mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-08-11 15:20:10 +02:00
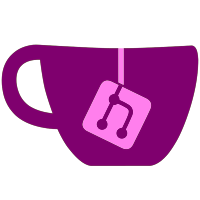
* Start of the ARMeilleure project * Refactoring around the old IRAdapter, now renamed to PreAllocator * Optimize the LowestBitSet method * Add CLZ support and fix CLS implementation * Add missing Equals and GetHashCode overrides on some structs, misc small tweaks * Implement the ByteSwap IR instruction, and some refactoring on the assembler * Implement the DivideUI IR instruction and fix 64-bits IDIV * Correct constant operand type on CSINC * Move division instructions implementation to InstEmitDiv * Fix destination type for the ConditionalSelect IR instruction * Implement UMULH and SMULH, with new IR instructions * Fix some issues with shift instructions * Fix constant types for BFM instructions * Fix up new tests using the new V128 struct * Update tests * Move DIV tests to a separate file * Add support for calls, and some instructions that depends on them * Start adding support for SIMD & FP types, along with some of the related ARM instructions * Fix some typos and the divide instruction with FP operands * Fix wrong method call on Clz_V * Implement ARM FP & SIMD move instructions, Saddlv_V, and misc. fixes * Implement SIMD logical instructions and more misc. fixes * Fix PSRAD x86 instruction encoding, TRN, UABD and UABDL implementations * Implement float conversion instruction, merge in LDj3SNuD fixes, and some other misc. fixes * Implement SIMD shift instruction and fix Dup_V * Add SCVTF and UCVTF (vector, fixed-point) variants to the opcode table * Fix check with tolerance on tester * Implement FP & SIMD comparison instructions, and some fixes * Update FCVT (Scalar) encoding on the table to support the Half-float variants * Support passing V128 structs, some cleanup on the register allocator, merge LDj3SNuD fixes * Use old memory access methods, made a start on SIMD memory insts support, some fixes * Fix float constant passed to functions, save and restore non-volatile XMM registers, other fixes * Fix arguments count with struct return values, other fixes * More instructions * Misc. fixes and integrate LDj3SNuD fixes * Update tests * Add a faster linear scan allocator, unwinding support on windows, and other changes * Update Ryujinx.HLE * Update Ryujinx.Graphics * Fix V128 return pointer passing, RCX is clobbered * Update Ryujinx.Tests * Update ITimeZoneService * Stop using GetFunctionPointer as that can't be called from native code, misc. fixes and tweaks * Use generic GetFunctionPointerForDelegate method and other tweaks * Some refactoring on the code generator, assert on invalid operations and use a separate enum for intrinsics * Remove some unused code on the assembler * Fix REX.W prefix regression on float conversion instructions, add some sort of profiler * Add hardware capability detection * Fix regression on Sha1h and revert Fcm** changes * Add SSE2-only paths on vector extract and insert, some refactoring on the pre-allocator * Fix silly mistake introduced on last commit on CpuId * Generate inline stack probes when the stack allocation is too large * Initial support for the System-V ABI * Support multiple destination operands * Fix SSE2 VectorInsert8 path, and other fixes * Change placement of XMM callee save and restore code to match other compilers * Rename Dest to Destination and Inst to Instruction * Fix a regression related to calls and the V128 type * Add an extra space on comments to match code style * Some refactoring * Fix vector insert FP32 SSE2 path * Port over the ARM32 instructions * Avoid memory protection races on JIT Cache * Another fix on VectorInsert FP32 (thanks to LDj3SNuD * Float operands don't need to use the same register when VEX is supported * Add a new register allocator, higher quality code for hot code (tier up), and other tweaks * Some nits, small improvements on the pre allocator * CpuThreadState is gone * Allow changing CPU emulators with a config entry * Add runtime identifiers on the ARMeilleure project * Allow switching between CPUs through a config entry (pt. 2) * Change win10-x64 to win-x64 on projects * Update the Ryujinx project to use ARMeilleure * Ensure that the selected register is valid on the hybrid allocator * Allow exiting on returns to 0 (should fix test regression) * Remove register assignments for most used variables on the hybrid allocator * Do not use fixed registers as spill temp * Add missing namespace and remove unneeded using * Address PR feedback * Fix types, etc * Enable AssumeStrictAbiCompliance by default * Ensure that Spill and Fill don't load or store any more than necessary
293 lines
9.1 KiB
C#
293 lines
9.1 KiB
C#
using ARMeilleure.Common;
|
|
using ARMeilleure.IntermediateRepresentation;
|
|
using ARMeilleure.State;
|
|
using System.Collections.Generic;
|
|
|
|
using static ARMeilleure.IntermediateRepresentation.OperandHelper;
|
|
|
|
namespace ARMeilleure.Translation
|
|
{
|
|
static partial class Ssa
|
|
{
|
|
private class DefMap
|
|
{
|
|
private Dictionary<Register, Operand> _map;
|
|
|
|
private BitMap _phiMasks;
|
|
|
|
public DefMap()
|
|
{
|
|
_map = new Dictionary<Register, Operand>();
|
|
|
|
_phiMasks = new BitMap(RegisterConsts.TotalCount);
|
|
}
|
|
|
|
public bool TryAddOperand(Register reg, Operand operand)
|
|
{
|
|
return _map.TryAdd(reg, operand);
|
|
}
|
|
|
|
public bool TryGetOperand(Register reg, out Operand operand)
|
|
{
|
|
return _map.TryGetValue(reg, out operand);
|
|
}
|
|
|
|
public bool AddPhi(Register reg)
|
|
{
|
|
return _phiMasks.Set(GetIdFromRegister(reg));
|
|
}
|
|
|
|
public bool HasPhi(Register reg)
|
|
{
|
|
return _phiMasks.IsSet(GetIdFromRegister(reg));
|
|
}
|
|
}
|
|
|
|
public static void Construct(ControlFlowGraph cfg)
|
|
{
|
|
DefMap[] globalDefs = new DefMap[cfg.Blocks.Count];
|
|
|
|
foreach (BasicBlock block in cfg.Blocks)
|
|
{
|
|
globalDefs[block.Index] = new DefMap();
|
|
}
|
|
|
|
Queue<BasicBlock> dfPhiBlocks = new Queue<BasicBlock>();
|
|
|
|
// First pass, get all defs and locals uses.
|
|
foreach (BasicBlock block in cfg.Blocks)
|
|
{
|
|
Operand[] localDefs = new Operand[RegisterConsts.TotalCount];
|
|
|
|
LinkedListNode<Node> node = block.Operations.First;
|
|
|
|
Operand RenameLocal(Operand operand)
|
|
{
|
|
if (operand != null && operand.Kind == OperandKind.Register)
|
|
{
|
|
Operand local = localDefs[GetIdFromRegister(operand.GetRegister())];
|
|
|
|
operand = local ?? operand;
|
|
}
|
|
|
|
return operand;
|
|
}
|
|
|
|
while (node != null)
|
|
{
|
|
if (node.Value is Operation operation)
|
|
{
|
|
for (int index = 0; index < operation.SourcesCount; index++)
|
|
{
|
|
operation.SetSource(index, RenameLocal(operation.GetSource(index)));
|
|
}
|
|
|
|
Operand dest = operation.Destination;
|
|
|
|
if (dest != null && dest.Kind == OperandKind.Register)
|
|
{
|
|
Operand local = Local(dest.Type);
|
|
|
|
localDefs[GetIdFromRegister(dest.GetRegister())] = local;
|
|
|
|
operation.Destination = local;
|
|
}
|
|
}
|
|
|
|
node = node.Next;
|
|
}
|
|
|
|
for (int index = 0; index < RegisterConsts.TotalCount; index++)
|
|
{
|
|
Operand local = localDefs[index];
|
|
|
|
if (local == null)
|
|
{
|
|
continue;
|
|
}
|
|
|
|
Register reg = GetRegisterFromId(index);
|
|
|
|
globalDefs[block.Index].TryAddOperand(reg, local);
|
|
|
|
dfPhiBlocks.Enqueue(block);
|
|
|
|
while (dfPhiBlocks.TryDequeue(out BasicBlock dfPhiBlock))
|
|
{
|
|
foreach (BasicBlock domFrontier in dfPhiBlock.DominanceFrontiers)
|
|
{
|
|
if (globalDefs[domFrontier.Index].AddPhi(reg))
|
|
{
|
|
dfPhiBlocks.Enqueue(domFrontier);
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
// Second pass, rename variables with definitions on different blocks.
