mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-07-13 17:21:46 +02:00
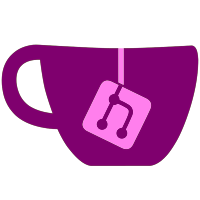
* Implement Jump Table for Native Calls NOTE: this slows down rejit considerably! Not recommended to be used without codegen optimisation or AOT. - Does not work on Linux - A32 needs an additional commit. * A32 Support (WIP) * Actually write Direct Call pointers to the table That would help. * Direct Calls: Rather than returning to the translator, attempt to keep within the native stack frame. A return to the translator can still happen, but only by exceptionally bubbling up to it. Also: - Always translate lowCq as a function. Faster interop with the direct jumps, and this will be useful in future if we want to do speculative translation. - Tail Call Detection: after the decoding stage, detect if we do a tail call, and avoid translating into it. Detected if a jump is made to an address outwith the contiguous sequence of blocks surrounding the entry point. The goal is to reduce code touched by jit and rejit. * A32 Support * Use smaller max function size for lowCq, fix exceptional returns When a return has an unexpected value and there is no code block following this one, we now return the value rather than continuing. * CompareAndSwap (buggy) * Ensure CompareAndSwap does not get optimized away. * Use CompareAndSwap to make the dynamic table thread safe. * Tail call for linux, throw on too many arguments. * Combine CompareAndSwap 128 and 32/64. They emit different IR instructions since their PreAllocator behaviour is different, but now they just have one function on EmitterContext. * Fix issues separating from optimisations. * Use a stub to find and execute missing functions. This allows us to skip doing many runtime comparisons and branches, and reduces the amount of code we need to emit significantly. For the indirect call table, this stub also does the work of moving in the highCq address to the table when one is found. * Make Jump Tables and Jit Cache dynmically resize Reserve virtual memory, commit as needed. * Move TailCallRemover to its own class. * Multithreaded Translation (based on heuristic) A poor one, at that. Need to get core count for a better one, which means a lot of OS specific garbage. * Better priority management for background threads. * Bound core limit a bit more Past a certain point the load is not paralellizable and starts stealing from the main thread. Likely due to GC, memory, heap allocation thread contention. Reduce by one core til optimisations come to improve the situation. * Fix memory management on linux. * Temporary solution to some sync problems. This will make sure threads exit correctly, most of the time. There is a potential race where setting the sync counter to 0 does nothing (counter stays at what it was before, thread could take too long to exit), but we need to find a better way to do this anyways. Synchronization frequency has been tightened as we never enter blockwise segments of code. Essentially this means, check every x functions or loop iterations, before lowcq blocks existed and were worth just as much. Ideally it should be done in a better way, since functions can be anywhere from 1 to 5000 instructions. (maybe based on host timer, or an interrupt flag from a scheduler thread) * Address feedback minus CompareAndSwap change. * Use default ReservedRegion granularity. * Merge CompareAndSwap with its V128 variant. * We already got the source, no need to do it again. * Make sure all background translation threads exit. * Fix CompareAndSwap128 Detection criteria was a bit scuffed. * Address Comments.
150 lines
5.8 KiB
C#
150 lines
5.8 KiB
C#
using ARMeilleure.Memory;
|
|
using System;
|
|
using System.Collections.Concurrent;
|
|
using System.Collections.Generic;
|
|
using System.Runtime.InteropServices;
|
|
using System.Threading;
|
|
|
|
namespace ARMeilleure.Translation
|
|
{
|
|
class JumpTable
|
|
{
|
|
public static JumpTable Instance { get; }
|
|
|
|
static JumpTable()
|
|
{
|
|
Instance = new JumpTable();
|
|
}
|
|
|
|
// The jump table is a block of (guestAddress, hostAddress) function mappings.
|
|
// Each entry corresponds to one branch in a JIT compiled function. The entries are
|
|
// reserved specifically for each call.
|
|
// The _dependants dictionary can be used to update the hostAddress for any functions that change.
|
|
|
|
public const int JumpTableStride = 16; // 8 byte guest address, 8 byte host address
|
|
|
|
private const int JumpTableSize = 1048576;
|
|
|
|
private const int JumpTableByteSize = JumpTableSize * JumpTableStride;
|
|
|
|
// The dynamic table is also a block of (guestAddress, hostAddress) function mappings.
|
|
// The main difference is that indirect calls and jumps reserve _multiple_ entries on the table.
|
|
// These start out as all 0. When an indirect call is made, it tries to find the guest address on the table.
|
|
|
|
// If we get to an empty address, the guestAddress is set to the call that we want.
|
|
|
|
// If we get to a guestAddress that matches our own (or we just claimed it), the hostAddress is read.
|
|
// If it is non-zero, we immediately branch or call the host function.
|
|
// If it is 0, NativeInterface is called to find the rejited address of the call.
