mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 13:18:46 +02:00
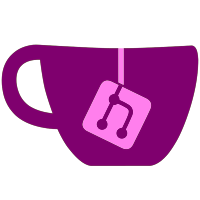
* rebase * add methods Ryyjinx.Common EmbeddedResources and SteamUtils * GAL changes - change SetData() methods and ThreadedTexture commands to use IMemoryOwner<byte> instead of SpanOrArray<byte> * Ryujinx.Graphics.Texture: change texture conversion methods to return IMemoryOwner<byte> and allocate from ByteMemoryPool * Ryujinx.Graphics.OpenGL: update ITexture and Texture-like types with SetData() methods to take IMemoryOwner<byte> instead of SpanOrArray<byte> * Ryujinx.Graphics.Vulkan: update ITexture and Texture-like types with SetData() methods to take IMemoryOwner<byte> instead of SpanOrArray<byte> * Ryujinx.Graphics.Gpu: update ITexture and Texture-like types with SetData() methods to take IMemoryOwner<byte> instead of SpanOrArray<byte> * Remove now-unused SpanOrArray<T> * post-rebase cleanup * PixelConverter: remove unsafe modifier on safe methods, and remove one unnecessary cast * use ByteMemoryPool.Rent() in GetWritableRegion() impls * fix formatting, rename `ReadRentedMemory()` to `ReadFileToRentedMemory()`` * Texture.ConvertToHostCompatibleFormat(): dispose of `result` in Astc decode branch
51 lines
2.0 KiB
C#
51 lines
2.0 KiB
C#
using System.Buffers;
|
|
|
|
namespace Ryujinx.Graphics.GAL
|
|
{
|
|
public interface ITexture
|
|
{
|
|
int Width { get; }
|
|
int Height { get; }
|
|
|
|
void CopyTo(ITexture destination, int firstLayer, int firstLevel);
|
|
void CopyTo(ITexture destination, int srcLayer, int dstLayer, int srcLevel, int dstLevel);
|
|
void CopyTo(ITexture destination, Extents2D srcRegion, Extents2D dstRegion, bool linearFilter);
|
|
void CopyTo(BufferRange range, int layer, int level, int stride);
|
|
|
|
ITexture CreateView(TextureCreateInfo info, int firstLayer, int firstLevel);
|
|
|
|
PinnedSpan<byte> GetData();
|
|
PinnedSpan<byte> GetData(int layer, int level);
|
|
|
|
/// <summary>
|
|
/// Sets the texture data. The data passed as a <see cref="IMemoryOwner{Byte}" /> will be disposed when
|
|
/// the operation completes.
|
|
/// </summary>
|
|
/// <param name="data">Texture data bytes</param>
|
|
void SetData(IMemoryOwner<byte> data);
|
|
|
|
/// <summary>
|
|
/// Sets the texture data. The data passed as a <see cref="IMemoryOwner{Byte}" /> will be disposed when
|
|
/// the operation completes.
|
|
/// </summary>
|
|
/// <param name="data">Texture data bytes</param>
|
|
/// <param name="layer">Target layer</param>
|
|
/// <param name="level">Target level</param>
|
|
void SetData(IMemoryOwner<byte> data, int layer, int level);
|
|
|
|
/// <summary>
|
|
/// Sets the texture data. The data passed as a <see cref="IMemoryOwner{Byte}" /> will be disposed when
|
|
/// the operation completes.
|
|
/// </summary>
|
|
/// <param name="data">Texture data bytes</param>
|
|
/// <param name="layer">Target layer</param>
|
|
/// <param name="level">Target level</param>
|
|
/// <param name="region">Target sub-region of the texture to update</param>
|
|
void SetData(IMemoryOwner<byte> data, int layer, int level, Rectangle<int> region);
|
|
|
|
void SetStorage(BufferRange buffer);
|
|
|
|
void Release();
|
|
}
|
|
}
|