 0xDC1F, "UnexpectedResponse" },
|
|
{ 0xDE1F, "UnexpectedResponseProtocolId" },
|
|
{ 0xE01F, "UnexpectedResponseProtocolVersion" },
|
|
{ 0xE21F, "UnexpectedResponsePacketCategory" },
|
|
{ 0xE41F, "UnexpectedResponsePacketType" },
|
|
{ 0xE61F, "UnexpectedResponseBodySize" },
|
|
{ 0xE81F, "UnexpectedResponseBody" },
|
|
{ 0x1901F, "InternalError" },
|
|
{ 0x1921F, "InvalidSize" },
|
|
{ 0x1A61F, "UnknownError" },
|
|
{ 0x1A81F, "UnsupportedProtocolVersion" },
|
|
{ 0x1AA1F, "InvalidRequest" },
|
|
{ 0x1AC1F, "InvalidHandle" },
|
|
{ 0x1AE1F, "OutOfHandle" },
|
|
{ 0x265, "NoAck" },
|
|
{ 0x465, "BusBusy" },
|
|
{ 0x665, "CommandListFull" },
|
|
{ 0xA65, "UnknownDevice" },
|
|
{ 0x1FA65, "Timeout" },
|
|
{ 0x266, "AlreadyBound" },
|
|
{ 0x466, "AlreadyOpen" },
|
|
{ 0x666, "DeviceNotFound" },
|
|
{ 0x866, "InvalidArgument" },
|
|
{ 0xC66, "NotOpen" },
|
|
{ 0x1669, "SettingsItemNotFound" },
|
|
{ 0xC869, "InternalError" },
|
|
{ 0xCA69, "SettingsItemKeyAllocationFailed" },
|
|
{ 0xCC69, "SettingsItemValueAllocationFailed" },
|
|
{ 0x19069, "InvalidArgument" },
|
|
{ 0x19269, "SettingsNameNull" },
|
|
{ 0x19469, "SettingsItemKeyNull" },
|
|
{ 0x19669, "SettingsItemValueNull" },
|
|
{ 0x19869, "SettingsItemKeyBufferNull" },
|
|
{ 0x19A69, "SettingsItemValueBufferNull" },
|
|
{ 0x1BA69, "SettingsNameEmpty" },
|
|
{ 0x1BC69, "SettingsItemKeyEmpty" },
|
|
{ 0x1E269, "SettingsNameTooLong" },
|
|
{ 0x1E469, "SettingsItemKeyTooLong" },
|
|
{ 0x20A69, "SettingsNameInvalidFormat" },
|
|
{ 0x20C69, "SettingsItemKeyInvalidFormat" },
|
|
{ 0x20E69, "SettingsItemValueInvalidFormat" },
|
|
{ 0x48869, "CalibrationDataError" },
|
|
{ 0x48A69, "CalibrationDataFileSystemCorrupted" },
|
|
{ 0x48C69, "CalibrationDataCrcError" },
|
|
{ 0x48E69, "CalibrationDataShaError" },
|
|
{ 0x272, "OperationFailed" },
|
|
{ 0xC72, "NotSupported" },
|
|
{ 0xE72, "NotFound" },
|
|
{ 0x74, "NotInitialized" },
|
|
{ 0x274, "NoCapability" },
|
|
{ 0xCC74, "OffsetInvalid" },
|
|
{ 0xCE74, "UninitializedClock" },
|
|
{ 0x19074, "NotComparable" },
|
|
{ 0x19274, "Overflowed" },
|
|
{ 0x64274, "OutOfMemory" },
|
|
{ 0x70874, "InvalidArgument" },
|
|
{ 0x70A74, "InvalidPointer" },
|
|
{ 0x70C74, "OutOfRange" },
|
|
{ 0x70E74, "InvalidTimeZoneBinary" },
|
|
{ 0x7BA74, "NotFound" },
|
|
{ 0x7BC74, "NotImplemented" },
|
|
{ 0x27A, "InvalidArgument" },
|
|
{ 0x47A, "NotFound" },
|
|
{ 0x67A, "TargetLocked" },
|
|
{ 0x87A, "TargetAlreadyMounted" },
|
|
{ 0xA7A, "TargetNotMounted" },
|
|
{ 0xC7A, "AlreadyOpen" },
|
|
{ 0xE7A, "NotOpen" },
|
|
{ 0x107A, "InternetRequestDenied" },
|
|
{ 0x127A, "ServiceOpenLimitReached" },
|
|
{ 0x147A, "SaveDataNotFound" },
|
|
{ 0x3E7A, "NetworkServiceAccountNotAvailable" },
|
|
