mirror of
https://github.com/Ryujinx/Ryujinx.git
synced 2024-09-19 17:49:46 +02:00
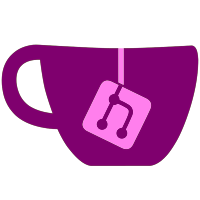
* Implement some IPC related kernel SVCs properly * Fix BLZ decompression when the segment also has a uncompressed chunck * Set default cpu core on process start from ProgramLoader, remove debug message * Load process capabilities properly on KIPs * Fix a copy/paste error in UnmapPhysicalMemory64 * Implement smarter switching between old and new IPC system to support the old HLE services implementation without the manual switch * Implement RegisterService on sm and AcceptSession (partial) * Misc fixes and improvements on new IPC methods * Move IPC related SVCs into a separate file, and logging on RegisterService (sm) * Some small fixes related to receive list buffers and error cases * Load NSOs using the correct pool partition * Fix corner case on GetMaskFromMinMax where range is 64, doesn't happen in pratice however * Fix send static buffer copy * Session release, implement closing requests on client disconnect * Implement ConnectToPort SVC * KLightSession init
97 lines
3.1 KiB
C#
97 lines
3.1 KiB
C#
using Ryujinx.Common.Logging;
|
|
using Ryujinx.HLE.HOS.Ipc;
|
|
using Ryujinx.HLE.HOS.Kernel.Common;
|
|
using Ryujinx.HLE.HOS.Kernel.Threading;
|
|
using System.Collections.Generic;
|
|
|
|
namespace Ryujinx.HLE.HOS.Services.Psm
|
|
{
|
|
class IPsmSession : IpcService
|
|
{
|
|
private Dictionary<int, ServiceProcessRequest> _commands;
|
|
|
|
public override IReadOnlyDictionary<int, ServiceProcessRequest> Commands => _commands;
|
|
|
|
private KEvent _stateChangeEvent;
|
|
private int _stateChangeEventHandle;
|
|
|
|
public IPsmSession(Horizon system)
|
|
{
|
|
_commands = new Dictionary<int, ServiceProcessRequest>
|
|
{
|
|
{ 0, BindStateChangeEvent },
|
|
{ 1, UnbindStateChangeEvent },
|
|
{ 2, SetChargerTypeChangeEventEnabled },
|
|
{ 3, SetPowerSupplyChangeEventEnabled },
|
|
{ 4, SetBatteryVoltageStateChangeEventEnabled }
|
|
};
|
|
|
|
_stateChangeEvent = new KEvent(system);
|
|
_stateChangeEventHandle = -1;
|
|
}
|
|
|
|
// BindStateChangeEvent() -> KObject
|
|
public long BindStateChangeEvent(ServiceCtx context)
|
|
{
|
|
if (_stateChangeEventHandle == -1)
|
|
{
|
|
KernelResult resultCode = context.Process.HandleTable.GenerateHandle(_stateChangeEvent.ReadableEvent, out int stateChangeEventHandle);
|
|
|
|
if (resultCode != KernelResult.Success)
|
|
{
|
|
return (long)resultCode;
|
|
}
|
|
}
|
|
|
|
context.Response.HandleDesc = IpcHandleDesc.MakeCopy(_stateChangeEventHandle);
|
|
|
|
Logger.PrintStub(LogClass.ServicePsm);
|
|
|
|
return 0;
|
|
}
|
|
|
|
// UnbindStateChangeEvent()
|
|
public long UnbindStateChangeEvent(ServiceCtx context)
|
|
{
|
|
if (_stateChangeEventHandle != -1)
|
|
{
|
|
context.Process.HandleTable.CloseHandle(_stateChangeEventHandle);
|
|
_stateChangeEventHandle = -1;
|
|
}
|
|
|
|
Logger.PrintStub(LogClass.ServicePsm);
|
|
|
|
return 0;
|
|
}
|
|
|
|
// SetChargerTypeChangeEventEnabled(u8)
|
|
public long SetChargerTypeChangeEventEnabled(ServiceCtx context)
|
|
{
|
|
bool chargerTypeChangeEventEnabled = context.RequestData.ReadBoolean();
|
|
|
|
Logger.PrintStub(LogClass.ServicePsm, new { chargerTypeChangeEventEnabled });
|
|
|
|
return 0;
|
|
}
|
|
|
|
// SetPowerSupplyChangeEventEnabled(u8)
|
|
public long SetPowerSupplyChangeEventEnabled(ServiceCtx context)
|
|
{
|
|
bool powerSupplyChangeEventEnabled = context.RequestData.ReadBoolean();
|
|
|
|
Logger.PrintStub(LogClass.ServicePsm, new { powerSupplyChangeEventEnabled });
|
|
|
|
return 0;
|
|
}
|
|
|
|
// SetBatteryVoltageStateChangeEventEnabled(u8)
|
|
public long SetBatteryVoltageStateChangeEventEnabled(ServiceCtx context)
|
|
{
|
|
bool batteryVoltageStateChangeEventEnabled = context.RequestData.ReadBoolean();
|
|
|
|
Logger.PrintStub(LogClass.ServicePsm, new { batteryVoltageStateChangeEventEnabled });
|
|
|
|
return 0;
|
|
}
|
|
}
|
|
} |