mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-09-19 01:29:45 +02:00
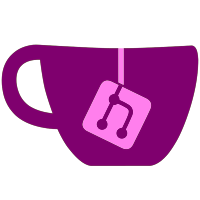
* Initial implementation of KProcess * Some improvements to the memory manager, implement back guest stack trace printing * Better GetInfo implementation, improve checking in some places with information from process capabilities * Allow the cpu to read/write from the correct memory locations for accesses crossing a page boundary * Change long -> ulong for address/size on memory related methods to avoid unnecessary casts * Attempt at implementing ldr:ro with new KProcess * Allow BSS with size 0 on ldr:ro * Add checking for memory block slab heap usage, return errors if full, exit gracefully * Use KMemoryBlockSize const from KMemoryManager * Allow all methods to read from non-contiguous locations * Fix for TransactParcelAuto * Address PR feedback, additionally fix some small issues related to the KIP loader and implement SVCs GetProcessId, GetProcessList, GetSystemInfo, CreatePort and ManageNamedPort * Fix wrong check for source pages count from page list on MapPhysicalMemory * Fix some issues with UnloadNro on ldr:ro
56 lines
1.3 KiB
C#
56 lines
1.3 KiB
C#
using OpenTK.Graphics.OpenGL;
|
|
using System;
|
|
|
|
namespace Ryujinx.Graphics.Gal.OpenGL
|
|
{
|
|
class OGLStreamBuffer : IDisposable
|
|
{
|
|
public int Handle { get; protected set; }
|
|
|
|
public long Size { get; protected set; }
|
|
|
|
protected BufferTarget Target { get; private set; }
|
|
|
|
public OGLStreamBuffer(BufferTarget Target, long Size)
|
|
{
|
|
this.Target = Target;
|
|
this.Size = Size;
|
|
|
|
Handle = GL.GenBuffer();
|
|
|
|
GL.BindBuffer(Target, Handle);
|
|
|
|
GL.BufferData(Target, (IntPtr)Size, IntPtr.Zero, BufferUsageHint.StreamDraw);
|
|
}
|
|
|
|
public void SetData(long Size, IntPtr HostAddress)
|
|
{
|
|
GL.BindBuffer(Target, Handle);
|
|
|
|
GL.BufferSubData(Target, IntPtr.Zero, (IntPtr)Size, HostAddress);
|
|
}
|
|
|
|
public void SetData(byte[] Data)
|
|
{
|
|
GL.BindBuffer(Target, Handle);
|
|
|
|
GL.BufferSubData(Target, IntPtr.Zero, (IntPtr)Data.Length, Data);
|
|
}
|
|
|
|
public void Dispose()
|
|
{
|
|
Dispose(true);
|
|
}
|
|
|
|
protected virtual void Dispose(bool Disposing)
|
|
{
|
|
if (Disposing && Handle != 0)
|
|
{
|
|
GL.DeleteBuffer(Handle);
|
|
|
|
Handle = 0;
|
|
}
|
|
}
|
|
}
|
|
}
|