mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-09-20 18:19:52 +02:00
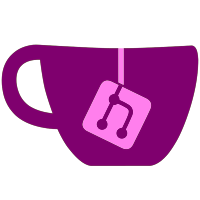
* Fix inconsistencies with UserId The account user id isn't an UUID. This PR adds a new UserId type with the correct value ordering to avoid mismatch with LibHac's Uid. This also fix an hardcoded value of the UserId. As the userid has been invalid for quite some time (and to avoid forcing users to their recreate saves), the userid has been changed to "00000000000000010000000000000000". Also implement a stub for IApplicationFunctions::GetSaveDataSize. (see the sources for the reason) Fix #626 * Address jd's & Ac_k's comments
116 lines
3.0 KiB
C#
116 lines
3.0 KiB
C#
using Ryujinx.HLE.HOS.Services.Account.Acc;
|
|
using System;
|
|
|
|
namespace Ryujinx.HLE.HOS.SystemState
|
|
{
|
|
public class SystemStateMgr
|
|
{
|
|
internal static string[] LanguageCodes = new string[]
|
|
{
|
|
"ja",
|
|
"en-US",
|
|
"fr",
|
|
"de",
|
|
"it",
|
|
"es",
|
|
"zh-CN",
|
|
"ko",
|
|
"nl",
|
|
"pt",
|
|
"ru",
|
|
"zh-TW",
|
|
"en-GB",
|
|
"fr-CA",
|
|
"es-419",
|
|
"zh-Hans",
|
|
"zh-Hant"
|
|
};
|
|
|
|
internal static string[] AudioOutputs = new string[]
|
|
{
|
|
"AudioTvOutput",
|
|
"AudioStereoJackOutput",
|
|
"AudioBuiltInSpeakerOutput"
|
|
};
|
|
|
|
internal long DesiredLanguageCode { get; private set; }
|
|
|
|
public TitleLanguage DesiredTitleLanguage { get; private set; }
|
|
|
|
internal string ActiveAudioOutput { get; private set; }
|
|
|
|
public bool DockedMode { get; set; }
|
|
|
|
public ColorSet ThemeColor { get; set; }
|
|
|
|
public bool InstallContents { get; set; }
|
|
|
|
public AccountUtils Account { get; private set; }
|
|
|
|
public SystemStateMgr()
|
|
{
|
|
SetAudioOutputAsBuiltInSpeaker();
|
|
|
|
Account = new AccountUtils();
|
|
|
|
UserId defaultUid = new UserId("00000000000000010000000000000000");
|
|
|
|
Account.AddUser(defaultUid, "Player");
|
|
Account.OpenUser(defaultUid);
|
|
}
|
|
|
|
public void SetLanguage(SystemLanguage language)
|
|
{
|
|
DesiredLanguageCode = GetLanguageCode((int)language);
|
|
|
|
switch (language)
|
|
{
|
|
case SystemLanguage.Taiwanese:
|
|
case SystemLanguage.TraditionalChinese:
|
|
DesiredTitleLanguage = TitleLanguage.Taiwanese;
|
|
break;
|
|
case SystemLanguage.Chinese:
|
|
case SystemLanguage.SimplifiedChinese:
|
|
DesiredTitleLanguage = TitleLanguage.Chinese;
|
|
break;
|
|
default:
|
|
DesiredTitleLanguage = Enum.Parse<TitleLanguage>(Enum.GetName(typeof(SystemLanguage), language));
|
|
break;
|
|
}
|
|
}
|
|
|
|
public void SetAudioOutputAsTv()
|
|
{
|
|
ActiveAudioOutput = AudioOutputs[0];
|
|
}
|
|
|
|
public void SetAudioOutputAsStereoJack()
|
|
{
|
|
ActiveAudioOutput = AudioOutputs[1];
|
|
}
|
|
|
|
public void SetAudioOutputAsBuiltInSpeaker()
|
|
{
|
|
ActiveAudioOutput = AudioOutputs[2];
|
|
}
|
|
|
|
internal static long GetLanguageCode(int index)
|
|
{
|
|
if ((uint)index >= LanguageCodes.Length)
|
|
{
|
|
throw new ArgumentOutOfRangeException(nameof(index));
|
|
}
|
|
|
|
long code = 0;
|
|
int shift = 0;
|
|
|
|
foreach (char chr in LanguageCodes[index])
|
|
{
|
|
code |= (long)(byte)chr << shift++ * 8;
|
|
}
|
|
|
|
return code;
|
|
}
|
|
}
|
|
}
|