mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-06-02 21:28:46 +02:00
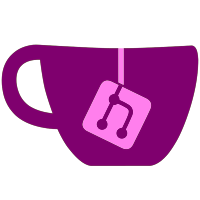
* avalonia part 1 * remove vulkan ui backend * move ui common files to ui common project * get name for oading screen from device * rebase. * review 1 * review 1.1 * review * cleanup * addressed review * use cancellation token * review * review * rebased * cancel library loading when closing window * remove star image, use fonticon instead * delete render control frame buffer when game ends. change position of fav star * addressed @Thog review * ensure the right ui is downloaded in updates * fix crash when showing not supported dialog during controller request * add prefix to artifact names * Auto-format Avalonia project * Fix input * Fix build, simplify app disposal * remove nv stutter thread * addressed review * add missing change * maintain window size if new size is zero length * add game, handheld, docked to local * reverse scale main window * Update de_DE.json * Update de_DE.json * Update de_DE.json * Update italian json * Update it_IT.json * let render timer poll with no wait * remove unused code * more unused code * enabled tiered compilation and trimming * check if window event is not closed before signaling * fix atmospher case * locale fix * locale fix * remove explicit tiered compilation declarations * Remove ) it_IT.json * Remove ) de_DE.json * Update it_IT.json * Update pt_BR locale with latest strings * Remove ')' * add more strings to locale * update locale * remove extra slash * remove extra slash * set firmware version to 0 if key's not found * fix * revert timer changes * lock on object instead * Update it_IT.json * remove unused method * add load screen text to locale * drop swap event * Update de_DE.json * Update de_DE.json * do null check when stopping emulator * Update de_DE.json * Create tr_TR.json * Add tr_TR * Add tr_TR + Turkish * Update it_IT.json * Update Ryujinx.Ava/Input/AvaloniaMappingHelper.cs Co-authored-by: Ac_K <Acoustik666@gmail.com> * Apply suggestions from code review Co-authored-by: Ac_K <Acoustik666@gmail.com> * Apply suggestions from code review Co-authored-by: Ac_K <Acoustik666@gmail.com> * addressed review * Update Ryujinx.Ava/Ui/Backend/OpenGl/OpenGlRenderTarget.cs Co-authored-by: gdkchan <gab.dark.100@gmail.com> * use avalonia's inbuilt renderer on linux * removed whitespace * workaround for queue render crash with vsync off * drop custom backend * format files * fix not closing issue * remove warnings * rebase * update avalonia library * Reposition the Text and Button on About Page * Assign build version * Remove appveyor text Co-authored-by: gdk <gab.dark.100@gmail.com> Co-authored-by: Niwu34 <67392333+Niwu34@users.noreply.github.com> Co-authored-by: Antonio Brugnolo <36473846+AntoSkate@users.noreply.github.com> Co-authored-by: aegiff <99728970+aegiff@users.noreply.github.com> Co-authored-by: Ac_K <Acoustik666@gmail.com> Co-authored-by: MostlyWhat <78652091+MostlyWhat@users.noreply.github.com>
188 lines
6.8 KiB
C#
188 lines
6.8 KiB
C#
using OpenTK;
|
|
using OpenTK.Graphics.OpenGL;
|
|
using Ryujinx.Common.Configuration;
|
|
using Ryujinx.Common.Logging;
|
|
using Ryujinx.Graphics.OpenGL;
|
|
using Ryujinx.Input.HLE;
|
|
using System;
|
|
|
|
using static SDL2.SDL;
|
|
|
|
namespace Ryujinx.Headless.SDL2.OpenGL
|
|
{
|
|
class OpenGLWindow : WindowBase
|
|
{
|
|
private static void SetupOpenGLAttributes(bool sharedContext, GraphicsDebugLevel debugLevel)
|
|
{
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_CONTEXT_MAJOR_VERSION, 3);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_CONTEXT_MINOR_VERSION, 3);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_CONTEXT_PROFILE_MASK, SDL_GLprofile.SDL_GL_CONTEXT_PROFILE_COMPATIBILITY);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_CONTEXT_FLAGS, debugLevel != GraphicsDebugLevel.None ? (int)SDL_GLcontext.SDL_GL_CONTEXT_DEBUG_FLAG : 0);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_SHARE_WITH_CURRENT_CONTEXT, sharedContext ? 1 : 0);
|
|
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_ACCELERATED_VISUAL, 1);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_RED_SIZE, 8);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_GREEN_SIZE, 8);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_BLUE_SIZE, 8);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_ALPHA_SIZE, 8);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_DEPTH_SIZE, 16);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_STENCIL_SIZE, 0);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_DOUBLEBUFFER, 1);
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_STEREO, 0);
|
|
}
|
|
|
|
private class OpenToolkitBindingsContext : IBindingsContext
|
|
{
|
|
public IntPtr GetProcAddress(string procName)
|
|
{
|
|
return SDL_GL_GetProcAddress(procName);
|
|
}
|
|
}
|
|
|
|
private class SDL2OpenGLContext : IOpenGLContext
|
|
{
|
|
private IntPtr _context;
|
|
private IntPtr _window;
|
|
private bool _shouldDisposeWindow;
|
|
|
|
public SDL2OpenGLContext(IntPtr context, IntPtr window, bool shouldDisposeWindow = true)
|
|
{
|
|
_context = context;
|
|
_window = window;
|
|
_shouldDisposeWindow = shouldDisposeWindow;
|
|
}
|
|
|
|
public static SDL2OpenGLContext CreateBackgroundContext(SDL2OpenGLContext sharedContext)
|
|
{
|
|
sharedContext.MakeCurrent();
|
|
|
|
// Ensure we share our contexts.