{ 0xA07A, "PassphrasePathNotFound" },
|
|
{ 0xA27A, "DataVerificationFailed" },
|
|
{ 0xB47A, "PermissionDenied" },
|
|
{ 0xB67A, "AllocationFailed" },
|
|
{ 0xC47A, "InvalidOperation" },
|
|
{ 0x1987A, "InvalidDeliveryCacheStorageFile" },
|
|
{ 0x19A7A, "StorageOpenLimitReached" },
|
|
{ 0x7B, "SslService" },
|
|
{ 0x7C, "Cancelled" },
|
|
{ 0x27C, "CancelledByUser" },
|
|
{ 0xC87C, "UserNotExist" },
|
|
{ 0x1907C, "NetworkServiceAccountUnavailable" },
|
|
{ 0x35C7C, "TokenCacheUnavailable" },
|
|
{ 0x17707C, "NetworkCommunicationError" },
|
|
{ 0x2085, "IllegalRequest" },
|
|
{ 0x8C89, "HttpConnectionCanceled" },
|
|
{ 0x48A, "AlreadyInitialized" },
|
|
{ 0x68A, "NotInitialized" },
|
|
{ 0x8C, "NotInitialized" },
|
|
{ 0x28C, "AlreadyInitialized" },
|
|
{ 0xC88C, "InvalidParameter" },
|
|
{ 0xCE8C, "AlignmentError" },
|
|
{ 0x1928C, "OperationDenied" },
|
|
{ 0x1948C, "MemAllocFailure" },
|
|
{ 0x19C8C, "ResourceBusy" },
|
|
{ 0x19E8C, "InternalStateError" },
|
|
{ 0x3228C, "TransactionError" },
|
|
{ 0x3328C, "Interrupted" },
|
|
{ 0x293, "NotInitialized" },
|
|
{ 0x493, "AlreadyInitialized" },
|
|
{ 0x693, "OutOfArraySpace" },
|
|
{ 0x893, "OutOfFieldSpace" },
|
|
{ 0xA93, "OutOfMemory" },
|
|
{ 0xC93, "NotSupported" },
|
|
{ 0xE93, "InvalidArgument" },
|
|
{ 0x1093, "NotFound" },
|
|
{ 0x1293, "FieldCategoryMismatch" },
|
|
{ 0x1493, "FieldTypeMismatch" },
|
|
{ 0x1693, "AlreadyExists" },
|
|
{ 0x1893, "CorruptJournal" },
|
|
{ 0x1A93, "CategoryNotFound" },
|
|
{ 0x1C93, "RequiredContextMissing" },
|
|
{ 0x1E93, "RequiredFieldMissing" },
|
|
{ 0x2093, "FormatterError" },
|
|
{ 0x2293, "InvalidPowerState" },
|
|
{ 0x2493, "ArrayFieldTooLarge" },
|
|
{ 0x2693, "AlreadyOwned" },
|
|
{ 0x49E, "BootImagePackageNotFound" },
|
|
{ 0x69E, "InvalidBootImagePackage" },
|
|
{ 0x89E, "TooSmallWorkBuffer" },
|
|
{ 0xA9E, "NotAlignedWorkBuffer" },
|
|
{ 0xC9E, "NeedsRepairBootImages" },
|
|
{ 0x2A2, "ApplicationAborted" },
|
|
{ 0x4A2, "SystemModuleAborted" },
|
|
{ 0x2A3, "AllocationFailed" },
|
|
{ 0x4A3, "NullGraphicsBuffer" },
|
|
{ 0x6A3, "AlreadyThrown" },
|
|
{ 0x8A3, "TooManyEvents" },
|
|
{ 0xAA3, "InRepairWithoutVolHeld" },
|
|
{ 0xCA3, "InRepairWithoutTimeReviserCartridge" },
|
|
{ 0xA8, "UndefinedInstruction" },
|
|
{ 0x2A8, "InstructionAbort" },
|
|
{ 0x4A8, "DataAbort" },
|
|
{ 0x6A8, "AlignmentFault" },
|
|
{ 0x8A8, "DebuggerAttached" },
|
|
{ 0xAA8, "BreakPoint" },
|
|
{ 0xCA8, "UserBreak" },
|
|
{ 0xEA8, "DebuggerBreak" },
|
|
{ 0x10A8, "UndefinedSystemCall" },
|
|
{ 0x12A8, "MemorySystemError" },
|
|
{ 0xC6A8, "IncompleteReport" },
|
|
{ 0x2B7, "CannotDebug" },
|
|
{ 0x4B7, "AlreadyAttached" },
|
|
{ 0x6B7, "Cancelled" },
|
|
{ 0x4BD, "InvalidArgument" },
|
|
{ 0x2C6, "NotSupported" },
|
|
