mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-09-20 18:19:52 +02:00
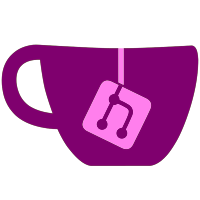
* Fix inconsistencies with UserId The account user id isn't an UUID. This PR adds a new UserId type with the correct value ordering to avoid mismatch with LibHac's Uid. This also fix an hardcoded value of the UserId. As the userid has been invalid for quite some time (and to avoid forcing users to their recreate saves), the userid has been changed to "00000000000000010000000000000000". Also implement a stub for IApplicationFunctions::GetSaveDataSize. (see the sources for the reason) Fix #626 * Address jd's & Ac_k's comments
66 lines
1.8 KiB
C#
66 lines
1.8 KiB
C#
using System.Collections.Concurrent;
|
|
using System.Collections.Generic;
|
|
using System.Linq;
|
|
|
|
namespace Ryujinx.HLE.HOS.Services.Account.Acc
|
|
{
|
|
public class AccountUtils
|
|
{
|
|
private ConcurrentDictionary<string, UserProfile> _profiles;
|
|
|
|
internal UserProfile LastOpenedUser { get; private set; }
|
|
|
|
public AccountUtils()
|
|
{
|
|
_profiles = new ConcurrentDictionary<string, UserProfile>();
|
|
}
|
|
|
|
public void AddUser(UserId userId, string name)
|
|
{
|
|
UserProfile profile = new UserProfile(userId, name);
|
|
|
|
_profiles.AddOrUpdate(userId.ToString(), profile, (key, old) => profile);
|
|
}
|
|
|
|
public void OpenUser(UserId userId)
|
|
{
|
|
if (_profiles.TryGetValue(userId.ToString(), out UserProfile profile))
|
|
{
|
|
(LastOpenedUser = profile).AccountState = AccountState.Open;
|
|
}
|
|
}
|
|
|
|
public void CloseUser(UserId userId)
|
|
{
|
|
if (_profiles.TryGetValue(userId.ToString(), out UserProfile profile))
|
|
{
|
|
profile.AccountState = AccountState.Closed;
|
|
}
|
|
}
|
|
|
|
public int GetUserCount()
|
|
{
|
|
return _profiles.Count;
|
|
}
|
|
|
|
internal bool TryGetUser(UserId userId, out UserProfile profile)
|
|
{
|
|
return _profiles.TryGetValue(userId.ToString(), out profile);
|
|
}
|
|
|
|
internal IEnumerable<UserProfile> GetAllUsers()
|
|
{
|
|
return _profiles.Values;
|
|
}
|
|
|
|
internal IEnumerable<UserProfile> GetOpenedUsers()
|
|
{
|
|
return _profiles.Values.Where(x => x.AccountState == AccountState.Open);
|
|
}
|
|
|
|
internal UserProfile GetFirst()
|
|
{
|
|
return _profiles.First().Value;
|
|
}
|
|
}
|
|
} |