mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-09-17 08:44:49 +02:00
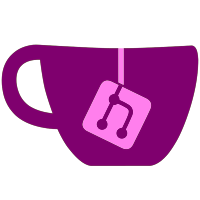
* Renaming part 1 * Renaming part 2 * Renaming part 3 * Renaming part 4 * Renaming part 5 * Renaming part 6 * Renaming part 7 * Renaming part 8 * Renaming part 9 * Renaming part 10 * General cleanup * Thought I got all of these * Apply #595 * Additional renaming * Tweaks from feedback * Rename files
54 lines
1.7 KiB
C#
54 lines
1.7 KiB
C#
using ChocolArm64.Memory;
|
|
using Ryujinx.Common;
|
|
using Ryujinx.Graphics.Gal;
|
|
using Ryujinx.Graphics.Memory;
|
|
|
|
namespace Ryujinx.Graphics.Texture
|
|
{
|
|
static class TextureHelper
|
|
{
|
|
public static ISwizzle GetSwizzle(GalImage image)
|
|
{
|
|
int blockWidth = ImageUtils.GetBlockWidth (image.Format);
|
|
int blockHeight = ImageUtils.GetBlockHeight (image.Format);
|
|
int blockDepth = ImageUtils.GetBlockDepth (image.Format);
|
|
int bytesPerPixel = ImageUtils.GetBytesPerPixel(image.Format);
|
|
|
|
int width = BitUtils.DivRoundUp(image.Width, blockWidth);
|
|
int height = BitUtils.DivRoundUp(image.Height, blockHeight);
|
|
int depth = BitUtils.DivRoundUp(image.Depth, blockDepth);
|
|
|
|
if (image.Layout == GalMemoryLayout.BlockLinear)
|
|
{
|
|
int alignMask = image.TileWidth * (64 / bytesPerPixel) - 1;
|
|
|
|
width = (width + alignMask) & ~alignMask;
|
|
|
|
return new BlockLinearSwizzle(
|
|
width,
|
|
height,
|
|
depth,
|
|
image.GobBlockHeight,
|
|
image.GobBlockDepth,
|
|
bytesPerPixel);
|
|
}
|
|
else
|
|
{
|
|
return new LinearSwizzle(image.Pitch, bytesPerPixel, width, height);
|
|
}
|
|
}
|
|
|
|
public static (MemoryManager Memory, long Position) GetMemoryAndPosition(
|
|
IMemory memory,
|
|
long position)
|
|
{
|
|
if (memory is NvGpuVmm vmm)
|
|
{
|
|
return (vmm.Memory, vmm.GetPhysicalAddress(position));
|
|
}
|
|
|
|
return ((MemoryManager)memory, position);
|
|
}
|
|
}
|
|
}
|