{ 0x4C6, "InvalidArgument" },
|
|
{ 0x6C6, "NotAvailable" },
|
|
{ 0xCAC6, "CalibrationDataCrcError" },
|
|
{ 0x118CA, "Invalid" },
|
|
{ 0x4B2CA, "DualConnected" },
|
|
{ 0x4B4CA, "SameJoyTypeConnected" },
|
|
{ 0x4B6CA, "ColorNotAvailable" },
|
|
{ 0x4B8CA, "ControllerNotConnected" },
|
|
{ 0x183ACA, "Canceled" },
|
|
{ 0x183CCA, "NotSupportedNpadStyle" },
|
|
{ 0x1900CA, "ControllerFirmwareUpdateError" },
|
|
{ 0x1902CA, "ControllerFirmwareUpdateFailed" },
|
|
{ 0x4CC, "UnknownCommand" },
|
|
{ 0x8CC, "OutOfResource" },
|
|
{ 0xECC, "NoSocket" },
|
|
{ 0xDCCD, "IrsensorUnavailable" },
|
|
{ 0xDECD, "IrsensorUnsupported" },
|
|
{ 0xF0CD, "IrsensorNotReady" },
|
|
{ 0xF4CD, "IrsensorDeviceError" },
|
|
{ 0x4CE, "AlbumError" },
|
|
{ 0x6CE, "AlbumWorkMemoryError" },
|
|
{ 0xECE, "AlbumAlreadyOpened" },
|
|
{ 0x10CE, "AlbumOutOfRange" },
|
|
{ 0x14CE, "AlbumInvalidFileId" },
|
|
{ 0x16CE, "AlbumInvalidApplicationId" },
|
|
{ 0x18CE, "AlbumInvalidTimestamp" },
|
|
{ 0x1ACE, "AlbumInvalidStorage" },
|
|
{ 0x1CCE, "AlbumInvalidFileContents" },
|
|
{ 0x2ACE, "AlbumIsNotMounted" },
|
|
{ 0x2CCE, "AlbumIsFull" },
|
|
{ 0x2ECE, "AlbumFileNotFound" },
|
|
{ 0x30CE, "AlbumInvalidFileData" },
|
|
{ 0x32CE, "AlbumFileCountLimit" },
|
|
{ 0x34CE, "AlbumFileNoThumbnail" },
|
|
{ 0x3CCE, "AlbumReadBufferShortage" },
|
|
{ 0xB4CE, "AlbumFileSystemError" },
|
|
{ 0xBCCE, "AlbumAccessCorrupted" },
|
|
{ 0xC0CE, "AlbumDestinationAccessCorrupted" },
|
|
{ 0x640CE, "ControlError" },
|
|
{ 0x668CE, "ControlResourceLimit" },
|
|
{ 0x66CCE, "ControlNotOpened" },
|
|
{ 0x7FECE, "NotSupported" },
|
|
{ 0x800CE, "InternalError" },
|
|
{ 0x974CE, "InternalJpegEncoderError" },
|
|
{ 0x978CE, "InternalJpegWorkMemoryShortage" },
|
|
{ 0xA28CE, "InternalFileDataVerificationError" },
|
|
{ 0xA2ACE, "InternalFileDataVerificationEmptyFileData" },
|
|
{ 0xA2CCE, "InternalFileDataVerificationExifExtractionFailed" },
|
|
{ 0xA2ECE, "InternalFileDataVerificationExifAnalyzationFailed" },
|
|
{ 0xA30CE, "InternalFileDataVerificationDateTimeExtractionFailed" },
|
|
{ 0xA32CE, "InternalFileDataVerificationInvalidDateTimeLength" },
|
|
{ 0xA34CE, "InternalFileDataVerificationInconsistentDateTime" },
|
|
{ 0xA36CE, "InternalFileDataVerificationMakerNoteExtractionFailed" },
|
|
{ 0xA38CE, "InternalFileDataVerificationInconsistentApplicationId" },
|
|
{ 0xA3ACE, "InternalFileDataVerificationInconsistentSignature" },
|
|
{ 0xA3CCE, "InternalFileDataVerificationUnsupportedOrientation" },
|
|
{ 0xA3ECE, "InternalFileDataVerificationInvalidDataDimension" },
|
|
{ 0xA40CE, "InternalFileDataVerificationInconsistentOrientation" },
|
|
{ 0xAF0CE, "InternalAlbumLimitationError" },
|
|
{ 0xAF2CE, "InternalAlbumLimitationFileCountLimit" },
|
|
