mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-09-19 17:49:46 +02:00
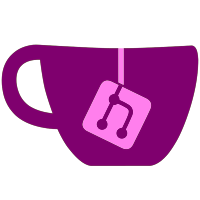
* Rename enum fields
* Naming conventions
* Remove unneeded ".this"
* Remove unneeded semicolons
* Remove unused Usings
* Don't use var
* Remove unneeded enum underlying types
* Explicitly label class visibility
* Remove unneeded @ prefixes
* Remove unneeded commas
* Remove unneeded if expressions
* Method doesn't use unsafe code
* Remove unneeded casts
* Initialized objects don't need an empty constructor
* Remove settings from DotSettings
* Revert "Explicitly label class visibility"
This reverts commit ad5eb5787c
.
* Small changes
* Revert external enum renaming
* Changes from feedback
* Apply previous refactorings to the merged code
59 lines
1.6 KiB
C#
59 lines
1.6 KiB
C#
using Ryujinx.Common.Logging;
|
|
using Ryujinx.HLE.HOS.Ipc;
|
|
using System;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
using System.Net;
|
|
using System.Net.NetworkInformation;
|
|
using System.Net.Sockets;
|
|
|
|
using static Ryujinx.HLE.HOS.ErrorCode;
|
|
|
|
namespace Ryujinx.HLE.HOS.Services.Nifm
|
|
{
|
|
class IGeneralService : IpcService
|
|
{
|
|
private Dictionary<int, ServiceProcessRequest> _commands;
|
|
|
|
public override IReadOnlyDictionary<int, ServiceProcessRequest> Commands => _commands;
|
|
|
|
public IGeneralService()
|
|
{
|
|
_commands = new Dictionary<int, ServiceProcessRequest>
|
|
{
|
|
{ 4, CreateRequest },
|
|
{ 12, GetCurrentIpAddress }
|
|
};
|
|
}
|
|
|
|
public long CreateRequest(ServiceCtx context)
|
|
{
|
|
int unknown = context.RequestData.ReadInt32();
|
|
|
|
MakeObject(context, new IRequest(context.Device.System));
|
|
|
|
Logger.PrintStub(LogClass.ServiceNifm, "Stubbed.");
|
|
|
|
return 0;
|
|
}
|
|
|
|
public long GetCurrentIpAddress(ServiceCtx context)
|
|
{
|
|
if (!NetworkInterface.GetIsNetworkAvailable())
|
|
{
|
|
return MakeError(ErrorModule.Nifm, NifmErr.NoInternetConnection);
|
|
}
|
|
|
|
IPHostEntry host = Dns.GetHostEntry(Dns.GetHostName());
|
|
|
|
IPAddress address = host.AddressList.FirstOrDefault(a => a.AddressFamily == AddressFamily.InterNetwork);
|
|
|
|
context.ResponseData.Write(BitConverter.ToUInt32(address.GetAddressBytes()));
|
|
|
|
Logger.PrintInfo(LogClass.ServiceNifm, $"Console's local IP is \"{address}\".");
|
|
|
|
return 0;
|
|
}
|
|
}
|
|
}
|