mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 13:18:46 +02:00
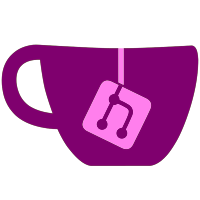
* Refactor * Apply suggestions from code review Co-authored-by: Ac_K <Acoustik666@gmail.com> * Update src/Ryujinx/UI/Views/Settings/SettingsHotkeysView.axaml.cs Co-authored-by: Ac_K <Acoustik666@gmail.com> * Update src/Ryujinx.Input/ButtonValueType.cs Co-authored-by: Ac_K <Acoustik666@gmail.com> * Add empty line * Requested renames * Update src/Ryujinx/UI/Views/Settings/SettingsHotkeysView.axaml.cs Co-authored-by: gdkchan <gab.dark.100@gmail.com> * Make parent models private readonly * Fix ControllerInputView * Make line shorter * Mac keys in locale * Double line break * Fix build * Get rid of _isValid * Fix potential race condition * Rename HasAnyButtonPressed to IsAnyButtonPressed * Use switches * Simplify enumeration --------- Co-authored-by: Ac_K <Acoustik666@gmail.com> Co-authored-by: gdkchan <gab.dark.100@gmail.com> Co-authored-by: TSR Berry <20988865+TSRBerry@users.noreply.github.com>
103 lines
2.7 KiB
C#
103 lines
2.7 KiB
C#
using Avalonia.Controls.Primitives;
|
|
using Avalonia.Threading;
|
|
using Ryujinx.Input;
|
|
using Ryujinx.Input.Assigner;
|
|
using System;
|
|
using System.Threading.Tasks;
|
|
|
|
namespace Ryujinx.Ava.UI.Helpers
|
|
{
|
|
internal class ButtonKeyAssigner
|
|
{
|
|
internal class ButtonAssignedEventArgs : EventArgs
|
|
{
|
|
public ToggleButton Button { get; }
|
|
public Button? ButtonValue { get; }
|
|
|
|
public ButtonAssignedEventArgs(ToggleButton button, Button? buttonValue)
|
|
{
|
|
Button = button;
|
|
ButtonValue = buttonValue;
|
|
}
|
|
}
|
|
|
|
public ToggleButton ToggledButton { get; set; }
|
|
|
|
private bool _isWaitingForInput;
|
|
private bool _shouldUnbind;
|
|
public event EventHandler<ButtonAssignedEventArgs> ButtonAssigned;
|
|
|
|
public ButtonKeyAssigner(ToggleButton toggleButton)
|
|
{
|
|
ToggledButton = toggleButton;
|
|
}
|
|
|
|
public async void GetInputAndAssign(IButtonAssigner assigner, IKeyboard keyboard = null)
|
|
{
|
|
Dispatcher.UIThread.Post(() =>
|
|
{
|
|
ToggledButton.IsChecked = true;
|
|
});
|
|
|
|
if (_isWaitingForInput)
|
|
{
|
|
Dispatcher.UIThread.Post(() =>
|
|
{
|
|
Cancel();
|
|
});
|
|
|
|
return;
|
|
}
|
|
|
|
_isWaitingForInput = true;
|
|
|
|
assigner.Initialize();
|
|
|
|
await Task.Run(async () =>
|
|
{
|
|
while (true)
|
|
{
|
|
if (!_isWaitingForInput)
|
|
{
|
|
return;
|
|
}
|
|
|
|
await Task.Delay(10);
|
|
|
|
assigner.ReadInput();
|
|
|
|
if (assigner.IsAnyButtonPressed() || assigner.ShouldCancel() || (keyboard != null && keyboard.IsPressed(Key.Escape)))
|
|
{
|
|
break;
|
|
}
|
|
}
|
|
});
|
|
|
|
await Dispatcher.UIThread.InvokeAsync(() =>
|
|
{
|
|
Button? pressedButton = assigner.GetPressedButton();
|
|
|
|
if (_shouldUnbind)
|
|
{
|
|
pressedButton = null;
|
|
}
|
|
|
|
_shouldUnbind = false;
|
|
_isWaitingForInput = false;
|
|
|
|
ToggledButton.IsChecked = false;
|
|
|
|
ButtonAssigned?.Invoke(this, new ButtonAssignedEventArgs(ToggledButton, pressedButton));
|
|
|
|
});
|
|
}
|
|
|
|
public void Cancel(bool shouldUnbind = false)
|
|
{
|
|
_isWaitingForInput = false;
|
|
ToggledButton.IsChecked = false;
|
|
_shouldUnbind = shouldUnbind;
|
|
}
|
|
}
|
|
}
|