mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
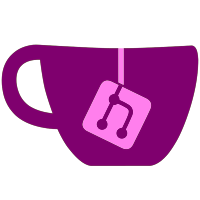
* Migrate audren to new IPC * Migrate audout * Migrate audin * Migrate hwopus * Bye bye old audio service * Switch volume control to IHardwareDeviceDriver * Somewhat unrelated changes * Remove Concentus reference from HLE * Implement OpenAudioRendererForManualExecution * Remove SetVolume/GetVolume methods that are not necessary * Remove SetVolume/GetVolume methods that are not necessary (2) * Fix incorrect volume update * PR feedback * PR feedback * Stub audrec * Init outParameter * Make FinalOutputRecorderParameter/Internal readonly * Make FinalOutputRecorder IDisposable * Fix HardwareOpusDecoderManager parameter buffers * Opus work buffer size and error handling improvements * Add AudioInProtocolName enum * Fix potential divisions by zero
77 lines
2.0 KiB
C#
77 lines
2.0 KiB
C#
using Ryujinx.Audio.Common;
|
|
using System;
|
|
using System.Runtime.InteropServices;
|
|
|
|
namespace Ryujinx.Audio.Integration
|
|
{
|
|
public class HardwareDeviceImpl : IHardwareDevice
|
|
{
|
|
private readonly IHardwareDeviceSession _session;
|
|
private readonly uint _channelCount;
|
|
private readonly uint _sampleRate;
|
|
private uint _currentBufferTag;
|
|
|
|
private readonly byte[] _buffer;
|
|
|
|
public HardwareDeviceImpl(IHardwareDeviceDriver deviceDriver, uint channelCount, uint sampleRate)
|
|
{
|
|
_session = deviceDriver.OpenDeviceSession(IHardwareDeviceDriver.Direction.Output, null, SampleFormat.PcmInt16, sampleRate, channelCount);
|
|
_channelCount = channelCount;
|
|
_sampleRate = sampleRate;
|
|
_currentBufferTag = 0;
|
|
|
|
_buffer = new byte[Constants.TargetSampleCount * channelCount * sizeof(ushort)];
|
|
|
|
_session.Start();
|
|
}
|
|
|
|
public void AppendBuffer(ReadOnlySpan<short> data, uint channelCount)
|
|
{
|
|
data.CopyTo(MemoryMarshal.Cast<byte, short>(_buffer));
|
|
|
|
_session.QueueBuffer(new AudioBuffer
|
|
{
|
|
DataPointer = _currentBufferTag++,
|
|
Data = _buffer,
|
|
DataSize = (ulong)_buffer.Length,
|
|
});
|
|
|
|
_currentBufferTag %= 4;
|
|
}
|
|
|
|
public void SetVolume(float volume)
|
|
{
|
|
_session.SetVolume(volume);
|
|
}
|
|
|
|
public float GetVolume()
|
|
{
|
|
return _session.GetVolume();
|
|
}
|
|
|
|
public uint GetChannelCount()
|
|
{
|
|
return _channelCount;
|
|
}
|
|
|
|
public uint GetSampleRate()
|
|
{
|
|
return _sampleRate;
|
|
}
|
|
|
|
public void Dispose()
|
|
{
|
|
GC.SuppressFinalize(this);
|
|
Dispose(true);
|
|
}
|
|
|
|
protected virtual void Dispose(bool disposing)
|
|
{
|
|
if (disposing)
|
|
{
|
|
_session.Dispose();
|
|
}
|
|
}
|
|
}
|
|
}
|