mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-09-19 17:49:46 +02:00
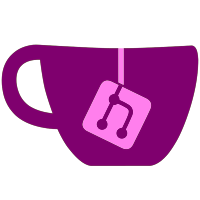
* Use savedata FS commands from LibHac * Add EnsureSaveData. Use ApplicationControlProperty struct * Add a function to migrate to the new directory layout * LibHac update * Change backup structure * Don't create UI files in the save path * Update RyuFs paths * Add GetProgramIndexForAccessLog Ryujinx only runs one program at a time, so always return values reflecting that * Load control NCA when loading from an NSP * Skip over UI stats when exiting * Set TitleName and TitleId in more cases. Fix TitleID naming style * Completely comment out GUI play stats code * rebase * Update LibHac * Update LibHac * Revert UI changes * Do migration automatically at startup * Rename RyuFs directory to Ryujinx * Update RyuFs text * Store savedata paths in the GUI * Make "Open Save Directory" work * Use a dummy NACP in EnsureSaveData if one is not loaded * Remove manual migration button * Respond to feedback * Don't read the installer config to get a version string * Delete nuget.config * Exclude 'sdcard' and 'bis' during migration Co-authored-by: Thog <thog@protonmail.com>
127 lines
4.3 KiB
C#
127 lines
4.3 KiB
C#
using LibHac;
|
|
using LibHac.Fs;
|
|
using LibHac.FsSystem;
|
|
using LibHac.FsSystem.NcaUtils;
|
|
using LibHac.Spl;
|
|
using System.IO;
|
|
|
|
namespace Ryujinx.HLE.HOS.Services.Fs.FileSystemProxy
|
|
{
|
|
static class FileSystemProxyHelper
|
|
{
|
|
public static ResultCode OpenNsp(ServiceCtx context, string pfsPath, out IFileSystem openedFileSystem)
|
|
{
|
|
openedFileSystem = null;
|
|
|
|
try
|
|
{
|
|
LocalStorage storage = new LocalStorage(pfsPath, FileAccess.Read, FileMode.Open);
|
|
PartitionFileSystem nsp = new PartitionFileSystem(storage);
|
|
|
|
ImportTitleKeysFromNsp(nsp, context.Device.System.KeySet);
|
|
|
|
openedFileSystem = new IFileSystem(nsp);
|
|
}
|
|
catch (HorizonResultException ex)
|
|
{
|
|
return (ResultCode)ex.ResultValue.Value;
|
|
}
|
|
|
|
return ResultCode.Success;
|
|
}
|
|
|
|
public static ResultCode OpenNcaFs(ServiceCtx context, string ncaPath, LibHac.Fs.IStorage ncaStorage, out IFileSystem openedFileSystem)
|
|
{
|
|
openedFileSystem = null;
|
|
|
|
try
|
|
{
|
|
Nca nca = new Nca(context.Device.System.KeySet, ncaStorage);
|
|
|
|
if (!nca.SectionExists(NcaSectionType.Data))
|
|
{
|
|
return ResultCode.PartitionNotFound;
|
|
}
|
|
|
|
LibHac.Fs.IFileSystem fileSystem = nca.OpenFileSystem(NcaSectionType.Data, context.Device.System.FsIntegrityCheckLevel);
|
|
|
|
openedFileSystem = new IFileSystem(fileSystem);
|
|
}
|
|
catch (HorizonResultException ex)
|
|
{
|
|
return (ResultCode)ex.ResultValue.Value;
|
|
}
|
|
|
|
return ResultCode.Success;
|
|
}
|
|
|
|
public static ResultCode OpenFileSystemFromInternalFile(ServiceCtx context, string fullPath, out IFileSystem openedFileSystem)
|
|
{
|
|
openedFileSystem = null;
|
|
|
|
DirectoryInfo archivePath = new DirectoryInfo(fullPath).Parent;
|
|
|
|
while (string.IsNullOrWhiteSpace(archivePath.Extension))
|
|
{
|
|
archivePath = archivePath.Parent;
|
|
}
|
|
|
|
if (archivePath.Extension == ".nsp" && File.Exists(archivePath.FullName))
|
|
{
|
|
FileStream pfsFile = new FileStream(
|
|
archivePath.FullName.TrimEnd(Path.DirectorySeparatorChar),
|
|
FileMode.Open,
|
|
FileAccess.Read);
|
|
|
|
try
|
|
{
|
|
PartitionFileSystem nsp = new PartitionFileSystem(pfsFile.AsStorage());
|
|
|
|
ImportTitleKeysFromNsp(nsp, context.Device.System.KeySet);
|
|
|
|
string filename = fullPath.Replace(archivePath.FullName, string.Empty).TrimStart('\\');
|
|
|
|
Result result = nsp.OpenFile(out LibHac.Fs.IFile ncaFile, filename, OpenMode.Read);
|
|
if (result.IsFailure())
|
|
{
|
|
return (ResultCode)result.Value;
|
|
}
|
|
|
|
return OpenNcaFs(context, fullPath, ncaFile.AsStorage(), out openedFileSystem);
|
|
}
|
|
catch (HorizonResultException ex)
|
|
{
|
|
return (ResultCode)ex.ResultValue.Value;
|
|
}
|
|
}
|
|
|
|
return ResultCode.PathDoesNotExist;
|
|
}
|
|
|
|
public static void ImportTitleKeysFromNsp(LibHac.Fs.IFileSystem nsp, Keyset keySet)
|
|
{
|
|
foreach (DirectoryEntryEx ticketEntry in nsp.EnumerateEntries("/", "*.tik"))
|
|
{
|
|
Result result = nsp.OpenFile(out LibHac.Fs.IFile ticketFile, ticketEntry.FullPath, OpenMode.Read);
|
|
|
|
if (result.IsSuccess())
|
|
{
|
|
Ticket ticket = new Ticket(ticketFile.AsStream());
|
|
|
|
keySet.ExternalKeySet.Add(new RightsId(ticket.RightsId), new AccessKey(ticket.GetTitleKey(keySet)));
|
|
}
|
|
}
|
|
}
|
|
|
|
public static Result ReadFsPath(out FsPath path, ServiceCtx context, int index = 0)
|
|
{
|
|
long position = context.Request.SendBuff[index].Position;
|
|
long size = context.Request.SendBuff[index].Size;
|
|
|
|
byte[] pathBytes = context.Memory.ReadBytes(position, size);
|
|
|
|
return FsPath.FromSpan(out path, pathBytes);
|
|
}
|
|
}
|
|
}
|