mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
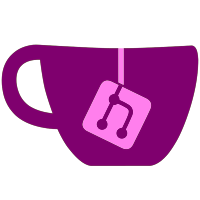
* avalonia part 1 * remove vulkan ui backend * move ui common files to ui common project * get name for oading screen from device * rebase. * review 1 * review 1.1 * review * cleanup * addressed review * use cancellation token * review * review * rebased * cancel library loading when closing window * remove star image, use fonticon instead * delete render control frame buffer when game ends. change position of fav star * addressed @Thog review * ensure the right ui is downloaded in updates * fix crash when showing not supported dialog during controller request * add prefix to artifact names * Auto-format Avalonia project * Fix input * Fix build, simplify app disposal * remove nv stutter thread * addressed review * add missing change * maintain window size if new size is zero length * add game, handheld, docked to local * reverse scale main window * Update de_DE.json * Update de_DE.json * Update de_DE.json * Update italian json * Update it_IT.json * let render timer poll with no wait * remove unused code * more unused code * enabled tiered compilation and trimming * check if window event is not closed before signaling * fix atmospher case * locale fix * locale fix * remove explicit tiered compilation declarations * Remove ) it_IT.json * Remove ) de_DE.json * Update it_IT.json * Update pt_BR locale with latest strings * Remove ')' * add more strings to locale * update locale * remove extra slash * remove extra slash * set firmware version to 0 if key's not found * fix * revert timer changes * lock on object instead * Update it_IT.json * remove unused method * add load screen text to locale * drop swap event * Update de_DE.json * Update de_DE.json * do null check when stopping emulator * Update de_DE.json * Create tr_TR.json * Add tr_TR * Add tr_TR + Turkish * Update it_IT.json * Update Ryujinx.Ava/Input/AvaloniaMappingHelper.cs Co-authored-by: Ac_K <Acoustik666@gmail.com> * Apply suggestions from code review Co-authored-by: Ac_K <Acoustik666@gmail.com> * Apply suggestions from code review Co-authored-by: Ac_K <Acoustik666@gmail.com> * addressed review * Update Ryujinx.Ava/Ui/Backend/OpenGl/OpenGlRenderTarget.cs Co-authored-by: gdkchan <gab.dark.100@gmail.com> * use avalonia's inbuilt renderer on linux * removed whitespace * workaround for queue render crash with vsync off * drop custom backend * format files * fix not closing issue * remove warnings * rebase * update avalonia library * Reposition the Text and Button on About Page * Assign build version * Remove appveyor text Co-authored-by: gdk <gab.dark.100@gmail.com> Co-authored-by: Niwu34 <67392333+Niwu34@users.noreply.github.com> Co-authored-by: Antonio Brugnolo <36473846+AntoSkate@users.noreply.github.com> Co-authored-by: aegiff <99728970+aegiff@users.noreply.github.com> Co-authored-by: Ac_K <Acoustik666@gmail.com> Co-authored-by: MostlyWhat <78652091+MostlyWhat@users.noreply.github.com>
274 lines
7.9 KiB
C#
274 lines
7.9 KiB
C#
using Avalonia;
|
|
using Avalonia.Controls;
|
|
using Avalonia.Data;
|
|
using Avalonia.Media;
|
|
using Avalonia.OpenGL;
|
|
using Avalonia.Platform;
|
|
using Avalonia.Rendering.SceneGraph;
|
|
using Avalonia.Skia;
|
|
using Avalonia.Threading;
|
|
