mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 13:18:46 +02:00
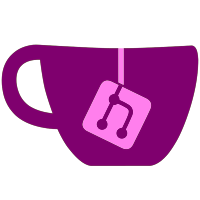
* perf: use ByteMemoryPool * feat: KPageTableBase/KPageTable new methods to read and write `ReadOnlySequence<byte>` * new: add IWritableBlock.Write(ulong, ReadOnlySequence<byte>) with default impl * perf: use GetReadOnlySequence() instead of GetSpan() * perf: make `Parcel` IDisposable, use `ByteMemoryPool` for internal allocation, and make Parcel consumers dispose of it * remove comment about copySize * remove unnecessary Clear()
28 lines
878 B
C#
28 lines
878 B
C#
using System;
|
|
using System.Buffers;
|
|
|
|
namespace Ryujinx.Memory
|
|
{
|
|
public interface IWritableBlock
|
|
{
|
|
/// <summary>
|
|
/// Writes data to CPU mapped memory, with write tracking.
|
|
/// </summary>
|
|
/// <param name="va">Virtual address to write the data into</param>
|
|
/// <param name="data">Data to be written</param>
|
|
/// <exception cref="InvalidMemoryRegionException">Throw for unhandled invalid or unmapped memory accesses</exception>
|
|
void Write(ulong va, ReadOnlySequence<byte> data)
|
|
{
|
|
foreach (ReadOnlyMemory<byte> segment in data)
|
|
{
|
|
Write(va, segment.Span);
|
|
va += (ulong)segment.Length;
|
|
}
|
|
}
|
|
|
|
void Write(ulong va, ReadOnlySpan<byte> data);
|
|
|
|
void WriteUntracked(ulong va, ReadOnlySpan<byte> data) => Write(va, data);
|
|
}
|
|
}
|