mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-09-20 18:19:52 +02:00
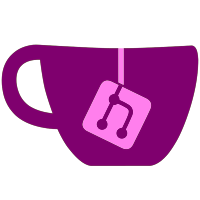
* IPC refactor part 2: Use ReplyAndReceive on HLE services and remove special handling from kernel * Fix for applet transfer memory + some nits * Keep handles if possible to avoid server handle table exhaustion * Fix IPC ZeroFill bug * am: Correctly implement CreateManagedDisplayLayer and implement CreateManagedDisplaySeparableLayer CreateManagedDisplaySeparableLayer is requires since 10.x+ when appletResourceUserId != 0 * Make it exit properly * Make ServiceNotImplementedException show the full message again * Allow yielding execution to avoid starving other threads * Only wait if active * Merge IVirtualMemoryManager and IAddressSpaceManager * Fix Ro loading data from the wrong process Co-authored-by: Thog <me@thog.eu>
61 lines
2.2 KiB
C#
61 lines
2.2 KiB
C#
using Ryujinx.Common.Logging;
|
|
using Ryujinx.HLE.HOS.Kernel.Memory;
|
|
using Ryujinx.HLE.HOS.Services.Am.AppletAE.AllSystemAppletProxiesService.LibraryAppletCreator;
|
|
|
|
namespace Ryujinx.HLE.HOS.Services.Am.AppletAE.AllSystemAppletProxiesService.SystemAppletProxy
|
|
{
|
|
class ILibraryAppletCreator : IpcService
|
|
{
|
|
public ILibraryAppletCreator() { }
|
|
|
|
[Command(0)]
|
|
// CreateLibraryApplet(u32, u32) -> object<nn::am::service::ILibraryAppletAccessor>
|
|
public ResultCode CreateLibraryApplet(ServiceCtx context)
|
|
{
|
|
AppletId appletId = (AppletId)context.RequestData.ReadInt32();
|
|
int libraryAppletMode = context.RequestData.ReadInt32();
|
|
|
|
MakeObject(context, new ILibraryAppletAccessor(appletId, context.Device.System));
|
|
|
|
return ResultCode.Success;
|
|
}
|
|
|
|
[Command(10)]
|
|
// CreateStorage(u64) -> object<nn::am::service::IStorage>
|
|
public ResultCode CreateStorage(ServiceCtx context)
|
|
{
|
|
long size = context.RequestData.ReadInt64();
|
|
|
|
MakeObject(context, new IStorage(new byte[size]));
|
|
|
|
return ResultCode.Success;
|
|
}
|
|
|
|
[Command(11)]
|
|
// CreateTransferMemoryStorage(b8, u64, handle<copy>) -> object<nn::am::service::IStorage>
|
|
public ResultCode CreateTransferMemoryStorage(ServiceCtx context)
|
|
{
|
|
bool unknown = context.RequestData.ReadBoolean();
|
|
long size = context.RequestData.ReadInt64();
|
|
int handle = context.Request.HandleDesc.ToCopy[0];
|
|
|
|
KTransferMemory transferMem = context.Process.HandleTable.GetObject<KTransferMemory>(handle);
|
|
|
|
if (transferMem == null)
|
|
{
|
|
Logger.Warning?.Print(LogClass.ServiceAm, $"Invalid TransferMemory Handle: {handle:X}");
|
|
|
|
return ResultCode.Success; // TODO: Find correct error code
|
|
}
|
|
|
|
var data = new byte[transferMem.Size];
|
|
transferMem.Creator.CpuMemory.Read(transferMem.Address, data);
|
|
|
|
context.Device.System.KernelContext.Syscall.CloseHandle(handle);
|
|
|
|
MakeObject(context, new IStorage(data));
|
|
|
|
return ResultCode.Success;
|
|
}
|
|
}
|
|
} |