|
|
foreach (BasicBlock block in cfg.Blocks)
|
|
{
|
|
Operand[] localDefs = new Operand[RegisterConsts.TotalCount];
|
|
|
|
LinkedListNode<Node> node = block.Operations.First;
|
|
|
|
Operand RenameGlobal(Operand operand)
|
|
{
|
|
if (operand != null && operand.Kind == OperandKind.Register)
|
|
{
|
|
int key = GetIdFromRegister(operand.GetRegister());
|
|
|
|
Operand local = localDefs[key];
|
|
|
|
if (local == null)
|
|
{
|
|
local = FindDef(globalDefs, block, operand);
|
|
|
|
localDefs[key] = local;
|
|
}
|
|
|
|
operand = local;
|
|
}
|
|
|
|
return operand;
|
|
}
|
|
|
|
while (node != null)
|
|
{
|
|
if (node.Value is Operation operation)
|
|
{
|
|
for (int index = 0; index < operation.SourcesCount; index++)
|
|
{
|
|
operation.SetSource(index, RenameGlobal(operation.GetSource(index)));
|
|
}
|
|
}
|
|
|
|
node = node.Next;
|
|
}
|
|
}
|
|
}
|
|
|
|
private static Operand FindDef(DefMap[] globalDefs, BasicBlock current, Operand operand)
|
|
{
|
|
if (globalDefs[current.Index].HasPhi(operand.GetRegister()))
|
|
{
|
|
return InsertPhi(globalDefs, current, operand);
|
|
}
|
|
|
|
if (current != current.ImmediateDominator)
|
|
{
|
|
return FindDefOnPred(globalDefs, current.ImmediateDominator, operand);
|
|
}
|
|
|
|
return Undef();
|
|
}
|
|
|
|
private static Operand FindDefOnPred(DefMap[] globalDefs, BasicBlock current, Operand operand)
|
|
{
|
|
BasicBlock previous;
|
|
|
|
do
|
|
{
|
|
DefMap defMap = globalDefs[current.Index];
|
|
|
|
Register reg = operand.GetRegister();
|
|
|
|
if (defMap.TryGetOperand(reg, out Operand lastDef))
|
|
{
|
|
return lastDef;
|
|
}
|
|
|
|
if (defMap.HasPhi(reg))
|
|
{
|
|
return InsertPhi(globalDefs, current, operand);
|
|
}
|
|
|
|
previous = current;
|
|
current = current.ImmediateDominator;
|
|
}
|
|
while (previous != current);
|
|
|
|
return Undef();
|
|
}
|
|
|
|
private static Operand InsertPhi(DefMap[] globalDefs, BasicBlock block, Operand operand)
|
|
{
|
|
// This block has a Phi that has not been materialized yet, but that
|
|
// would define a new version of the variable we're looking for. We need
|
|
// to materialize the Phi, add all the block/operand pairs into the Phi, and
|
|
// then use the definition from that Phi.
|
|
Operand local = Local(operand.Type);
|
|
|
|
PhiNode phi = new PhiNode(local, block.Predecessors.Count);
|
|
|
|
AddPhi(block, phi);
|
|
|
|
globalDefs[block.Index].TryAddOperand(operand.GetRegister(), local);
|
|
|
|
for (int index = 0; index < block.Predecessors.Count; index++)
|
|
{
|
|
BasicBlock predecessor = block.Predecessors[index];
|
|
|
|
phi.SetBlock(index, predecessor);
|
|
phi.SetSource(index, FindDefOnPred(globalDefs, predecessor, operand));
|
|
}
|
|
|
|
return local;
|
|
}
|
|
|
|
private static void AddPhi(BasicBlock block, PhiNode phi)
|
|
{
|
|
LinkedListNode<Node> node = block.Operations.First;
|
|
|
|
if (node != null)
|
|
{
|
|
while (node.Next?.Value is PhiNode)
|
|
{
|
|
node = node.Next;
|
|
}
|
|
}
|
|
|
|
if (node?.Value is PhiNode)
|
|
{
|
|
block.Operations.AddAfter(node, phi);
|
|
}
|
|
else
|
|
{
|
|
block.Operations.AddFirst(phi);
|
|
}
|
|
}
|
|
|
|
private static int GetIdFromRegister(Register reg)
|
|
{
|
|
if (reg.Type == RegisterType.Integer)
|
|
{
|
|
return reg.Index;
|
|
}
|
|
else if (reg.Type == RegisterType.Vector)
|
|
{
|
|
return RegisterConsts.IntRegsCount + reg.Index;
|
|
}
|
|
else /* if (reg.Type == RegisterType.Flag) */
|
|
{
|
|
return RegisterConsts.IntAndVecRegsCount + reg.Index;
|
|
}
|
|
}
|
|
|
|
private static Register GetRegisterFromId(int id)
|
|
{
|
|
if (id < RegisterConsts.IntRegsCount)
|
|
{
|
|
return new Register(id, RegisterType.Integer);
|
|
}
|
|
else if (id < RegisterConsts.IntAndVecRegsCount)
|
|
{
|
|
return new Register(id - RegisterConsts.IntRegsCount, RegisterType.Vector);
|
|
}
|
|
else /* if (id < RegisterConsts.TotalCount) */
|
|
{
|
|
return new Register(id - RegisterConsts.IntAndVecRegsCount, RegisterType.Flag);
|
|
}
|
|
}
|
|
}
|
|
} |