|
|
// If none is found, the hostAddress entry stays at 0. Otherwise, the new address is placed in the entry.
|
|
|
|
// If the table size is exhausted and we didn't find our desired address, we fall back to requesting
|
|
// the function from the JIT.
|
|
|
|
private const int DynamicTableSize = 1048576;
|
|
|
|
public const int DynamicTableElems = 1;
|
|
|
|
public const int DynamicTableStride = DynamicTableElems * JumpTableStride;
|
|
|
|
private const int DynamicTableByteSize = DynamicTableSize * JumpTableStride * DynamicTableElems;
|
|
|
|
private int _tableEnd = 0;
|
|
private int _dynTableEnd = 0;
|
|
|
|
private ConcurrentDictionary<ulong, TranslatedFunction> _targets;
|
|
private ConcurrentDictionary<ulong, LinkedList<int>> _dependants; // TODO: Attach to TranslatedFunction or a wrapper class.
|
|
|
|
private ReservedRegion _jumpRegion;
|
|
private ReservedRegion _dynamicRegion;
|
|
public IntPtr JumpPointer => _jumpRegion.Pointer;
|
|
public IntPtr DynamicPointer => _dynamicRegion.Pointer;
|
|
|
|
public JumpTable()
|
|
{
|
|
_jumpRegion = new ReservedRegion(JumpTableByteSize);
|
|
_dynamicRegion = new ReservedRegion(DynamicTableByteSize);
|
|
|
|
_targets = new ConcurrentDictionary<ulong, TranslatedFunction>();
|
|
_dependants = new ConcurrentDictionary<ulong, LinkedList<int>>();
|
|
}
|
|
|
|
public void RegisterFunction(ulong address, TranslatedFunction func) {
|
|
address &= ~3UL;
|
|
_targets.AddOrUpdate(address, func, (key, oldFunc) => func);
|
|
long funcPtr = func.GetPointer().ToInt64();
|
|
|
|
// Update all jump table entries that target this address.
|
|
LinkedList<int> myDependants;
|
|
if (_dependants.TryGetValue(address, out myDependants))
|
|
{
|
|
lock (myDependants)
|
|
{
|
|
foreach (var entry in myDependants)
|
|
{
|
|
IntPtr addr = _jumpRegion.Pointer + entry * JumpTableStride;
|
|
Marshal.WriteInt64(addr, 8, funcPtr);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
public int ReserveDynamicEntry(bool isJump)
|
|
{
|
|
int entry = Interlocked.Increment(ref _dynTableEnd);
|
|
if (entry >= DynamicTableSize)
|
|
{
|
|
throw new OutOfMemoryException("JIT Dynamic Jump Table exhausted.");
|
|
}
|
|
|
|
_dynamicRegion.ExpandIfNeeded((ulong)((entry + 1) * DynamicTableStride));
|
|
|
|
// Initialize all host function pointers to the indirect call stub.
|
|
|
|
IntPtr addr = _dynamicRegion.Pointer + entry * DynamicTableStride;
|
|
long stubPtr = (long)DirectCallStubs.IndirectCallStub(isJump);
|
|
|
|
for (int i = 0; i < DynamicTableElems; i++)
|
|
{
|
|
Marshal.WriteInt64(addr, i * JumpTableStride + 8, stubPtr);
|
|
}
|
|
|
|
return entry;
|
|
}
|
|
|
|
public int ReserveTableEntry(long ownerAddress, long address, bool isJump)
|
|
{
|
|
int entry = Interlocked.Increment(ref _tableEnd);
|
|
if (entry >= JumpTableSize)
|
|
{
|
|
throw new OutOfMemoryException("JIT Direct Jump Table exhausted.");
|
|
}
|
|
|
|
_jumpRegion.ExpandIfNeeded((ulong)((entry + 1) * JumpTableStride));
|
|
|
|
// Is the address we have already registered? If so, put the function address in the jump table.
|
|
// If not, it will point to the direct call stub.
|
|
long value = (long)DirectCallStubs.DirectCallStub(isJump);
|
|
TranslatedFunction func;
|
|
if (_targets.TryGetValue((ulong)address, out func))
|
|
{
|
|
value = func.GetPointer().ToInt64();
|
|
}
|
|
|
|
// Make sure changes to the function at the target address update this jump table entry.
|
|
LinkedList<int> targetDependants = _dependants.GetOrAdd((ulong)address, (addr) => new LinkedList<int>());
|
|
lock (targetDependants)
|
|
{
|
|
targetDependants.AddLast(entry);
|
|
}
|
|
|
|
IntPtr addr = _jumpRegion.Pointer + entry * JumpTableStride;
|
|
|
|
Marshal.WriteInt64(addr, 0, address);
|
|
Marshal.WriteInt64(addr, 8, value);
|
|
|
|
return entry;
|
|
}
|
|
}
|
|
}
|