{ 0xBB8CE, "InternalSignatureError" },
|
|
{ 0xBBACE, "InternalSignatureExifExtractionFailed" },
|
|
{ 0xBBCCE, "InternalSignatureMakerNoteExtractionFailed" },
|
|
{ 0xD48CE, "InternalAlbumSessionError" },
|
|
{ 0xD4ACE, "InternalAlbumLimitationSessionCountLimit" },
|
|
{ 0xED8CE, "InternalAlbumTemporaryFileError" },
|
|
{ 0xEDACE, "InternalAlbumTemporaryFileCountLimit" },
|
|
{ 0xEDCCE, "InternalAlbumTemporaryFileCreateError" },
|
|
{ 0xEDECE, "InternalAlbumTemporaryFileCreateRetryCountLimit" },
|
|
{ 0xEE0CE, "InternalAlbumTemporaryFileOpenError" },
|
|
{ 0xEE2CE, "InternalAlbumTemporaryFileGetFileSizeError" },
|
|
{ 0xEE4CE, "InternalAlbumTemporaryFileSetFileSizeError" },
|
|
{ 0xEE6CE, "InternalAlbumTemporaryFileReadFileError" },
|
|
{ 0xEE8CE, "InternalAlbumTemporaryFileWriteFileError" },
|
|
{ 0x2E4, "NotImplemented" },
|
|
{ 0x4E4, "NotAvailable" },
|
|
{ 0x6E4, "ApplicationNotRunning" },
|
|
{ 0x8E4, "BufferNotEnough" },
|
|
{ 0xAE4, "ApplicationContentNotFound" },
|
|
{ 0xCE4, "ContentMetaNotFound" },
|
|
{ 0xEE4, "OutOfMemory" },
|
|
{ 0x3AC, "InvalidArgument" },
|
|
{ 0x5AC, "NullArgument" },
|
|
{ 0x7AC, "ArgumentOutOfRange" },
|
|
{ 0x9AC, "BufferTooSmall" },
|
|
{ 0x67AC, "ServiceNotInitialized" },
|
|
{ 0xCBAC, "NotImplemented" },
|
|
{ 0x7D1AC, "InvalidData" },
|
|
{ 0x7D3AC, "InvalidInitialProcessData" },
|
|
{ 0x7D5AC, "InvalidKip" },
|
|
{ 0x7D7AC, "InvalidKipFileSize" },
|
|
{ 0x7D9AC, "InvalidKipMagic" },
|
|
{ 0x7DBAC, "InvalidKipSegmentSize" },
|
|
{ 0x7DDAC, "KipSegmentDecompressionFailed" },
|
|
{ 0x7E5AC, "InvalidIni" },
|
|
{ 0x7E7AC, "InvalidIniFileSize" },
|
|
{ 0x7E9AC, "InvalidIniMagic" },
|
|
{ 0x7EBAC, "InvalidIniProcessCount" },
|
|
{ 0x7F9AC, "InvalidPackage2" },
|
|
{ 0x7FBAC, "InvalidPackage2HeaderSignature" },
|
|
{ 0x7FDAC, "InvalidPackage2MetaSizeA" },
|
|
{ 0x7FFAC, "InvalidPackage2MetaSizeB" },
|
|
{ 0x801AC, "InvalidPackage2MetaKeyGeneration" },
|
|
{ 0x803AC, "InvalidPackage2MetaMagic" },
|
|
{ 0x805AC, "InvalidPackage2MetaEntryPointAlignment" },
|
|
{ 0x807AC, "InvalidPackage2MetaPayloadAlignment" },
|
|
{ 0x809AC, "InvalidPackage2MetaPayloadSizeAlignment" },
|
|
{ 0x80BAC, "InvalidPackage2MetaTotalSize" },
|
|
{ 0x80DAC, "InvalidPackage2MetaPayloadSize" },
|
|
{ 0x80FAC, "InvalidPackage2MetaPayloadsOverlap" },
|
|
{ 0x811AC, "InvalidPackage2MetaEntryPointNotFound" },
|
|
{ 0x813AC, "InvalidPackage2PayloadCorrupted" },
|
|
{ 0x821AC, "InvalidPackage1" },
|
|
{ 0x823AC, "InvalidPackage1SectionSize" },
|
|
{ 0x825AC, "InvalidPackage1MarikoBodySize" },
|
|
{ 0x827AC, "InvalidPackage1Pk11Size" },
|
|
};
|
|
|
|
public static bool TryGet(int errorCode, out string name)
|
|
{
|
|
return _names.TryGetValue(errorCode, out name);
|
|
}
|
|
}
|
|
}
|