using OpenTK.Graphics.OpenGL;
|
|
using Ryujinx.Common.Configuration;
|
|
using SkiaSharp;
|
|
using SPB.Graphics;
|
|
using SPB.Graphics.OpenGL;
|
|
using SPB.Platform;
|
|
using SPB.Windowing;
|
|
using System;
|
|
|
|
namespace Ryujinx.Ava.Ui.Controls
|
|
{
|
|
public class RendererControl : Control
|
|
{
|
|
private int _image;
|
|
|
|
static RendererControl()
|
|
{
|
|
AffectsRender<RendererControl>(ImageProperty);
|
|
}
|
|
|
|
public readonly static StyledProperty<int> ImageProperty =
|
|
AvaloniaProperty.Register<RendererControl, int>(nameof(Image), 0, inherits: true, defaultBindingMode: BindingMode.TwoWay);
|
|
|
|
protected int Image
|
|
{
|
|
get => _image;
|
|
set => SetAndRaise(ImageProperty, ref _image, value);
|
|
}
|
|
|
|
public event EventHandler<EventArgs> GlInitialized;
|
|
public event EventHandler<Size> SizeChanged;
|
|
|
|
protected Size RenderSize { get; private set; }
|
|
public bool IsStarted { get; private set; }
|
|
|
|
public int Major { get; }
|
|
public int Minor { get; }
|
|
public GraphicsDebugLevel DebugLevel { get; }
|
|
public OpenGLContextBase GameContext { get; set; }
|
|
|
|
public static OpenGLContextBase PrimaryContext => AvaloniaLocator.Current.GetService<IPlatformOpenGlInterface>().PrimaryContext.AsOpenGLContextBase();
|
|
|
|
private SwappableNativeWindowBase _gameBackgroundWindow;
|
|
|
|
private bool _isInitialized;
|
|
|
|
private IntPtr _fence;
|
|
|
|
private GlDrawOperation _glDrawOperation;
|
|
|
|
public RendererControl(int major, int minor, GraphicsDebugLevel graphicsDebugLevel)
|
|
{
|
|
Major = major;
|
|
Minor = minor;
|
|
DebugLevel = graphicsDebugLevel;
|
|
IObservable<Rect> resizeObservable = this.GetObservable(BoundsProperty);
|
|
|
|
resizeObservable.Subscribe(Resized);
|
|
|
|
Focusable = true;
|
|
}
|
|
|
|
private void Resized(Rect rect)
|
|
{
|
|
SizeChanged?.Invoke(this, rect.Size);
|
|
|
|
RenderSize = rect.Size * Program.WindowScaleFactor;
|
|
|
|
_glDrawOperation?.Dispose();
|
|
_glDrawOperation = new GlDrawOperation(this);
|
|
}
|
|
|
|
public override void Render(DrawingContext context)
|
|
{
|
|
if (!_isInitialized)
|
|
{
|
|
CreateWindow();
|
|
|
|
OnGlInitialized();
|
|
_isInitialized = true;
|
|
}
|
|
|
|
if (GameContext == null || !IsStarted || Image == 0)
|
|
{
|
|
return;
|
|
}
|
|
|
|
if (_glDrawOperation != null)
|
|
{
|
|
context.Custom(_glDrawOperation);
|
|
}
|
|
|
|
base.Render(context);
|
|
}
|
|
|
|
protected void OnGlInitialized()
|
|
{
|
|
GlInitialized?.Invoke(this, EventArgs.Empty);
|
|
}
|
|
|
|
public void QueueRender()
|
|
{
|
|
Program.RenderTimer.TickNow();
|
|
}
|
|
|
|
internal void Present(object image)
|
|
{
|
|
Dispatcher.UIThread.InvokeAsync(() =>
|
|
{
|
|
Image = (int)image;
|
|
}).Wait();
|
|
|
|
if (_fence != IntPtr.Zero)
|
|
{
|
|
GL.DeleteSync(_fence);
|
|
}
|
|
|
|
_fence = GL.FenceSync(SyncCondition.SyncGpuCommandsComplete, WaitSyncFlags.None);
|
|
|
|
QueueRender();
|
|
|
|
_gameBackgroundWindow.SwapBuffers();
|
|
}
|
|
|
|
internal void Start()
|
|
{
|
|
IsStarted = true;
|
|
QueueRender();
|
|
}
|
|
|
|
internal void Stop()
|
|
{
|
|
IsStarted = false;
|
|
}
|
|
|
|
public void DestroyBackgroundContext()
|
|
{
|
|
_image = 0;
|
|
|
|
if (_fence != IntPtr.Zero)
|
|
{
|
|
_glDrawOperation.Dispose();
|
|
GL.DeleteSync(_fence);