|
|
SetupOpenGLAttributes(true, GraphicsDebugLevel.None);
|
|
IntPtr windowHandle = SDL_CreateWindow("Ryujinx background context window", 0, 0, 1, 1, SDL_WindowFlags.SDL_WINDOW_OPENGL | SDL_WindowFlags.SDL_WINDOW_HIDDEN);
|
|
IntPtr context = SDL_GL_CreateContext(windowHandle);
|
|
|
|
GL.LoadBindings(new OpenToolkitBindingsContext());
|
|
|
|
SDL_GL_SetAttribute(SDL_GLattr.SDL_GL_SHARE_WITH_CURRENT_CONTEXT, 0);
|
|
|
|
SDL_GL_MakeCurrent(windowHandle, IntPtr.Zero);
|
|
|
|
return new SDL2OpenGLContext(context, windowHandle);
|
|
}
|
|
|
|
public void MakeCurrent()
|
|
{
|
|
if (SDL_GL_GetCurrentContext() == _context || SDL_GL_GetCurrentWindow() == _window)
|
|
{
|
|
return;
|
|
}
|
|
|
|
int res = SDL_GL_MakeCurrent(_window, _context);
|
|
|
|
if (res != 0)
|
|
{
|
|
string errorMessage = $"SDL_GL_CreateContext failed with error \"{SDL_GetError()}\"";
|
|
|
|
Logger.Error?.Print(LogClass.Application, errorMessage);
|
|
|
|
throw new Exception(errorMessage);
|
|
}
|
|
}
|
|
|
|
public void Dispose()
|
|
{
|
|
SDL_GL_DeleteContext(_context);
|
|
|
|
if (_shouldDisposeWindow)
|
|
{
|
|
SDL_DestroyWindow(_window);
|
|
}
|
|
}
|
|
}
|
|
|
|
private GraphicsDebugLevel _glLogLevel;
|
|
private SDL2OpenGLContext _openGLContext;
|
|
|
|
public OpenGLWindow(InputManager inputManager, GraphicsDebugLevel glLogLevel, AspectRatio aspectRatio, bool enableMouse) : base(inputManager, glLogLevel, aspectRatio, enableMouse)
|
|
{
|
|
_glLogLevel = glLogLevel;
|
|
}
|
|
|
|
protected override string GetGpuVendorName()
|
|
{
|
|
return ((Renderer)Renderer).GpuVendor;
|
|
}
|
|
|
|
public override SDL_WindowFlags GetWindowFlags() => SDL_WindowFlags.SDL_WINDOW_OPENGL;
|
|
|
|
protected override void InitializeRenderer()
|
|
{
|
|
// Ensure to not share this context with other contexts before this point.
|
|
SetupOpenGLAttributes(false, _glLogLevel);
|
|
IntPtr context = SDL_GL_CreateContext(WindowHandle);
|
|
SDL_GL_SetSwapInterval(1);
|
|
|
|
if (context == IntPtr.Zero)
|
|
{
|
|
string errorMessage = $"SDL_GL_CreateContext failed with error \"{SDL_GetError()}\"";
|
|
|
|
Logger.Error?.Print(LogClass.Application, errorMessage);
|
|
|
|
throw new Exception(errorMessage);
|
|
}
|
|
|
|
// NOTE: The window handle needs to be disposed by the thread that created it and is handled separately.
|
|
_openGLContext = new SDL2OpenGLContext(context, WindowHandle, false);
|
|
|
|
// First take exclusivity on the OpenGL context.
|
|
((Renderer)Renderer).InitializeBackgroundContext(SDL2OpenGLContext.CreateBackgroundContext(_openGLContext));
|
|
|
|
_openGLContext.MakeCurrent();
|
|
|
|
GL.ClearColor(0, 0, 0, 1.0f);
|
|
GL.Clear(ClearBufferMask.ColorBufferBit);
|
|
SwapBuffers(0);
|
|
|
|
Renderer?.Window.SetSize(DefaultWidth, DefaultHeight);
|
|
MouseDriver.SetClientSize(DefaultWidth, DefaultHeight);
|
|
}
|
|
|
|
protected override void FinalizeRenderer()
|
|
{
|
|
// Try to bind the OpenGL context before calling the gpu disposal.
|
|
_openGLContext.MakeCurrent();
|
|
|
|
Device.DisposeGpu();
|
|
|
|
// Unbind context and destroy everything
|
|
SDL_GL_MakeCurrent(WindowHandle, IntPtr.Zero);
|
|
_openGLContext.Dispose();
|
|
}
|
|
|
|
protected override void SwapBuffers(object image)
|
|
{
|
|
if ((int)image != 0)
|
|
{
|
|
// The game's framebruffer is already bound, so blit it to the window's backbuffer
|
|
GL.BindFramebuffer(FramebufferTarget.DrawFramebuffer, 0);
|
|
|
|
GL.Clear(ClearBufferMask.ColorBufferBit);
|
|
GL.ClearColor(0, 0, 0, 1);
|
|
|
|
GL.BlitFramebuffer(0,
|
|
0,
|
|
Width,
|
|
Height,
|
|
0,
|
|
0,
|
|
Width,
|
|
Height,
|
|
ClearBufferMask.ColorBufferBit,
|
|
BlitFramebufferFilter.Linear);
|
|
}
|
|
|
|
SDL_GL_SwapWindow(WindowHandle);
|
|
}
|
|
}
|
|
}
|