|
|
}
|
|
|
|
GlDrawOperation.DeleteFramebuffer();
|
|
|
|
GameContext?.Dispose();
|
|
|
|
_gameBackgroundWindow?.Dispose();
|
|
}
|
|
|
|
internal void MakeCurrent()
|
|
{
|
|
GameContext.MakeCurrent(_gameBackgroundWindow);
|
|
}
|
|
|
|
internal void MakeCurrent(SwappableNativeWindowBase window)
|
|
{
|
|
GameContext.MakeCurrent(window);
|
|
}
|
|
|
|
protected void CreateWindow()
|
|
{
|
|
var flags = OpenGLContextFlags.Compat;
|
|
if (DebugLevel != GraphicsDebugLevel.None)
|
|
{
|
|
flags |= OpenGLContextFlags.Debug;
|
|
}
|
|
_gameBackgroundWindow = PlatformHelper.CreateOpenGLWindow(FramebufferFormat.Default, 0, 0, 100, 100);
|
|
_gameBackgroundWindow.Hide();
|
|
|
|
GameContext = PlatformHelper.CreateOpenGLContext(FramebufferFormat.Default, Major, Minor, flags, shareContext: PrimaryContext);
|
|
GameContext.Initialize(_gameBackgroundWindow);
|
|
}
|
|
|
|
private class GlDrawOperation : ICustomDrawOperation
|
|
{
|
|
private static int _framebuffer;
|
|
|
|
public Rect Bounds { get; }
|
|
|
|
private readonly RendererControl _control;
|
|
|
|
public GlDrawOperation(RendererControl control)
|
|
{
|
|
_control = control;
|
|
Bounds = _control.Bounds;
|
|
}
|
|
|
|
public void Dispose() { }
|
|
|
|
public static void DeleteFramebuffer()
|
|
{
|
|
if (_framebuffer == 0)
|
|
{
|
|
GL.DeleteFramebuffer(_framebuffer);
|
|
}
|
|
|
|
_framebuffer = 0;
|
|
}
|
|
|
|
public bool Equals(ICustomDrawOperation other)
|
|
{
|
|
return other is GlDrawOperation operation && Equals(this, operation) && operation.Bounds == Bounds;
|
|
}
|
|
|
|
public bool HitTest(Point p)
|
|
{
|
|
return Bounds.Contains(p);
|
|
}
|
|
|
|
private void CreateRenderTarget()
|
|
{
|
|
_framebuffer = GL.GenFramebuffer();
|
|
}
|
|
|
|
public void Render(IDrawingContextImpl context)
|
|
{
|
|
if (_control.Image == 0)
|
|
{
|
|
return;
|
|
}
|
|
|
|
if (_framebuffer == 0)
|
|
{
|
|
CreateRenderTarget();
|
|
}
|
|
|
|
int currentFramebuffer = GL.GetInteger(GetPName.FramebufferBinding);
|
|
|
|
var image = _control.Image;
|
|
var fence = _control._fence;
|
|
|
|
GL.BindFramebuffer(FramebufferTarget.Framebuffer, _framebuffer);
|
|
GL.FramebufferTexture2D(FramebufferTarget.Framebuffer, FramebufferAttachment.ColorAttachment0, TextureTarget.Texture2D, image, 0);
|
|
GL.BindFramebuffer(FramebufferTarget.Framebuffer, currentFramebuffer);
|
|
|
|
if (context is not ISkiaDrawingContextImpl skiaDrawingContextImpl)
|
|
{
|
|
return;
|
|
}
|
|
|
|
var imageInfo = new SKImageInfo((int)_control.RenderSize.Width, (int)_control.RenderSize.Height, SKColorType.Rgba8888);
|
|
var glInfo = new GRGlFramebufferInfo((uint)_framebuffer, SKColorType.Rgba8888.ToGlSizedFormat());
|
|
|
|
GL.WaitSync(fence, WaitSyncFlags.None, ulong.MaxValue);
|
|
|
|
using var backendTexture = new GRBackendRenderTarget(imageInfo.Width, imageInfo.Height, 1, 0, glInfo);
|
|
using var surface = SKSurface.Create(skiaDrawingContextImpl.GrContext, backendTexture, GRSurfaceOrigin.BottomLeft, SKColorType.Rgba8888);
|
|
|
|
if (surface == null)
|
|
{
|
|
return;
|
|
}
|
|
|
|
var rect = new Rect(new Point(), _control.RenderSize);
|
|
|
|
using var snapshot = surface.Snapshot();
|
|
skiaDrawingContextImpl.SkCanvas.DrawImage(snapshot, rect.ToSKRect(), _control.Bounds.ToSKRect(), new SKPaint());
|
|
}
|
|
}
|
|
}
|
|
}
|